What is graphql-scalars?
The graphql-scalars npm package provides a collection of custom GraphQL scalar types for common use cases not covered by the default GraphQL specification. These scalars help in validating and parsing data types such as DateTime, Email, URL, and more, making it easier to handle various data formats in a GraphQL API.
What are graphql-scalars's main functionalities?
DateTime Scalar
The DateTime scalar type is used to handle ISO 8601 date and time strings. This is useful for ensuring that date and time values are properly formatted and validated.
const { DateTimeResolver } = require('graphql-scalars');
const typeDefs = gql`
scalar DateTime
type Event {
id: ID!
name: String!
date: DateTime!
}
`;
const resolvers = {
DateTime: DateTimeResolver,
};
Email Scalar
The Email scalar type is used to validate email addresses. This ensures that any email address provided in the GraphQL API is in a valid format.
const { EmailAddressResolver } = require('graphql-scalars');
const typeDefs = gql`
scalar EmailAddress
type User {
id: ID!
email: EmailAddress!
}
`;
const resolvers = {
EmailAddress: EmailAddressResolver,
};
URL Scalar
The URL scalar type is used to validate URLs. This ensures that any URL provided in the GraphQL API is in a valid format.
const { URLResolver } = require('graphql-scalars');
const typeDefs = gql`
scalar URL
type Website {
id: ID!
url: URL!
}
`;
const resolvers = {
URL: URLResolver,
};
JSON Scalar
The JSON scalar type is used to handle arbitrary JSON objects. This is useful for fields that need to store complex data structures.
const { JSONResolver } = require('graphql-scalars');
const typeDefs = gql`
scalar JSON
type Config {
id: ID!
settings: JSON!
}
`;
const resolvers = {
JSON: JSONResolver,
};
Other packages similar to graphql-scalars
graphql-type-json
The graphql-type-json package provides a JSON scalar type for GraphQL. It allows you to use JSON objects as scalar values in your GraphQL schema. Compared to graphql-scalars, it focuses solely on JSON handling and does not provide other custom scalar types.
graphql-iso-date
The graphql-iso-date package provides ISO 8601 compliant date/time scalar types for GraphQL. It includes Date, Time, and DateTime scalars. Compared to graphql-scalars, it is more specialized in handling date and time formats but does not offer other scalar types like Email or URL.
graphql-custom-types
The graphql-custom-types package offers a set of custom scalar types for GraphQL, including DateTime, Email, and URL. It is similar to graphql-scalars in terms of the types it provides, but it may have different implementations and additional features.
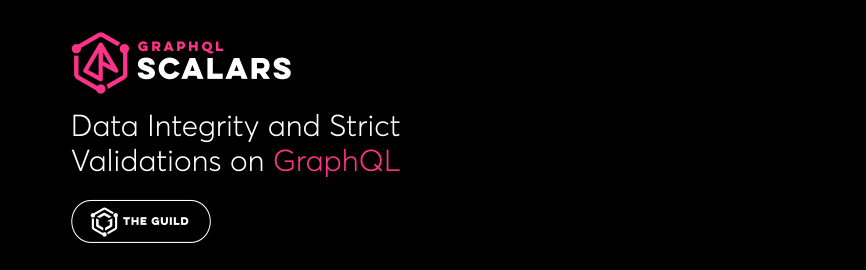

A library of custom GraphQL scalar types for creating precise type-safe GraphQL schemas.
Getting Started
Please refer to our website for all the documentation related to GraphQL Scalars
Contributions
Contributions, issues and feature requests are very welcome. If you are using this package and fixed a bug for yourself, please consider submitting a PR!
And if this is your first time contributing to this project, please do read our Contributor Workflow Guide before you get started off.
Code of Conduct
Help us keep GraphQL Scalars open and inclusive. Please read and follow our Code of Conduct as adopted from Contributor Covenant
License
Released under the MIT license.
Thanks
This library was originally published as @okgrow/graphql-scalars
.
It was created and maintained by the company ok-grow
.
We, The Guild, took over the maintenance of that library later on.
We also like to say thank you to @adriano-di-giovanni for being extremely generous and giving us the graphql-scalars
name on npm which was previously owned by his own library.
And thanks to excitement-engineer for graphql-iso-date, stems for graphql-bigint, taion for graphql-type-json, langpavel for GraphQLTimestamp.js, vespertilian for Duration scalar, maxwellsmart84 for NonEmptyString
scalar