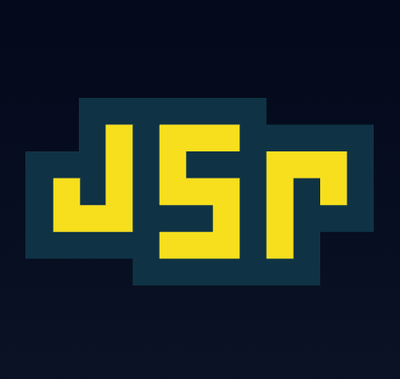
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
NOTE: This is the README for grunt-s3
v0.2.0-alpha
. For v0.1.0
, go here.
Amazon S3 is a great tool for storing/serving data. Thus, there is a chance it is part of your build process. This task can help you automate uploading/downloading files to/from Amazon S3. All file transfers are verified and will produce errors if incomplete.
npm install grunt-s3 --save-dev
Then add this line to your project's Gruntfile.js
:
grunt.loadNpmTasks('grunt-s3');
The grunt-s3 task is now a multi-task; meaning you can specify different targets for this task to run as.
A quick reference of options
{ 'X-Awesomeness': 'Out-Of-This-World', 'X-Stuff': 'And Things!' }
private
, public-read
, public-read-write
, authenticated-read
, bucket-owner-read
, bucket-owner-full-control
['.jpg', '.jpeg', '.png']
.src
and a dest
. Any of the above values may also be overriden. Passing rel:DIR
will cause the filesnames to be
expanded so that wild cards are not passed to the source name.src
and a dest
. Any of the above values may also be overriden.src
to delete from s3. Any of
the above values may also be overriden.src
and dest
. Default behavior is to
only upload new files (that don't exist). Adding verify:true
forces an MD5 hash and Modified time check prior
to overwriting the server files.Template strings in grunt will allow you to easily include values from other files. The below example demonstrates loading aws settings from another file, Where grunt-aws.json is just a json key:value file like package.json. (Special thanks to @nanek)
This is important because you should never check in your S3 credentials to github! Load them from an external file that is outside of the repo.
grunt.initConfig({
aws: grunt.file.readJSON('~/grunt-aws.json'),
s3: {
options: {
key: '<%= aws.key %>',
secret: '<%= aws.secret %>',
bucket: '<%= aws.bucket %>',
access: 'public-read',
headers: {
// Two Year cache policy (1000 * 60 * 60 * 24 * 730)
"Cache-Control": "max-age=630720000, public",
"Expires": new Date(Date.now() + 63072000000).toUTCString()
}
},
dev: {
// These options override the defaults
options: {
encodePaths: true,
maxOperations: 20
},
// Files to be uploaded.
upload: [
{
src: 'important_document.txt',
dest: 'documents/important.txt',
options: { gzip: true }
},
{
src: 'passwords.txt',
dest: 'documents/ignore.txt',
// These values will override the above settings.
bucket: 'some-specific-bucket',
access: 'authenticated-read'
},
{
// Wildcards are valid *for uploads only* until I figure out a good implementation
// for downloads.
src: 'documents/*.txt',
// But if you use wildcards, make sure your destination is a directory.
dest: 'documents/'
}
],
// Files to be downloaded.
download: [
{
src: 'documents/important.txt',
dest: 'important_document_download.txt'
},
{
src: 'garbage/IGNORE.txt',
dest: 'passwords_download.txt'
}
],
del: [
{
src: 'documents/launch_codes.txt'
},
{
src: 'documents/backup_plan.txt'
}
],
sync: [
{
// only upload this document if it does not exist already
src: 'important_document.txt',
dest: 'documents/important.txt',
options: { gzip: true }
},
{
// make sure this document is newer than the one on S3 and replace it
verify: true,
src: 'passwords.txt',
dest: 'documents/ignore.txt'
},
{
src: path.join(variable.to.release, "build/cdn/js/**/*.js"),
dest: "jsgz",
// make sure the wildcard paths are fully expanded in the dest
rel: path.join(variable.to.release, "build/cdn/js"),
options: { gzip: true }
}
]
}
}
});
Running grunt s3
using the above config produces the following output:
$ grunt s3
Running "s3" task
>> ↙ Downloaded: documents/important.txt (e704f1f4bec2d17f09a0e08fecc6cada)
>> ↙ Downloaded: garbage/IGNORE.txt (04f7cb4c893b2700e4fa8787769508e8)
>> ↗ Uploaded: documents/document1.txt (04f7cb4c893b2700e4fa8787769508e8)
>> ↗ Uploaded: passwords.txt (04f7cb4c893b2700e4fa8787769508e8)
>> ↗ Uploaded: important_document.txt (e704f1f4bec2d17f09a0e08fecc6cada)
>> ↗ Uploaded: documents/document2.txt (04f7cb4c893b2700e4fa8787769508e8)
>> ✗ Deleted: documents/launch_codes.txt
>> ✗ Deleted: documents/backup_plan.txt
Done, without errors.
If you do not pass in a key and secret with your config, grunt-s3
will fallback to the following
environment variables:
AWS_ACCESS_KEY_ID
AWS_SECRET_ACCESS_KEY
Helpers have been removed from Grunt 0.4 to access these methods directly. You can now require the s3 library files directly like so:
var s3 = require('grunt-s3').helpers;
Make sure you explicitly pass the options into the method. If you've used grunt.initConfig()
you can use grunt.config.get('s3')
to access them.
Upload a file to s3. Returns a Promises/J-style Deferred object.
src (required) - The path to the file to be uploaded. Accepts wildcards, i.e. files/*.txt
dest (required) - The path on s3 where the file will be uploaded, relative to the bucket. If you use a wildcard for src, this should be a directory.
options (optional) - An object containing any of the following values. These values override any values specified in the main config.
private
, public-read
, public-read-write
,
authenticated-read
, bucket-owner-read
, bucket-owner-full-control
Download a file from s3. Returns a Promises/J-style Deferred object.
src (required) - The path on S3 from which the file will be downloaded, relative to the bucket. Does not accept wildcards
dest (required) - The local path where the file will be saved.
options (optional) - An object containing any of the following values. These values override any values specified in the main config.
Delete a file from s3. Returns a Promises/J-style Deferred object.
src (required) - The path on S3 of the file to delete, relative to the bucket. Does not accept wildcards
options (optional) - An object containing any of the following values. These values override any values specified in the main config.
var upload = s3.upload('dist/my-app-1.0.0.tar.gz', 'archive/my-app-1.0.0.tar.gz');
upload
.done(function(msg) {
console.log(msg);
})
.fail(function(err) {
console.log(err);
})
.always(function() {
console.log('dance!');
});
var download = s3.download('dist/my-app-0.9.9.tar.gz', 'local/my-app-0.9.9.tar.gz');
download.done(function() {
s3.delete('dist/my-app-0.9.9.tar.gz');
});
grunt
version 0.4.x
.knox
to 0.4.1
.underscore.deferred
to 0.1.4
. Version 0.1.3
would fail to install sometimes
due to there being two versions of the module with different capitalizations in npm.FAQs
A grunt task to automate moving files to/from Amazon S3.
The npm package grunt-s3 receives a total of 117 weekly downloads. As such, grunt-s3 popularity was classified as not popular.
We found that grunt-s3 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.