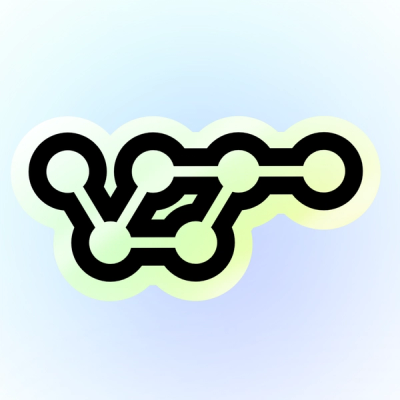
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
heroku-cli-util
Advanced tools
Set of helpful CLI utilities
npm install heroku-cli-util --save
let cli = require('heroku-cli-util');
let promise = heroku.apps(appname).info();
let app = yield cli.action('getting apps', promise);
console.log(`app name: ${app.name}`);
// getting apps... done
// app name: appname
Note: to use yield
you need to wrap this in a co block.
Callback style
let cli = require('heroku-cli-util');
cli.prompt('email', {}, function (_, email) {
console.log(`your email is: ${email}`);
});
Promise style
let cli = require('heroku-cli-util');
cli.prompt('email', {}).then(function (email) {
console.log(`your email is: ${email}`);
});
Generator style (must be wrapped in a co block)
let cli = require('heroku-cli-util');
let email = yield cli.prompt('email', {});
console.log(`your email is: ${email}`);
cli.prompt options
cli.prompt('email', {
mask: true, // mask input field after submitting
hide: true // mask characters while entering
});
Supports the same async styles as prompt()
. Errors if not confirmed.
Basic
let cli = require('heroku-cli-util');
yield cli.confirmApp('appname', context.flags.confirm);
// ! WARNING: Destructive Action
// ! This command will affect the app appname
// ! To proceed, type appname or re-run this command with --confirm appname
> appname
Custom message
let cli = require('heroku-cli-util');
yield cli.confirmApp('appname', context.flags.confirm, 'foo');
// ! foo
// ! To proceed, type appname or re-run this command with --confirm appname
> appname
let cli = require('heroku-cli-util');
cli.error("App not found");
// ! App not found
let cli = require('heroku-cli-util');
cli.warn("App not found");
// ! App not found
let cli = require('heroku-cli-util');
let d = new Date();
console.log(cli.formatDate(d));
// 2001-01-01T08:00:00.000Z
Use hush for verbose logging when HEROKU_DEBUG=1
.
let cli = require('heroku-cli-util');
cli.hush('foo');
// only prints if HEROKU_DEBUG is set
Pretty print an object.
let cli = require('heroku-cli-util');
cli.debug({foo: [1,2,3]});
// { foo: [ 1, 2, 3 ] }
Pretty print a header and hash
let cli = require('heroku-cli-util');
cli.styledHeader("MyApp");
cli.styledHash({name: "myapp", collaborators: ["user1@example.com", "user2@example.com"]});
Produces
=== MyApp
Collaborators: user1@example.com
user1@example.com
Name: myapp
Mock stdout and stderr by using cli.log()
and cli.error()
.
let cli = require('heroku-cli-util');
cli.log('message 1'); // prints 'message 1'
cli.mockConsole();
cli.log('message 2'); // prints nothing
cli.stdout.should.eq('message 2\n');
Used for initializing a plugin command.
give you an auth'ed instance of heroku-client
and cleanly handle API exceptions.
It expects you to return a promise chain. This is usually done with co.
let cli = require('heroku-cli-util');
let co = require('co');
module.exports.commands = [
{
topic: 'apps',
command: 'info',
needsAuth: true,
needsApp: true,
run: cli.command(function (context, heroku) {
return co(function* () {
let app = yield heroku.apps(context.app).info();
console.dir(app);
});
})
}
];
With options:
let cli = require('heroku-cli-util');
let co = require('co');
module.exports.commands = [
{
topic: 'apps',
command: 'info',
needsAuth: true,
needsApp: true,
run: cli.command(
{preauth: true},
function (context, heroku) {
return co(function* () {
let app = yield heroku.apps(context.app).info();
console.dir(app);
});
}
)
}
];
If the command has a two_factor
API error, it will ask the user for a 2fa code and retry.
If you set preauth: true
it will preauth against the current app instead of just setting the header on an app. (This is necessary if you need to do more than 1 API call that will require 2fa)
npm install
npm test
ISC
FAQs
Set of helpful CLI utilities
We found that heroku-cli-util demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.