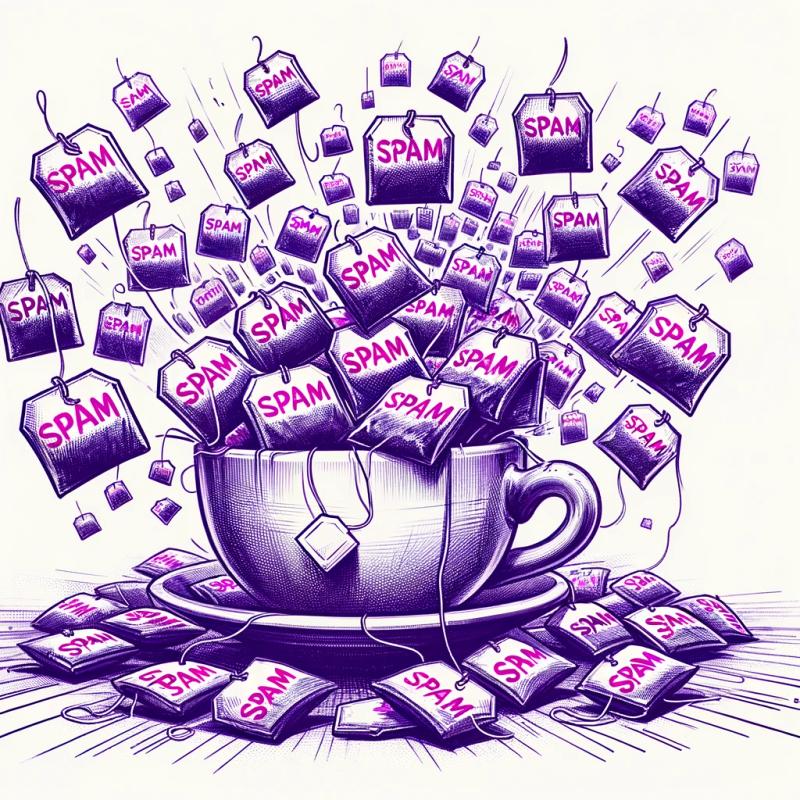
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
honox
Advanced tools
Readme
HonoX is a simple and fast meta-framework for creating full-stack websites or Web APIs - (formerly Sonik). It stands on the shoulders of giants; built on Hono, Vite, and UI libraries.
Note: HonoX is currently in the "alpha stage". Breaking changes are introduced without following semantic versioning.
You can install the honox
package from the npm.
npm install hono honox
If you are starting a new HonoX project, use the hono-create
command. Run the following and choose x-basic
.
npm create hono@latest
Let's create a basic HonoX application using hono/jsx as a renderer. This application has no client JavaScript and renders JSX on the server side.
Below is a typical project structure for a HonoX application.
.
├── app
│ ├── global.d.ts // global type definitions
│ ├── routes
│ │ ├── _404.tsx // not found page
│ │ ├── _error.tsx // error page
│ │ ├── _renderer.tsx // renderer definition
│ │ ├── about
│ │ │ └── [name].tsx // matches `/about/:name`
│ │ └── index.tsx // matches `/`
│ └── server.ts // server entry file
├── package.json
├── tsconfig.json
└── vite.config.ts
vite.config.ts
The minimum Vite setup for development is as follows:
import { defineConfig } from 'vite'
import honox from 'honox/vite'
export default defineConfig({
plugins: [honox()],
})
A server entry file is required. The file should be placed at app/server.ts
. This file is first called by the Vite during the development or build phase.
In the entry file, simply initialize your app using the createApp()
function. app
will be an instance of Hono, so you can use Hono's middleware and the showRoutes()
in hono/dev
.
// app/server.ts
import { createApp } from 'honox/server'
import { showRoutes } from 'hono/dev'
const app = createApp()
showRoutes(app)
export default app
There are three ways to define routes.
createRoute()
Each route should return an array of Handler | MiddlewareHandler
. createRoute()
is a helper function to return it. You can write a route for a GET request with default export
.
