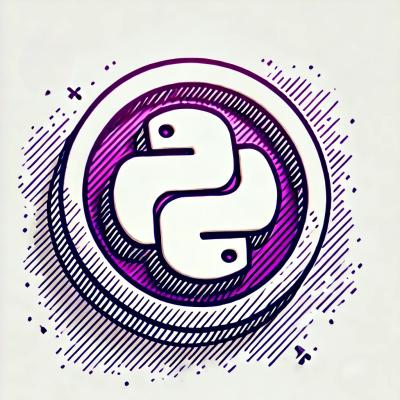
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Hono is a small, simple, and fast web framework for building web applications and APIs in Node.js. It is designed to be lightweight and efficient, making it suitable for high-performance applications.
Basic Routing
Hono allows you to define routes for your web application. In this example, a basic GET route is defined that responds with 'Hello, Hono!' when accessed.
const { Hono } = require('hono');
const app = new Hono();
app.get('/', (c) => c.text('Hello, Hono!'));
app.listen(3000);
Middleware Support
Hono supports middleware, allowing you to execute code before your route handlers. This example demonstrates a simple logger middleware that logs the request method and URL.
const { Hono } = require('hono');
const app = new Hono();
const logger = (c, next) => {
console.log(`${c.req.method} ${c.req.url}`);
return next();
};
app.use(logger);
app.get('/', (c) => c.text('Hello, Hono!'));
app.listen(3000);
Error Handling
Hono provides a way to handle errors globally. In this example, an error is thrown in the route handler, and the global error handler responds with a 500 status code and a message.
const { Hono } = require('hono');
const app = new Hono();
app.get('/', (c) => {
throw new Error('Something went wrong!');
});
app.onError((err, c) => {
c.status(500);
return c.text('Internal Server Error');
});
app.listen(3000);
Express is a widely-used web framework for Node.js, known for its simplicity and flexibility. It offers a robust set of features for web and mobile applications, including routing, middleware support, and more. Compared to Hono, Express has a larger community and more extensive documentation, but Hono aims to be more lightweight and faster.
Koa is a web framework designed by the team behind Express. It aims to be a smaller, more expressive, and more robust foundation for web applications and APIs. Koa uses async functions to help eliminate callback hell and improve error handling. Compared to Hono, Koa is more modern and has a different approach to middleware, using a stack-like structure.
Fastify is a web framework highly focused on providing the best developer experience with the least overhead and a powerful plugin architecture. It is designed for high performance and low overhead. Compared to Hono, Fastify is more feature-rich and has a more extensive ecosystem, but Hono aims to be simpler and more lightweight.
Hono[炎] - means flame🔥 in Japanese - is small, simple, and ultrafast web framework for Service Worker based serverless applications like Cloudflare Workers and Fastly Compute@Edge.
import { Hono } from 'hono'
const app = new Hono()
app.get('/', (c) => c.text('Hono!!'))
app.fire()
Hono is fastest compared to other routers for Cloudflare Workers.
hono x 779,197 ops/sec ±6.55% (78 runs sampled)
itty-router x 161,813 ops/sec ±3.87% (87 runs sampled)
sunder x 334,096 ops/sec ±1.33% (93 runs sampled)
worktop x 212,661 ops/sec ±4.40% (81 runs sampled)
Fastest is hono
✨ Done in 58.29s.
A demonstration to create an application of Cloudflare Workers with Hono.
Now, the named path parameter has types.
You can install Hono from the npm registry.
$ yarn add hono
or
$ npm install hono
An instance of Hono
has these methods.
// HTTP Methods
app.get('/', (c) => c.text('GET /'))
app.post('/', (c) => c.text('POST /'))
// Wildcard
app.get('/wild/*/card', (c) => {
return c.text('GET /wild/*/card')
})
// Any HTTP methods
app.all('/hello', (c) => c.text('Any Method /hello'))
app.get('/user/:name', (c) => {
const name = c.req.param('name')
...
})
app.get('/post/:date{[0-9]+}/:title{[a-z]+}', (c) => {
const date = c.req.param('date')
const title = c.req.param('title')
...
})
const book = app.route('/book')
book.get('/', (c) => c.text('List Books')) // GET /book
book.get('/:id', (c) => {
// GET /book/:id
const id = c.req.param('id')
return c.text('Get Book: ' + id)
})
book.post('/', (c) => c.text('Create Book')) // POST /book
If strict
is set false, /hello
and/hello/
are treated the same.
const app = new Hono({ strict: false }) // Default is true
app.get('/hello', (c) => c.text('/hello or /hello/'))
app.get('/fetch-url', async (c) => {
const response = await fetch('https://example.com/')
return c.text(`Status is ${response.status}`)
})
import { Hono } from 'hono'
import { poweredBy } from 'hono/powered-by'
import { logger } from 'hono/logger'
import { basicAuth } from 'hono/basicAuth'
const app = new Hono()
app.use('*', poweredBy())
app.use('*', logger())
app.use(
'/auth/*',
basicAuth({
username: 'hono',
password: 'acoolproject',
})
)
Available builtin middleware are listed on src/middleware.
