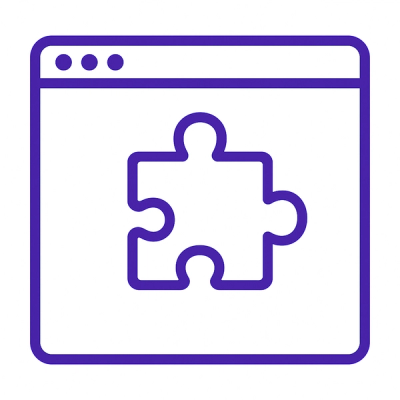
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
header-stack
Advanced tools
A `StreamStack` subclass that parses headers until an emtpy line is found.
StreamStack
subclass that parses headers until an emtpy line is found.This module can be used to parse "headers" from a ReadableStream
until an empty
line is found. "headers" is a somewhat broad term that has a lot of edge-cases,
but this parser can be used to parse HTTP headers, MIME e-mail headers, CGI script
headers, multipart headers, and I'm sure much more.
The parser by default only emits a single 'headers' event when the end of the headers have been reached. It can optionally emit a 'firstLine' event after the first line has been parsed (useful for HTTP, off by default). See below for the other parsing options available.
Creates a new Parser
instance that will parse headers. If a readableStream is passed
in (optional), then 'data' events from it will be used to parse the header. An
optional options argument may also be provided. Recognized options are:
emitFirstLine
- (Default false) - If set to true, then the first line
that gets parsed by the Parser won't be treated like a header line, but
instead will be given back to the user in a 'firstLine' event.
strictCRLF
- (Default false) - If set to true, then ONLY CRLF values
will be allowed for the line delimiter. If false, then both CRLF and
lone LF will be valid delimiters.
strictSpaceAfterColon
- (Default false) - If set to true, an error
will be emitted if a header is found without a space after the delimiter
colon. If false then a space after the colon will be optional.
allowFoldedHeaders
- (Default false) - If set to true then folded headers
will be allowed. Folded headers are headers lines that start with whitespace,
and are intended to be concatenated with the previous header. If false, then
the parse will throw an error if a folded header is encountered.
If no readableStream
instance was passed into the Parser constructor, then you
have to option to manually call parse(buffer)
to do the parsing.
Emitted when the end of the headers has been parsed. headers is a Headers
instance,
which is a special Array subclass with other helper functions. leftover may be
a Buffer with any leftover data the Parser received before finishing, it will be the
beginning of anything after the headers, and should be fed into whatever is parsing
the Stream next.
Emitted if a malformed header line is encountered. i.e. a header line without a valid delimiter. If this is emitted, then a 'headers' event will NOT be emitted.
The Headers
class is an Array subclass that has some additional helper functions
to use and mutate the headers easily.
Adds a new header to the end of the list of headers with the given key and value.
The toString
function of the Headers
class can be used to turn a headers instance
back into it's sendable form. The default options are for the most common use cases,
but you may specify:
firstLine
- (Default false) - If set to a String instance, then the given string
will be used as the first line of the output string (useful for HTTP).
delimiter
- (Default '\r\n') - The delimiter that should be used in between
each header and to signify the end of the headers.
emptyLastLine
- (Default true) - If true then the returned String will contain
another "delimiter" at the end, to signify the end of the headers.
FAQs
A `StreamStack` subclass that parses headers until an emtpy line is found.
The npm package header-stack receives a total of 94 weekly downloads. As such, header-stack popularity was classified as not popular.
We found that header-stack demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.