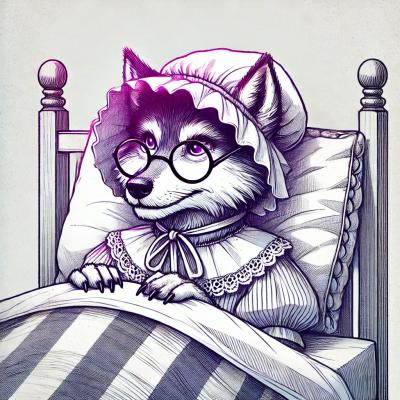
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Hogan.js is a JavaScript templating engine developed by Twitter. It is designed to be a performant and lightweight solution for rendering templates, particularly Mustache templates, on both the client and server sides.
Template Compilation
Hogan.js allows you to compile Mustache templates into JavaScript functions. These functions can then be used to render the templates with given data.
const Hogan = require('hogan.js');
const template = Hogan.compile('Hello, {{name}}!');
const output = template.render({ name: 'World' });
console.log(output); // Output: Hello, World!
Partial Templates
Hogan.js supports partial templates, which allows you to include one template inside another. This is useful for reusing common template fragments.
const Hogan = require('hogan.js');
const mainTemplate = Hogan.compile('Main template: {{>partial}}');
const partialTemplate = Hogan.compile('This is a partial.');
const output = mainTemplate.render({}, { partial: partialTemplate });
console.log(output); // Output: Main template: This is a partial.
Custom Delimiters
Hogan.js allows you to define custom delimiters for your templates. This can be useful if the default Mustache delimiters conflict with other templating languages or syntaxes in your project.
const Hogan = require('hogan.js');
const template = Hogan.compile('Hello, <%name%>!', { delimiters: '<% %>' });
const output = template.render({ name: 'World' });
console.log(output); // Output: Hello, World!
Mustache is a logic-less template syntax. It is a popular templating engine that Hogan.js is based on. Mustache templates can be used in various programming languages, making it a versatile choice. Compared to Hogan.js, Mustache is more widely adopted and has a larger community.
Handlebars.js is an extension of Mustache templates that adds powerful features like helpers and block expressions. It is more feature-rich compared to Hogan.js and is suitable for more complex templating needs. Handlebars.js also has a larger ecosystem of plugins and extensions.
EJS (Embedded JavaScript) is a simple templating language that lets you generate HTML markup with plain JavaScript. It is more flexible than Hogan.js as it allows embedding JavaScript code directly within the templates. EJS is often used in server-side rendering scenarios.
hogan.js is a compiler for the Mustache templating language. For information on Mustache, see the manpage and the spec.
Hogan compiles templates to HoganTemplate objects, which have a render method.
var data = {
screenName: "dhg",
};
var template = Hogan.compile("Follow @{{screenName}}.");
var output = template.render(data);
// prints "Follow @dhg."
console.log(output);
Hogan is fast--try it on your workload.
Hogan has separate scanning, parsing and code generation phases. This way it's possible to add new features without touching the scanner at all, and many different code generation techniques can be tried without changing the parser.
Hogan exposes scan and parse methods. These can be useful for pre-processing templates on the server.
var text = "{{^check}}{{i18n}}No{{/i18n}}{{/check}}";
text += "{{#check}}{{i18n}}Yes{{/i18n}}{{/check}}";
var tree = Hogan.parse(Hogan.scan(text));
// outputs "# check"
console.log(tree[0].tag + " " + tree[0].name);
// outputs "Yes"
console.log(tree[1].nodes[0].nodes[0]);
It's also possible to use HoganTemplate objects without the Hogan compiler present. That means you can pre-compile your templates on the server, and avoid shipping the compiler. However, the optional lambda features from the Mustache spec do require the compiler to be present.
Why another templating library?
Hogan.js was written to meet three templating library requirements: good performance, standalone template objects, and a parser API.
Have a bug? Please create an issue here on GitHub!
https://github.com/twitter/hogan.js/issues
For transparency and insight into our release cycle, releases will be numbered with the follow format:
<major>.<minor>.<patch>
And constructed with the following guidelines:
For more information on semantic versioning, please visit http://semver.org/.
Robert Sayre
Jacob Thornton
Copyright 2011 Twitter, Inc.
Licensed under the Apache License, Version 2.0: http://www.apache.org/licenses/LICENSE-2.0
FAQs
A mustache compiler.
We found that hogan.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.