You can write your own middleware.
// Custom logger
app.use('*', async (c, next) => {
console.log(`[${c.req.method}] ${c.req.url}`)
await next()
})
// Add a custom header
app.use('/message/*', async (c, next) => {
await next()
await c.header('x-message', 'This is middleware!')
})
app.get('/message/hello', (c) => c.text('Hello Middleware!'))
app.notFound
for customizing Not Found Response.
app.notFound((c) => {
return c.text('Custom 404 Message', 404)
})
app.onError
handle the error and return the customized Response.
app.onError((err, c) => {
console.error(`${err}`)
return c.text('Custom Error Message', 500)
})
To handle Request and Reponse, you can use Context object.
// Get Request object
app.get('/hello', (c) => {
const userAgent = c.req.headers.get('User-Agent')
...
})
// Shortcut to get a header value
app.get('/shortcut', (c) => {
const userAgent = c.req.header('User-Agent')
...
})
// Query params
app.get('/search', (c) => {
const query = c.req.query('q')
...
})
// Captured params
app.get('/entry/:id', (c) => {
const id = c.req.param('id')
...
})
app.get('/welcome', (c) => {
// Set headers
c.header('X-Message', 'Hello!')
c.header('Content-Type', 'text/plain')
// Set HTTP status code
c.status(201)
// Return the response body
return c.body('Thank you for comming')
})
The Response is the same as below.
new Response('Thank you for comming', {
status: 201,
statusText: 'Created',
headers: {
'X-Message': 'Hello',
'Content-Type': 'text/plain',
'Content-Length': '22',
},
})
Render texts as Content-Type:text/plain
.
app.get('/say', (c) => {
return c.text('Hello!')
})
Render JSON as Content-Type:application/json
.
app.get('/api', (c) => {
return c.json({ message: 'Hello!' })
})
Render HTML as Content-Type:text/html
.
app.get('/', (c) => {
return c.html('<h1>Hello! Hono!</h1>')
})
Return the 404 Not Found
Response.
app.get('/notfound', (c) => {
return c.notFound()
})
Redirect, default status code is 302
.
app.get('/redirect', (c) => c.redirect('/'))
app.get('/redirect-permanently', (c) => c.redirect('/', 301))
// Response object
app.use('/', (c, next) => {
next()
c.res.headers.append('X-Debug', 'Debug message')
})
// FetchEvent object
app.use('*', async (c, next) => {
c.event.waitUntil(
...
)
await next()
})
// Environment object for Cloudflare Workers
app.get('*', async c => {
const counter = c.env.COUNTER
...
})
app.fire()
do this.
addEventListener('fetch', (event) => {
event.respondWith(this.handleEvent(event))
})
app.fetch
for Cloudflare Module Worker syntax.
export default {
fetch(request: Request, env: Env, event: FetchEvent) {
return app.fetch(request, env, event)
},
}
/*
or just do:
export default app
*/
Using wrangler
or miniflare
, you can develop the application locally and publish it with few commands.
Let's write your first code for Cloudflare Workers with Hono.
Install Cloudflare Command Line "Wrangler".
$ npm i @cloudflare/wrangler -g
npm init
Make a npm skeleton directory.
mkdir hono-example
cd hono-example
npm init -y
wrangler init
Init as a wrangler project.
$ wrangler init
npm install hono
Install hono
from the npm registry.
$ npm i hono
Only 4 lines!!
import { Hono } from 'hono'
const app = new Hono()
app.get('/', (c) => c.text('Hello! Hono!'))
app.fire()
Run the development server locally. Then, access http://127.0.0.1:8787/
in your Web browser.
$ wrangler dev
Deploy to Cloudflare. That's all!
$ wrangler publish
You can start making your application of Cloudflare Workers with the starter template. It is a realy minimal using TypeScript, esbuild, and Miniflare.
To generate a project skelton, run this command.
$ wrangler generate my-app https://github.com/yusukebe/hono-minimal
Implementation of the original router TrieRouter
is inspired by goblin. RegExpRouter
is inspired by Router::Boom. API design is inspired by express and koa. itty-router, Sunder, and worktop are the other routers or frameworks for Cloudflare Workers.
Contributions Welcome! You can contribute by the following way.
Let's make Hono together!
Thanks to all contributors!
Yusuke Wada https://github.com/yusukebe
Distributed under the MIT License. See LICENSE for more information.
FAQs
Web framework built on Web Standards
The npm package hono receives a total of 178,687 weekly downloads. As such, hono popularity was classified as popular.
We found that hono demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.