GuideLoop
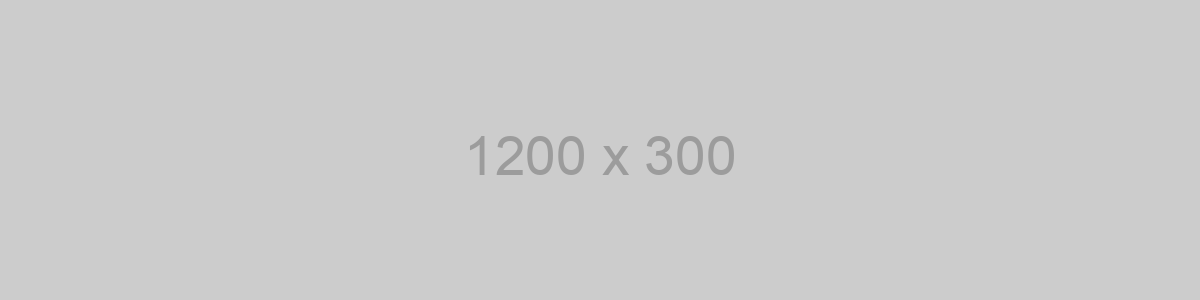
A modern, flexible, and powerful guided tour library for React applications. GuideLoop helps you create engaging product tours, feature introductions, and onboarding experiences with minimal effort.

✨ Features
- 🎨 Multiple pre-built themes (Tailwind, Material-UI, Ant Design)
- 🎯 Smart positioning with automatic repositioning
- 🔄 Smooth transitions between steps
- ⌨️ Full keyboard navigation support
- 📱 Responsive and mobile-friendly
- 🎛️ Highly customizable
- 🌗 Dark mode support
- 🎭 Custom rendering options
- 🔍 Scroll handling & element visibility detection
- 🎬 Custom animations
- ♿ ARIA-compliant accessibility
📦 Installation
npm install guideloop
yarn add guideloop
pnpm add guideloop
🚀 Quick Start
import { GuideLoop } from 'guideloop';
import { useState } from 'react';
function App() {
const [isGuideOpen, setIsGuideOpen] = useState(false);
const steps = [
{
target: '#welcome-button',
title: 'Welcome to Our App!',
content: 'Start your journey here.',
placement: 'bottom'
},
{
target: '#feature-section',
title: 'Key Features',
content: 'Discover what you can do.',
placement: 'right'
}
];
return (
<>
<button onClick={() => setIsGuideOpen(true)}>
Start Tour
</button>
<GuideLoop
steps={steps}
isOpen={isGuideOpen}
onClose={() => setIsGuideOpen(false)}
theme="tailwind"
/>
</>
);
}
🎨 Themes
GuideLoop comes with several built-in themes:
<GuideLoop theme="tailwind" />
<GuideLoop theme="material" />
<GuideLoop theme="antd" />
<GuideLoop
theme="custom"
customTheme={{
tooltip: {
background: '#2D3748',
textColor: '#FFFFFF',
borderRadius: '12px',
},
overlay: {
color: 'rgba(0, 0, 0, 0.75)'
}
}}
/>
🛠️ API Reference
GuideLoop Props
Prop | Type | Default | Description |
---|
steps | Step[] | Required | Array of step configurations |
isOpen | boolean | Required | Controls guide visibility |
onClose | () => void | Required | Handler for closing the guide |
theme | 'tailwind' | 'material' | 'antd' | 'custom' | 'tailwind' | Visual theme |
customTheme | CustomTheme | undefined | Custom theme configuration |
initialStep | number | 0 | Starting step index |
overlay | boolean | true | Show/hide overlay |
keyboard | boolean | true | Enable keyboard navigation |
scrollSmooth | boolean | true | Enable smooth scrolling |
spotlightPadding | number | 8 | Padding around highlighted elements |
Step Configuration
interface Step {
target: string;
title: string;
content: string | ReactNode;
placement?: Placement;
spotlightPadding?: number;
beforeStep?: () => Promise<void> | void;
afterStep?: () => Promise<void> | void;
buttons?: {
next?: ReactNode;
prev?: ReactNode;
close?: ReactNode;
};
}
🎮 Advanced Usage
Custom Animations
<GuideLoop
animations={{
tooltip: {
enter: 'fadeIn',
exit: 'fadeOut'
},
spotlight: {
enter: 'zoomIn',
exit: 'zoomOut'
}
}}
/>
Event Hooks
<GuideLoop
onStepChange={(step) => {
analytics.track('tour_step_viewed', { stepIndex: step });
}}
onComplete={() => {
analytics.track('tour_completed');
}}
onSkip={() => {
analytics.track('tour_skipped');
}}
/>
Conditional Steps
const steps = [
{
target: '#step1',
title: 'Step 1',
condition: () => userRole === 'admin'
},
{
target: '#step2',
title: 'Step 2',
condition: () => featureFlags.newFeature
}
];
📚 Examples
Check out our Storybook for live examples and code snippets.
🤝 Contributing
We welcome contributions! Please see our Contributing Guide for details.
📄 License
MIT © [Ozan Kesici]