// `createRoute()` helps you create handlers
import { createRoute } from 'honox/factory'
export default createRoute((c) => {
return c.render(
<div>
<h1>Hello!</h1>
</div>
)
})
You can also handle methods other than GET by export
POST
, PUT
, and DELETE
.
import { createRoute } from 'honox/factory'
import { getCookie, setCookie } from 'hono/cookie'
export const POST = createRoute(async (c) => {
const { name } = await c.req.parseBody<{ name: string }>()
setCookie(c, 'name', name)
return c.redirect('/')
})
export default createRoute((c) => {
const name = getCookie(c, 'name') ?? 'no name'
return c.render(
<div>
<h1>Hello, {name}!</h1>
<form method='POST'>
<input type='text' name='name' placeholder='name' />
<input type='submit' />
</form>
</div>
)
})
You can create API endpoints by exporting an instance of the Hono object.
// app/routes/about/index.ts
import { Hono } from 'hono'
const app = new Hono()
// matches `/about/:name`
app.get('/:name', (c) => {
const name = c.req.param('name')
return c.json({
'your name is': name,
})
})
export default app
Or simply, you can just return JSX.
export default function Home(_c: Context) {
return <h1>Welcome!</h1>
}
Define your renderer - the middleware that does c.setRender()
- by writing it in _renderer.tsx
.
Before writing _renderer.tsx
, write the Renderer type definition in global.d.ts
.
// app/global.d.ts
import type {} from 'hono'
type Head = {
title?: string
}
declare module 'hono' {
interface ContextRenderer {
(content: string | Promise<string>, head?: Head): Response | Promise<Response>
}
}
The JSX Renderer middleware allows you to create a Renderer as follows:
// app/routes/_renderer.tsx
import { jsxRenderer } from 'hono/jsx-renderer'
export default jsxRenderer(({ children, title }) => {
return (
<html lang='en'>
<head>
<meta charset='UTF-8' />
<meta name='viewport' content='width=device-width, initial-scale=1.0' />
{title ? <title>{title}</title> : <></>}
</head>
<body>{children}</body>
</html>
)
})
The _renderer.tsx
is applied under each directory, and the app/routes/posts/_renderer.tsx
is applied in app/routes/posts/*
.
You can write a custom Not Found page in _404.tsx
.
// app/routes/_404.tsx
import { NotFoundHandler } from 'hono'
const handler: NotFoundHandler = (c) => {
return c.render(<h1>Sorry, Not Found...</h1>)
}
export default handler
You can write a custom Error page in _error.tsx
.
// app/routes/_error.tsx
import { ErrorHandler } from 'hono'
const handler: ErrorHandler = (e, c) => {
return c.render(<h1>Error! {e.message}</h1>)
}
export default handler
Let's create an application that includes a client side. Here, we will use hono/jsx/dom.
Below is the project structure of a minimal application including a client side:
.
├── app
│ ├── client.ts // client entry file
│ ├── global.d.ts
│ ├── islands
│ │ └── counter.tsx // island component
│ ├── routes
│ │ ├── _renderer.tsx
│ │ └── index.tsx
│ └── server.ts
├── package.json
├── tsconfig.json
└── vite.config.ts
This is a _renderer.tsx
, which will load the /app/client.ts
entry file for the client. It will load the JavaScript file for production according to the variable import.meta.env.PROD
. And renders the inside of <HasIslands />
if there are islands on that page.
// app/routes/_renderer.tsx
import { jsxRenderer } from 'hono/jsx-renderer'
import { HasIslands } from 'honox/server'
export default jsxRenderer(({ children }) => {
return (
<html lang='en'>
<head>
<meta charset='UTF-8' />
<meta name='viewport' content='width=device-width, initial-scale=1.0' />
{import.meta.env.PROD ? (
<HasIslands>
<script type='module' src='/static/client.js'></script>
</HasIslands>
) : (
<script type='module' src='/app/client.ts'></script>
)}
</head>
<body>{children}</body>
</html>
)
})
If you have a manifest file in dist/.vite/manifest.json
, you can easily write it using <Script />
.
