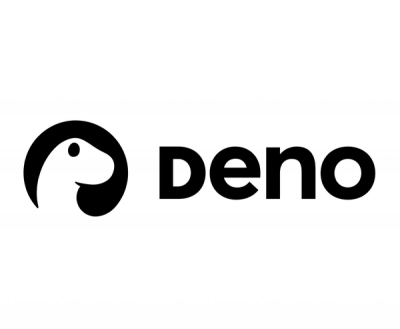
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
__ __ ______ ______ __ ___ __ ______
| | | | / __ \ / __ \ | |/ / | | / __ \
| |__| | | | | | | | | | | ' / | | | | | |
| __ | | | | | | | | | | < | | | | | |
| | | | | `--' | | `--' | | . \ __ | | | `--' |
|__| |__| \______/ \______/ |__|\__\ (__) |__| \______/
a full featured i/o framework for node.js
Core
Web
Utility
Humor
'hook.io` is currently self tested, it would be too hard to not do this. I will take patches to improve testing.
npm install hook.io-helloworld -g
Now run:
hookio-helloworld
Spawn up as many as you want. The first one becomes an output
( server ), the rest will become inputs
( clients ). Each helloworld input will emit a hello on an interval. Now watch the i/o party!
If you want to start logging all these messages simply install hookio-logger
with:
npm install hook.io-logger -g
Now run:
hookio-logger
You will now start logging all these messages.
Both the helloworld and logger hooks act as dual-sided hooks by default. The order you run hookio-logger
and hookio-helloworld
does not matter. They can work as both clients or servers ( inputs or outputs ) and will interface seamlessly using hook.io's default settings.
http://github.com/hookio/helloworld
#!/usr/bin/env node
var Hook = require('hook.io').Hook;
var myhook = new Hook( { name: "helloworld"} );
myhook.start();
myhook.on('i.*', function(source, event, data){
console.log('I am currently getting data on my inputs from: '.green + source.toString().yellow + ' ' + JSON.stringify(data));
});
myhook.on('o.*', function(event, data){
console.log('I am currently sending data to my ouputs on: '.green + event.toString().yellow + ' ' + JSON.stringify(data));
});
myhook.on('ready', function(){
//
// Add some startup commands here
//
console.log('Now that I am ready, I will emit to my outputs on an interval'.yellow);
myhook.emit('o.hello', 'Hello, I am ' + self.name);
// This is just to simulate I/O, don't use timers...
setInterval(function(){
myhook.emit('o.hello', 'Hello, I am ' + self.name);
}, 5000);
});
http://github.com/marak/hook.io-webhook
var Hook = require('hook.io').Hook,
http = require('http');
var webhook = new Hook( { name: 'webhook' });
webhook.listen();
http.createServer(function(req, res){
var body = '';
req.on('data', function(data){
body += data;
});
req.on('end', function(){
webhook.emit('o.request', {
request: {
url: req.url
},
body: body
})
res.end();
});
}).listen(9000);
console.log('http webhook server started on port '.green + '9000'.yellow);
Currently all tests require some setup. Eventually they will be compatible with npat
:
$ cd /path/to/hook.io
$ npm install forever
$ [sudo] npm link
$ cd /path/to/hook.io-helloworld
$ [sudo] npm link
$ [sudo] npm link hook.io
$ cd /path/to/hook.io
$ [sudo] npm link hook.io-helloworld
$ vows --spec
AvianFlu, Chapel, Substack, Dominic Tarr, Charlie Robbins, h1jinx, Tim Smart, tmpvar, kadir pekel
FAQs
Unknown package
The npm package hook.io receives a total of 5 weekly downloads. As such, hook.io popularity was classified as not popular.
We found that hook.io demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.