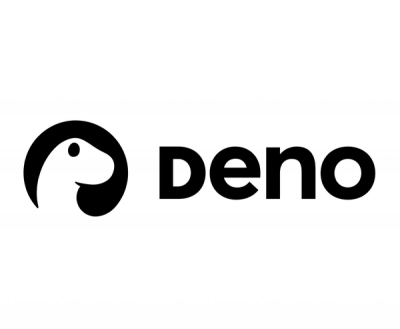
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
hapi-decorators
Advanced tools
Decorators for HapiJS routes. Heavily inspired and borrowed from https://github.com/stewartml/express-decorators
Decorators for HapiJS routes. Heavily inspired and borrowed from https://github.com/stewartml/express-decorators
Great to mix with https://github.com/jayphelps/core-decorators.js
npm install --save hapi-decorators
import {
controller,
get
} from 'hapi-decorators'
@controller('/hello')
public class TestController {
constructor(target) {
this.target = target
}
@get('/world')
sayHello(request, reply) {
reply({ message: `hello, ${this.target}` })
}
}
let test = new TestController('world')
server.routes(test.routes())
@controller(basePath)
REQUIRED This decorator is required at the class level, since it processes the other decorators, and adds
the instance.routes()
function, which returns the routes that can be used with Hapi, e.g. server.routes(users.routes())
.
@route(method, path)
This decorator should be attached to a method of a class, e.g.
@controller('/users')
class Users {
@route('post', '/')
newUser(request, reply) {
reply([])
}
}
Helper Decorators
@get(path)
@post(path)
@put(path)
@patch(path)
@delete(path)
@all(path)
These are shortcuts for @route(method, path)
where @get('/revoke')
would be @route('get', '/revoke')
.
@validate(config)
Add a validation object for the different types, except for the response.
config
is an object, with keys for the different types, e.g. payload
.
@config
- Passing a whole config, solution that would encompase all future decorators in a crude way. Most control to the user.@auth
- Handy auth, without doing the whole config.@cache
FAQs
Decorators for HapiJS routes using ES6 classes
We found that hapi-decorators demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.