// app/routes/_renderer.tsx
import { jsxRenderer } from 'hono/jsx-renderer'
import { Script } from 'honox/server'
export default jsxRenderer(({ children }) => {
return (
<html lang='en'>
<head>
<meta charset='UTF-8' />
<meta name='viewport' content='width=device-width, initial-scale=1.0' />
<Script src='/app/client.ts' />
</head>
<body>{children}</body>
</html>
)
})
Note: Since <HasIslands />
can slightly affect build performance when used, it is recommended that you do not use it in the development environment, but only at build time. <Script />
does not cause performance degradation during development, so it's better to use it.
A client side entry file should be in app/client.ts
. Simply, write createClient()
.
// app/client.ts
import { createClient } from 'honox/client'
createClient()
If you want to add interactions to your page, create Island components. Islands components should be:
app/islands
directory or named with _
prefix and island.tsx
suffix like _componentName.island.tsx
.export default function
.For example, you can write an interactive component such as the following counter:
// app/islands/counter.tsx
import { useState } from 'hono/jsx'
export default function Counter() {
const [count, setCount] = useState(0)
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
)
}
When you load the component in a route file, it is rendered as Server-Side rendering and JavaScript is also sent to the client side.
// app/routes/index.tsx
import { createRoute } from 'honox/factory'
import Counter from '../islands/counter'
export default createRoute((c) => {
return c.render(
<div>
<h1>Hello</h1>
<Counter />
</div>
)
})
Note: You cannot access a Context object in Island components. Therefore, you should pass the value from components outside of the Island.
import { useRequestContext } from 'hono/jsx-renderer'
import Counter from '../islands/counter.tsx'
export default function Component() {
const c = useRequestContext()
return <Counter init={parseInt(c.req.query('count') ?? '0', 10)} />
}
You can bring your own renderer using a UI library like React, Preact, Solid, or others.
Note: We may not provide supports for the renderer you bring.
You can define a renderer using @hono/react-renderer
. Install the modules first.
npm i @hono/react-renderer react react-dom hono
npm i -D @types/react @types/react-dom
Define the Props that the renderer will receive in global.d.ts
.
// global.d.ts
import '@hono/react-renderer'
declare module '@hono/react-renderer' {
interface Props {
title?: string
}
}
The following is an example of app/routes/_renderer.tsx
.
// app/routes/_renderer.tsx
import { reactRenderer } from '@hono/react-renderer'
export default reactRenderer(({ children, title }) => {
return (
<html lang='en'>
<head>
<meta charSet='UTF-8' />
<meta name='viewport' content='width=device-width, initial-scale=1.0' />
{import.meta.env.PROD ? (
<script type='module' src='/static/client.js'></script>
) : (
<script type='module' src='/app/client.ts'></script>
)}
{title ? <title>{title}</title> : ''}
</head>
<body>{children}</body>
</html>
)
})
The app/client.ts
will be like this.
// app/client.ts
import { createClient } from 'honox/client'
createClient({
hydrate: async (elem, root) => {
const { hydrateRoot } = await import('react-dom/client')
hydrateRoot(root, elem)
},
createElement: async (type: any, props: any) => {
const { createElement } = await import('react')
return createElement(type, props)
},
})
If you are using the JSX Renderer middleware, you can nest layouts using <Layout />
.
