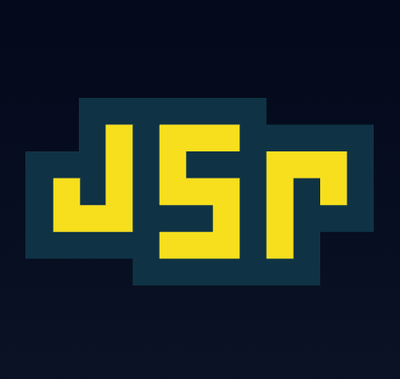
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The hoek package is a utility library that offers a variety of functions for object manipulation, array manipulation, type checking, and encoding. It is designed to provide developers with tools to simplify common tasks in JavaScript programming.
Object cloning
This feature allows for deep cloning of objects, ensuring that nested objects are cloned properly rather than just copying references.
const hoek = require('hoek');
const obj = { a: 1 };
const clone = hoek.clone(obj);
Merge objects
Merge two objects into one, where the second object's properties are added to the first object. This is useful for combining configurations or settings.
const hoek = require('hoek');
const target = { a: 1 };
const source = { b: 2 };
hoek.merge(target, source);
Assert
Provides a simple assertion utility to validate conditions. If the condition is false, it throws an error with the provided message.
const hoek = require('hoek');
hoek.assert(1 === 1, 'This will not throw');
hoek.assert(1 === 2, 'This will throw an error');
Reach
Allows for safely reaching into an object for a nested property. This helps in avoiding errors when accessing deeply nested properties.
const hoek = require('hoek');
const obj = { a: { b: { c: 1 } } };
const value = hoek.reach(obj, 'a.b.c');
Lodash is a comprehensive utility library offering a wide range of functions for tasks including object manipulation, array manipulation, string manipulation, and more. It is more extensive than hoek but can be bulkier due to its size.
Underscore is another utility library similar to lodash but with a smaller footprint. It provides many of the same functionalities as hoek but lacks some of the more specialized functions found in hoek.
Ramda focuses on functional programming, offering utilities that make it easier to apply functional paradigms in JavaScript. It provides similar functionalities for object and array manipulation but from a functional programming perspective, which is different from hoek's more general utility approach.
General purpose node utilities
The Hoek general purpose node utilities library is used to aid in a variety of manners. It comes with useful methods for Arrays (clone, merge, applyToDefaults), Objects (removeKeys, copy), Asserting and more.
For example, to use Hoek to set configuration with default options:
var Hoek = require('hoek');
var default = {url : "www.github.com", port : "8000", debug : true}
var config = Hoek.applyToDefaults(default, {port : "3000", admin : true});
// In this case, config would be { url: 'www.github.com', port: '3000', debug: true, admin: true }
Under each of the sections (such as Array), there are subsections which correspond to Hoek methods. Each subsection will explain how to use the corresponding method. In each js excerpt below, the var Hoek = require('hoek') is omitted for brevity.
Hoek provides several helpful methods for objects and arrays.
This method is used to clone an object or an array. A deep copy is made (duplicates everything, including values that are objects).
var nestedObj = {
w: /^something$/ig,
x: {
a: [1, 2, 3],
b: 123456,
c: new Date()
},
y: 'y',
z: new Date()
};
var copy = Hoek.clone(nestedObj);
copy.x.b = 100;
console.log(copy.y) // results in 'y'
console.log(nestedObj.x.b) // results in 123456
console.log(copy.x.b) // results in 100
isNullOverride, isMergeArrays default to true
Merge all the properties of source into target, source wins in conflic, and by default null and undefined from source are applied
var target = {a: 1, b : 2}
var source = {a: 0, c: 5}
var source2 = {a: null, c: 5}
var targetArray = [1, 2, 3];
var sourceArray = [4, 5];
var newTarget = Hoek.merge(target, source); // results in {a: 0, b: 2, c: 5}
newTarget = Hoek.merge(target, source2); // results in {a: null, b: 2, c: 5}
newTarget = Hoek.merge(target, source2, false); // results in {a: 1, b: 2, c: 5}
newTarget = Hoek.merge(targetArray, sourceArray) // results in [1, 2, 3, 4, 5]
newTarget = Hoek.merge(targetArray, sourceArray, true, false) // results in [4, 5]
Apply options to a copy of the defaults
var defaults = {host: "localhost", port: 8000};
var options = {port: 8080};
var config = Hoek.applyToDefaults(defaults, options); // results in {host: "localhost", port: 8080};
Remove duplicate items from Array
var array = [1, 2, 2, 3, 3, 4, 5, 6];
var newArray = Hoek.unique(array); // results in [1,2,3,4,5,6];
