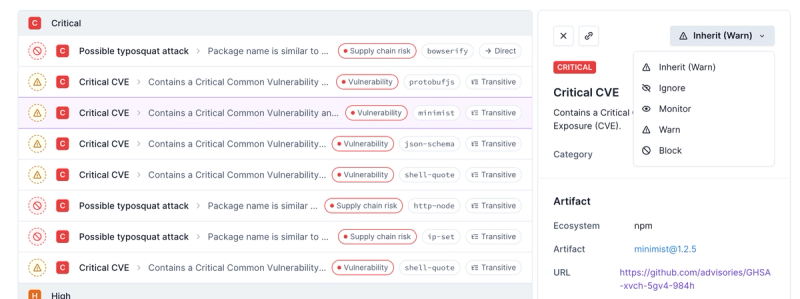
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
hast-util-from-parse5
Advanced tools
Readme
Transform HAST to Parse5’s AST.
npm:
npm install hast-util-from-parse5
Say we have the following file, example.html
:
<!doctype html><title>Hello!</title><h1 id="world">World!<!--after-->
And our script, example.js
, looks as follows:
var vfile = require('to-vfile')
var parse5 = require('parse5')
var inspect = require('unist-util-inspect')
var fromParse5 = require('hast-util-from-parse5')
var doc = vfile.readSync('example.html')
var ast = parse5.parse(String(doc), {sourceCodeLocationInfo: true})
var hast = fromParse5(ast, doc)
console.log(inspect(hast))
Now, running node example
yields:
root[2] (1:1-2:1, 0-70) [data={"quirksMode":false}]
├─ doctype (1:1-1:16, 0-15) [name="html"]
└─ element[2] [tagName="html"]
├─ element[1] [tagName="head"]
│ └─ element[1] (1:16-1:37, 15-36) [tagName="title"]
│ └─ text: "Hello!" (1:23-1:29, 22-28)
└─ element[1] [tagName="body"]
└─ element[3] (1:37-2:1, 36-70) [tagName="h1"][properties={"id":"world"}]
├─ text: "World!" (1:52-1:58, 51-57)
├─ comment: "after" (1:58-1:70, 57-69)
└─ text: "\n" (1:70-2:1, 69-70)
toParse5(ast[, options])
Transform an ASTNode
to a HAST Node.
options
If options
is a VFile, it’s treated as {file: options}
.
options.file
Virtual file, used to add positional information to HAST nodes. If given, the file should have the original HTML source as its contents.
options.verbose
Whether to add positional information about starting tags, closing tags,
and attributes to elements (boolean
, default: false
). Note: not used
without file
.
For the following HTML:
<img src="http://example.com/fav.ico" alt="foo" title="bar">
The verbose info would looks as follows:
{
type: 'element',
tagName: 'img',
properties: {
src: 'http://example.com/fav.ico',
alt: 'foo',
title: 'bar'
},
children: [],
data: {
position: {
opening: {
start: {line: 1, column: 1, offset: 0},
end: {line: 1, column: 61, offset: 60}
},
closing: null,
properties: {
src: {
start: {line: 1, column: 6, offset: 5},
end: {line: 1, column: 38, offset: 37}
},
alt: {
start: {line: 1, column: 39, offset: 38},
end: {line: 1, column: 48, offset: 47}
},
title: {
start: {line: 1, column: 49, offset: 48},
end: {line: 1, column: 60, offset: 59}
}
}
}
},
position: {
start: {line: 1, column: 1, offset: 0},
end: {line: 1, column: 61, offset: 60}
}
}
See contributing.md
in syntax-tree/hast
for ways to get
started.
This organisation has a Code of Conduct. By interacting with this repository, organisation, or community you agree to abide by its terms.
FAQs
hast utility to transform from Parse5’s AST
The npm package hast-util-from-parse5 receives a total of 2,687,010 weekly downloads. As such, hast-util-from-parse5 popularity was classified as popular.
We found that hast-util-from-parse5 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.