// app/routes/posts/_renderer.tsx
import { jsxRenderer } from 'hono/jsx-renderer'
export default jsxRenderer(({ children, Layout }) => {
return (
<Layout>
<nav>Posts Menu</nav>
<div>{children}</div>
</Layout>
)
})
Props passed to nested renderers do not automatically propagate to the parent renderers. To ensure that the parent layouts receive the necessary props, you should explicitly pass them from the nested component. Here's how you can achieve that:
Let's start with our route handler:
// app/routes/nested/index.tsx
export default createRoute((c) => {
return c.render(<div>Content</div>, { title: 'Dashboard' })
})
Now, let's take a look at our nested renderer:
// app/routes/nested/_renderer.tsx
export default jsxRenderer(({ children, Layout, title }) => {
return (
<Layout title={title}>
{/* Pass the title prop to the parent renderer */}
<main>{children}</main>
</Layout>
)
})
In this setup, all the props sent to the nested renderer's are consumed by the parent renderer:
// app/routes/_renderer.tsx
export default jsxRenderer(({ children, title }) => {
return (
<html lang='en'>
<head>
<title>{title}</title> {/* Use the title prop here */}
</head>
<body>
{children} {/* Insert the Layout's children here */}
</body>
</html>
)
})
You can use Hono's Middleware in each root file with the same syntax as Hono. For example, to validate a value with the Zod Validator, do the following:
import { z } from 'zod'
import { zValidator } from '@hono/zod-validator'
const schema = z.object({
name: z.string().max(10),
})
export const POST = createRoute(zValidator('form', schema), async (c) => {
const { name } = c.req.valid('form')
setCookie(c, 'name', name)
return c.redirect('/')
})
Alternatively, you can use a _middleware.(ts|tsx)
file in a directory to have that middleware applied to the current route, as well as all child routes. Middleware is ran in the order that it is listed within the array.
// /app/routes/_middleware.ts
import { createRoute } from 'honox/factory'
import { logger } from 'hono/logger'
import { secureHeaders } from 'hono/secure-headers'
export default createRoute(logger(), secureHeaders(), ...<more-middleware>)
By default, trailing slashes are removed if the root file is an index file such as index.tsx
or index.mdx
.
However, if you set the trailingSlash
option to true
as the following, the trailing slash is not removed.
import { createApp } from 'honox/server'
const app = createApp({
trailingSlash: true,
})
Like the followings:
trailingSlash
is false
(default): app/routes/path/index.mdx
=> /path
trailingSlash
is true
: app/routes/path/index.mdx
=> /path/
Given that HonoX is Vite-centric, if you wish to utilize Tailwind CSS, simply adhere to the official instructions.
Prepare tailwind.config.js
and postcss.config.js
:
// tailwind.config.js
export default {
content: ['./app/**/*.tsx'],
theme: {
extend: {},
},
plugins: [],
}
// postcss.config.js
export default {
plugins: {
tailwindcss: {},
autoprefixer: {},
},
}
Write app/style.css
:
@tailwind base;
@tailwind components;
@tailwind utilities;
Import it in a renderer file:
// app/routes/_renderer.tsx
import { jsxRenderer } from 'hono/jsx-renderer'
export default jsxRenderer(({ children }) => {
return (
<html lang='en'>
<head>
<meta charset='UTF-8' />
<meta name='viewport' content='width=device-width, initial-scale=1.0' />
{import.meta.env.PROD ? (
<link href='/static/assets/style.css' rel='stylesheet' />
) : (
<link href='/app/style.css' rel='stylesheet' />
)}
</head>
<body>{children}</body>
</html>
)
})
Finally, add vite.config.ts
configuration to output assets for the production.
import honox from 'honox/vite'
import { defineConfig } from 'vite'
export default defineConfig(({ mode }) => {
if (mode === 'client') {
return {
build: {
rollupOptions: {
input: ['/app/style.css'],
output: {
assetFileNames: 'static/assets/[name].[ext]',
},
},
},
}
} else {
return {
plugins: [honox()],
}
}
})
MDX can also be used. Here is the vite.config.ts
.
import devServer from '@hono/vite-dev-server'
import mdx from '@mdx-js/rollup'
import honox from 'honox/vite'
import remarkFrontmatter from 'remark-frontmatter'
import remarkMdxFrontmatter from 'remark-mdx-frontmatter'
import { defineConfig } from 'vite'
const entry = './app/server.ts'
export default defineConfig(() => {
return {
plugins: [
honox(),
devServer({ entry }),
mdx({
jsxImportSource: 'hono/jsx',
remarkPlugins: [remarkFrontmatter, remarkMdxFrontmatter],
}),
],
}
})
Blog site can be created.
