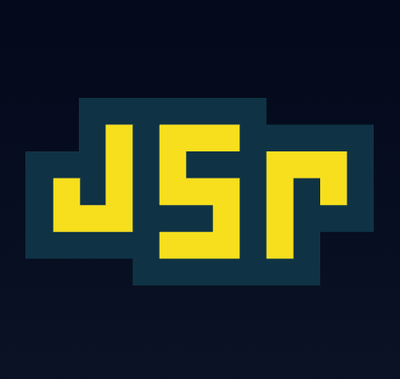
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
hls.js is a JavaScript library that allows you to play HLS (HTTP Live Streaming) streams in browsers that do not support it natively. It works by using Media Source Extensions (MSE) to play HLS streams in browsers like Chrome, Firefox, and Edge.
Basic HLS Playback
This code demonstrates how to set up basic HLS playback using hls.js. It checks if HLS is supported, creates an instance of Hls, loads the HLS stream, attaches it to a video element, and starts playback once the manifest is parsed.
const video = document.getElementById('video');
if (Hls.isSupported()) {
const hls = new Hls();
hls.loadSource('https://path/to/your/hls/playlist.m3u8');
hls.attachMedia(video);
hls.on(Hls.Events.MANIFEST_PARSED, function () {
video.play();
});
}
Error Handling
This code demonstrates how to handle errors in hls.js. It listens for the ERROR event and logs the error type and details. If the error is fatal, it attempts to recover based on the type of error.
hls.on(Hls.Events.ERROR, function (event, data) {
const errorType = data.type;
const errorDetails = data.details;
const errorFatal = data.fatal;
console.error('Error type:', errorType);
console.error('Error details:', errorDetails);
if (errorFatal) {
switch (errorType) {
case Hls.ErrorTypes.NETWORK_ERROR:
console.error('Fatal network error encountered, try to recover');
hls.startLoad();
break;
case Hls.ErrorTypes.MEDIA_ERROR:
console.error('Fatal media error encountered, try to recover');
hls.recoverMediaError();
break;
default:
hls.destroy();
break;
}
}
});
Quality Level Selection
This code demonstrates how to select a specific quality level for playback. It listens for the MANIFEST_PARSED event, logs the available quality levels, and sets the current level to 720p.
hls.on(Hls.Events.MANIFEST_PARSED, function (event, data) {
const availableQualities = hls.levels.map(level => level.height);
console.log('Available qualities:', availableQualities);
hls.currentLevel = availableQualities.indexOf(720); // Set to 720p
});
Video.js is a web video player built from the ground up for an HTML5 world. It supports HLS through plugins like videojs-http-streaming (VHS). Compared to hls.js, Video.js offers a more comprehensive solution for video playback with a wide range of plugins and customization options.
Shaka Player is an open-source JavaScript library for adaptive media. It supports DASH and HLS and is known for its robustness and extensive feature set. Compared to hls.js, Shaka Player offers more advanced features like offline playback and wide support for DRM.
Clappr is an extensible media player for the web. It supports HLS out of the box and is designed to be highly customizable. Compared to hls.js, Clappr provides a more flexible and modular approach to building media players.
Join the discussion via video-dev.org in #hlsjs (our Slack channel)
hls.js is a JavaScript library which implements an HTTP Live Streaming client. It relies on HTML5 video and MediaSource Extensions for playback.
It works by transmuxing MPEG-2 Transport Stream and AAC/MP3 streams into ISO BMFF (MP4) fragments. This transmuxing could be performed asynchronously using Web Worker if available in the browser. hls.js also supports HLS + fmp4, as announced during WWDC2016
hls.js does not need any player, it works directly on top of a standard HTML<video>
element.
hls.js is written in ECMAScript6 (*.js
) and TypeScript (*.ts
) (strongly typed superset of ES6), and transpiled in ECMAScript5 using the TypeScript compiler.
Modules written in TS and plain JS/ES6 can be interdependent and imported/required by each other.
To build our distro bundle and serve our development environment we use Webpack.
Note you can access the docs for a particular version using "https://github.com/video-dev/hls.js/blob/deployments/README.md"
https://hls-js.netlify.com/demo
https://hls-js-latest.netlify.com/demo
Find the commit on https://github.com/video-dev/hls.js/blob/deployments/README.md.
<script src="https://cdn.jsdelivr.net/npm/hls.js@latest"></script>
<!-- Or if you want a more recent canary version -->
<!-- <script src="https://cdn.jsdelivr.net/npm/hls.js@canary"></script> -->
<video id="video"></video>
<script>
var video = document.getElementById('video');
if(Hls.isSupported()) {
var hls = new Hls();
hls.loadSource('https://video-dev.github.io/streams/x36xhzz/x36xhzz.m3u8');
hls.attachMedia(video);
hls.on(Hls.Events.MANIFEST_PARSED,function() {
video.play();
});
}
// hls.js is not supported on platforms that do not have Media Source Extensions (MSE) enabled.