array = [{id: 1}, {id: 1}, {id: 2}];
newArray = Hoek.unique(array, "id") // results in [{id: 1}, {id: 2}]
Convert an Array into an Object
var array = [1,2,3];
var newObject = Hoek.mapToObject(array); // results in [{"1": true}, {"2": true}, {"3": true}]
array = [{id: 1}, {id: 2}];
newObject = Hoek.mapToObject(array, "id") // results in [{"id": 1}, {"id": 2}]
Find the common unique items in two arrays
var array1 = [1, 2, 3];
var array2 = [1, 4, 5];
var newArray = Hoek.intersect(array1, array2) // results in [1]
Find which keys are present
var obj = {a: 1, b: 2, c: 3};
var keys = ["a", "e"];
Hoek.matchKeys(obj, keys) // returns ["a"]
Flatten an array
var array = [1, 2, 3];
var target = [4, 5];
var flattenedArray = Hoek.flatten(array, target) // results in [4, 5, 1, 2, 3];
Remove keys
var object = {a: 1, b: 2, c: 3, d: 4};
var keys = ["a", "b"];
Hoek.removeKeys(object, keys) // object is now {c: 3, d: 4}
Converts an object key chain string to reference
var chain = 'a.b.c';
var obj = {a : {b : { c : 1}}};
Hoek.reach(obj, chain) // returns 1
Inherits a selected set of methods from an object, wrapping functions in asynchronous syntax and catching errors
var targetFunc = function () { };
var proto = {
a: function () {
return 'a!';
},
b: function () {
return 'b!';
},
c: function () {
throw new Error('c!');
}
};
var keys = ['a', 'c'];
Hoek.inheritAsync(targetFunc, proto, ['a', 'c']);
var target = new targetFunc();
target.a(function(err, result){console.log(result)} // returns 'a!'
target.c(function(err, result){console.log(result)} // returns undefined
target.b(function(err, result){console.log(result)} // gives error: Object [object Object] has no method 'b'
Rename a key of an object
var obj = {a : 1, b : 2};
Hoek.rename(obj, "a", "c"); // obj is now {c : 1, b : 2}
A Timer object. Initializing a new timer object sets the ts to the number of milliseconds elapsed since 1 January 1970 00:00:00 UTC.
example :
var timerObj = new Hoek.Timer();
console.log("Time is now: " + timerObj.ts)
console.log("Elapsed time from initialization: " + timerObj.elapsed() + 'milliseconds')
Encodes value in Base64 or URL encoding
Decodes data in Base64 or URL encoding.
Hoek provides convenient methods for escaping html characters. The escaped characters are as followed:
internals.htmlEscaped = {
'&': '&',
'<': '<',
'>': '>',
'"': '"',
"'": ''',
'`': '`'
};
var string = '<html> hey </html>';
var escapedString = Hoek.escapeHtml(string); // returns <html> hey </html>
Escape attribute value for use in HTTP header
var a = Hoek.escapeHeaderAttribute('I said "go w\\o me"'); //returns I said \"go w\\o me\"
Escape string for Regex construction
var a = Hoek.escapeRegex('4^f$s.4*5+-_?%=#!:@|~\\/`"(>)[<]d{}s,'); // returns 4\^f\$s\.4\*5\+\-_\?%\=#\!\:@\|~\\\/`"\(>\)\[<\]d\{\}s\,
var a = 1, b =2;
Hoek.assert(a === b, 'a should equal b'); // ABORT: a should equal b
First checks if process.env.NODE_ENV === 'test', and if so, throws error message. Otherwise, displays most recent stack and then exits process.
Displays the trace stack
var stack = Hoek.displayStack();
console.log(stack) // returns something like:
[ 'null (/Users/user/Desktop/hoek/test.js:4:18)',
'Module._compile (module.js:449:26)',
'Module._extensions..js (module.js:467:10)',
'Module.load (module.js:356:32)',
'Module._load (module.js:312:12)',
'Module.runMain (module.js:492:10)',
'startup.processNextTick.process._tickCallback (node.js:244:9)' ]
Returns a trace stack array.
var stack = Hoek.callStack();
console.log(stack) // returns something like:
[ [ '/Users/user/Desktop/hoek/test.js', 4, 18, null, false ],
[ 'module.js', 449, 26, 'Module._compile', false ],
[ 'module.js', 467, 10, 'Module._extensions..js', false ],
[ 'module.js', 356, 32, 'Module.load', false ],
[ 'module.js', 312, 12, 'Module._load', false ],
[ 'module.js', 492, 10, 'Module.runMain', false ],
[ 'node.js',
244,
9,
'startup.processNextTick.process._tickCallback',
false ] ]
toss(condition /*, [message], callback */)
Return an error as first argument of a callback
Load and parse package.json process root or given directory
var pack = Hoek.loadPackage(); // pack.name === 'hoek'
Loads modules from a given path; option to exclude files (array).
Read an entire stream buffer into a string
readStream(inputStream, function (outputString) {
...
});
FAQs
General purpose node utilities
We found that hoek demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.