// app/routes/index.tsx
import type { Meta } from '../types'
export default function Top() {
const posts = import.meta.glob<{ frontmatter: Meta }>('./posts/*.mdx', {
eager: true,
})
return (
<div>
<h2>Posts</h2>
<ul class='article-list'>
{Object.entries(posts).map(([id, module]) => {
if (module.frontmatter) {
return (
<li>
<a href={`${id.replace(/\.mdx$/, '')}`}>{module.frontmatter.title}</a>
</li>
)
}
})}
</ul>
</div>
)
}
If you want to use Cloudflare's Bindings in your development environment, create wrangler.toml
and configure it properly.
name = "my-project-name"
compatibility_date = "2023-12-01"
pages_build_output_dir = "./dist"
# [vars]
# MY_VARIABLE = "production_value"
# [[kv_namespaces]]
# binding = "MY_KV_NAMESPACE"
# id = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
In vite.config.ts
, use the Cloudflare Adapter in @hono/vite-dev-server
.
import honox from 'honox/vite'
import adapter from '@hono/vite-dev-server/cloudflare'
import { defineConfig } from 'vite'
export default defineConfig({
plugins: [
honox({
devServer: {
adapter,
},
}),
],
})
Since a HonoX instance is essentially a Hono instance, it can be deployed on any platform that Hono supports.
Setup the vite.config.ts
:
// vite.config.ts
import { defineConfig } from 'vite'
import honox from 'honox/vite'
import pages from '@hono/vite-cloudflare-pages'
export default defineConfig({
plugins: [honox(), pages()],
})
If you want to include client side scripts and assets:
// vite.config.ts
import pages from '@hono/vite-cloudflare-pages'
import honox from 'honox/vite'
import client from 'honox/vite/client'
import { defineConfig } from 'vite'
export default defineConfig(({ mode }) => {
if (mode === 'client') {
return {
plugins: [client()],
}
} else {
return {
plugins: [honox(), pages()],
}
}
})
Build command (including a client):
vite build --mode client && vite build
Deploy with the following commands after the build. Ensure you have Wrangler installed:
wrangler pages deploy ./dist
Using Hono's SSG feature, you can generate static HTML for each route.
import { defineConfig } from 'vite'
import honox from 'honox/vite'
import ssg from '@hono/vite-ssg'
const entry = './app/server.ts'
export default defineConfig(() => {
return {
plugins: [honox(), ssg({ entry })],
}
})
If you want to include client side scripts and assets:
// vite.config.ts
import ssg from '@hono/vite-ssg'
import honox from 'honox/vite'
import client from 'honox/vite/client'
import { defineConfig } from 'vite'
const entry = './app/server.ts'
export default defineConfig(({ mode }) => {
if (mode === 'client') {
return {
plugins: [client()],
}
} else {
return {
build: {
emptyOutDir: false,
},
plugins: [honox(), ssg({ entry })],
}
}
})
Build command (including a client):
vite build --mode client && vite build
You can also deploy it to Cloudflare Pages.
wrangler pages deploy ./dist
MIT
FAQs
**HonoX** is a simple and fast meta-framework for creating full-stack websites or Web APIs - (formerly _[Sonik](https://github.com/sonikjs/sonik)_). It stands on the shoulders of giants; built on [Hono](https://hono.dev/), [Vite](https://vitejs.dev/), and
The npm package honox receives a total of 378 weekly downloads. As such, honox popularity was classified as not popular.
We found that honox demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.