// When the browser has built-in HLS support (check using `canPlayType`), we can provide an HLS manifest (i.e. .m3u8 URL) directly to the video element throught the `src` property.
// This is using the built-in support of the plain video element, without using hls.js.
// Note: it would be more normal to wait on the 'canplay' event below however on Safari (where you are most likely to find built-in HLS support) the video.src URL must be on the user-driven
// white-list before a 'canplay' event will be emitted; the last video event that can be reliably listened-for when the URL is not on the white-list is 'loadedmetadata'.
else if (video.canPlayType('application/vnd.apple.mpegurl')) {
video.src = 'https://video-dev.github.io/streams/x36xhzz/x36xhzz.m3u8';
video.addEventListener('loadedmetadata',function() {
video.play();
});
}
</script>
Video is controlled through HTML <video>
element.
HTMLVideoElement control and events could be used seamlessly.
![]() | ![]() | ![]() | |
---|---|---|---|
![]() | ![]() | ![]() | |
![]() | ![]() | ![]() | ![]() |
![]() | ![]() | ![]() | |
![]() | ![]() | ||
![]() | ![]() | ![]() | ![]() |
![]() | ![]() | ![]() | |
![]() | ![]() | ![]() | |
![]() | ![]() |
hls.js is (being) integrated in the following players:
made by gramk, plays hls from address bar and m3u8 links
No external JS libs are needed. Prepackaged build is included with the releases.
If you want to bundle the application yourself, use node
npm install hls.js
or for the version from master (canary)
npm install hls.js@canary
NOTE: hls.light.*.js
dist files do not include subtitling and alternate-audio features.
Either directly include dist/hls.js or dist/hls.min.js
Or type
npm install --save hls.js
Optionally there is a declaration file available to help with code completion and hinting within your IDE for the hls.js api
npm install --save-dev @types/hls.js
hls.js is compatible with browsers supporting MediaSource extensions (MSE) API with 'video/MP4' mimetypes inputs.
Find a support matrix of the MediaSource API here: https://developer.mozilla.org/en-US/docs/Web/API/MediaSource
As of today, it is supported on:
Please note: iOS Safari "Mobile" does not support the MediaSource API. Safari browsers have however built-in HLS support through the plain video "tag" source URL. See the example above (Getting Started) to run appropriate feature detection and choose between using Hls.js or natively built-in HLS support.
When a platform has neither MediaSource nor native HLS support, you will not be able to play HLS.
require
from a Node.js runtimeWe support this now. You can safely require this library in Node and absolutely nothing will happen :) See https://github.com/video-dev/hls.js/pull/1841
(This is also known as "Universal builds" and "isomorphic apps")
All HLS resources must be delivered with CORS headers permitting GET
requests.
#EXTM3U
#EXTINF
#EXT-X-STREAM-INF
(adaptive streaming)#EXT-X-ENDLIST
(Live playlist)#EXT-X-MEDIA-SEQUENCE
#EXT-X-TARGETDURATION
#EXT-X-DISCONTINUITY
#EXT-X-DISCONTINUITY-SEQUENCE
#EXT-X-BYTERANGE
#EXT-X-MAP
#EXT-X-KEY
(https://tools.ietf.org/html/draft-pantos-http-live-streaming-08#section-3.4.4)#EXT-X-PROGRAM-DATE-TIME
(https://tools.ietf.org/html/draft-pantos-http-live-streaming-18#section-4.3.2.6)EXT-X-START:TIME-OFFSET=x
(https://tools.ietf.org/html/draft-pantos-http-live-streaming-18#section-4.3.5.2)hls.js is released under Apache 2.0 License
Pull requests are welcome. Here is a quick guide on how to start.
git clone https://github.com/video-dev/hls.js.git
# setup dev environment
cd hls.js
npm install
# build dist/hls.js, watch file change for rebuild and launch demo page
npm run dev
# lint
npm run lint
.editorconfig
file.dist/hls.js
file in your PR. We'll take care of generating an updated build right before releasing a new tagged version.After cloning or pulling from the repository, first of all, make sure your local node-modules are up-to-date with the package deps:
npm install
Build all flavors:
npm install
npm run build
Only debug:
npm run build:debug
Build and watch
npm run build:watch
Only specific flavor (known configs are: debug, dist, light, light-dist, demo):
npm run build -- --env.dist # replace "dist" by other configuration name, see above ^
Note: The "demo" config is always built.
Build with bundle analyzer (to help optimize build size)
npm run build:analyze
Run linter:
npm run lint
Run linter with auto-fix mode:
npm run lint:fix
Run linter with errors only (no warnings)
npm run lint:quiet
Run all tests at once:
npm test
Run unit tests:
npm run test:unit
Run unit tests in watch mode:
npm run test:unit:watch
Run functional (integration) tests:
npm run test:func
Click here for details.
FAQs
JavaScript HLS client using MediaSourceExtension
The npm package hls.js receives a total of 552,825 weekly downloads. As such, hls.js popularity was classified as popular.
We found that hls.js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.