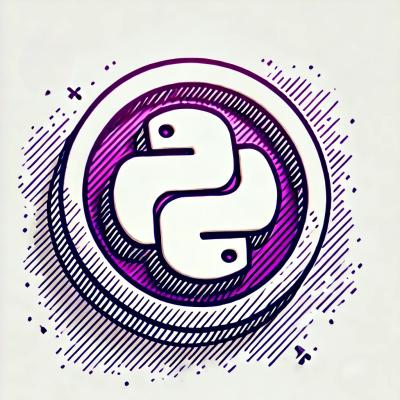
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
The hex2dec npm package provides utilities for converting hexadecimal numbers to decimal and vice versa. It is useful for applications that need to handle numerical data in different bases, particularly in fields like computer science, electronics, and data encoding.
Hexadecimal to Decimal Conversion
This feature allows you to convert a hexadecimal string to its decimal equivalent. In the example, the hexadecimal '1A' is converted to the decimal number 26.
const hex2dec = require('hex2dec');
const decimal = hex2dec.hexToDec('1A');
console.log(decimal); // Output: 26
Decimal to Hexadecimal Conversion
This feature allows you to convert a decimal number to its hexadecimal string equivalent. In the example, the decimal number 26 is converted to the hexadecimal '1A'.
const hex2dec = require('hex2dec');
const hex = hex2dec.decToHex(26);
console.log(hex); // Output: '1A'
The big-integer package provides arbitrary-precision arithmetic for integers, including base conversion functionalities. It is more versatile than hex2dec as it supports a wider range of mathematical operations and can handle very large integers.
The bignumber.js package offers arbitrary-precision decimal and non-decimal arithmetic. It includes methods for base conversion and is suitable for applications requiring high precision and large number handling, making it more comprehensive than hex2dec.
The convert-hex package provides utilities for converting between hexadecimal strings and byte arrays. While it focuses on a different aspect of conversion, it can be used in conjunction with other packages to achieve similar results to hex2dec.
Arbitrary precision decimal↔️hexadecimal converter, from a blog post by Dan Vanderkam.
npm install --save hex2dec
var converter = require('hex2dec');
var dec = converter.hexToDec('0xFA'); // 250
var hex = converter.decToHex('250'); // '0xfa'
var hexString = converter.decToHex('250', { prefix: false }); // 'fa'
(250).toString(16) === 'fa'
and 250 === 0xFA
both work just fine, and will provide enough precision for most uses. For large (>64-bit) numbers, however, precision is lost. This utility provides a higher-precision alternative.
This code may be used under the Apache 2 license.
FAQs
Arbitrary precision decimal/hexadecimal converter.
The npm package hex2dec receives a total of 292,379 weekly downloads. As such, hex2dec popularity was classified as popular.
We found that hex2dec demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.