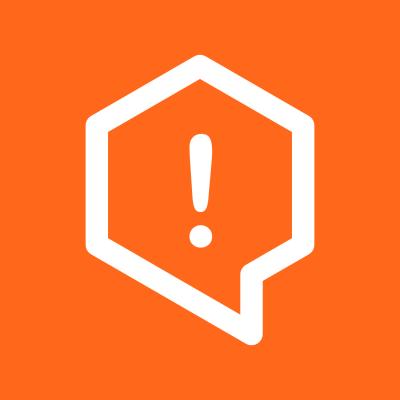
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
hermes-engine
Advanced tools
The hermes-engine npm package provides a JavaScript engine optimized for running React Native applications. It is designed to improve performance and reduce memory usage, making it ideal for mobile applications.
Running JavaScript Code
Hermes-engine allows you to run JavaScript code snippets and get the results. This is useful for executing scripts within a controlled environment.
const { Hermes } = require('hermes-engine');
const hermes = new Hermes();
const result = hermes.evaluate('1 + 1');
console.log(result); // Outputs: 2
Bytecode Compilation
Hermes-engine can compile JavaScript code to bytecode, which can then be executed more efficiently. This is particularly useful for performance-critical applications.
const { compileToBytecode } = require('hermes-engine');
const bytecode = compileToBytecode('function add(a, b) { return a + b; }');
console.log(bytecode);
Memory Management
Hermes-engine provides manual control over garbage collection, allowing developers to manage memory usage more effectively.
const { Hermes } = require('hermes-engine');
const hermes = new Hermes();
hermes.gc();
console.log('Garbage collection completed');
The v8 package provides bindings to the V8 JavaScript engine, which is used in Google Chrome and Node.js. It offers high performance and is widely used in server-side applications. Compared to hermes-engine, V8 is more general-purpose and not specifically optimized for React Native.
QuickJS is a small and embeddable JavaScript engine. It is designed to be lightweight and fast, making it suitable for embedded systems. While it shares some performance goals with hermes-engine, it is not specifically tailored for React Native applications.
Duktape is an embeddable JavaScript engine with a focus on portability and compactness. It is suitable for embedded systems and IoT devices. Unlike hermes-engine, Duktape is not optimized for mobile applications and React Native.
Hermes is a small and lightweight JavaScript VM optimized for running React Native apps on Android.
See hermesengine.dev for more information.
This package contains a Hermes bytecode compiler for Windows, Linux and macOS, plus Android runtime libraries.
Additional tools are available in hermes-engine-cli.
FAQs
A JavaScript engine optimized for running React Native on Android
The npm package hermes-engine receives a total of 110,401 weekly downloads. As such, hermes-engine popularity was classified as popular.
We found that hermes-engine demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.