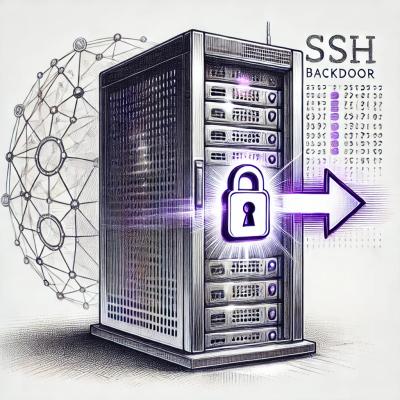
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
This module is for better organizing chain processes with multiple branches. It allows to design process flow by creating branches and setting conditions when they should be called.
Main use case is to create flow template and reuse it with setting concrete functions instead of template ones.
Main unit is a Branch. Each branch accepts condition function and action function. Also each branch can accept other branches.
import { Branch} from "Horpyna";
const branch = new Branch({
name: "branchName"
})
branch.execute(someInput)
.then(response => console.log(response))
or
import { Branch} from "Horpyna";
const branch = Branch.create({
name: "branchName"
})
branch.execute(someInput)
.then(response => console.log(response))
Creates new branch instance.
Creates new branch instance.
{ name: String
condition: Function,
action: Function,
branches: Array<Branch>,
exceptionHandler: Bool
}
import { Branch } from "Horpyna";
const mainBranch = new Branch({
name: "mainBranch",
condition: value => value > 10,
action: value => value + 1,
branches: [{
name: "maxBranch",
condition: value => value >= 15,
action: value => 15
}]
});
mainBranch.execute(10)
.then(console.log)//null
mainBranch.execute(11)
.then(console.log)//12
mainBranch.execute(15)
.then(console.log)//15
Changes branch condition. Returns this branch instance.
import { Branch } from "Horpyna";
const mainBranch = new Branch({
name: "mainBranch",
condition: value => value > 10,
action: value => value + 1,
});
mainBranch.execute(11)
.then(console.log)//12
mainBranch.setCondition(value => value > 11);
mainBranch.execute(11)
.then(console.log)//null
Changes/set branch action. Returns this branch instance.
import { Branch } from "Horpyna";
const mainBranch = new Branch({
name: "mainBranch",
condition: value => value > 10,
action: value => value + 1,
});
mainBranch.execute(11)
.then(console.log)//12
mainBranch.setAction(value => value + 2);
mainBranch.execute(11)
.then(console.log)//13
Adds additional branch to existing one. Returns this branch instance.
import { Branch } from "Horpyna";
const mainBranch = new Branch({
name: "mainBranch",
condition: value => value > 10,
action: value => value + 1,
branches: [{
name: "maxBranch",
condition: value => value >= 15,
action: value => 15
}]
});
mainBranch.execute(10)
.then(console.log)//null
mainBranch.addBranch(new Branch({
name: "minBranch",
condition: value => value < 15,
action: value => value
}));
mainBranch.execute(10)
.then(console.log)//11
Returns first branch by name. If branch doesn't exist it will return null.
import { Branch } from "Horpyna";
const mainBranch = new Branch({
name: "mainBranch",
condition: () => true,
action: () => true,
branches: [{
name: "maxBranch",
condition: () => true,
action: () => true
}]
});
const maxBranch = mainBranch.getBranch("maxBranch");
It will search in all branch tree beginning from current branch. If there is no such a branch it will return null.
import { Branch } from "Horpyna";
const mainBranch = new Branch({
name: "mainBranch",
condition: () => true,
action: () => true,
branches: [{
name: "maxBranch",
condition: () => true,
action: () => true,
branches: [{
name: "someDeepBranch",
condition: () => true,
action: () => true
}]
}]
});
const someDeepBranch = mainBranch.findBranch("someDeepBranch");
Returns branch action function
Returns branch condition function
Returns branch name
Returns all child branches
Add another branch to queue. All branches in queue will be invoked one by one after all child branches are done. Return current branch
import { Branch } from "Horpyna";
const mainBranch = new Branch({
name: "branchA",
condition: () => true,
action: value => value + "A",
branches: [{
name: "branchB",
condition: () => true,
action: value => value + "B",
}]
});
mainBranch.chain({
name: "branchC",
condition: () => true,
action: value => value + "C",
})
mainBranch.chain({
name: "branchD",
condition: () => true,
action: value => value + "D",
})
mainBranch.execute("")
.then(console.log)//"ABCD"
Set new branch name
Shallow copy current branch and return new branch
Returns promise resolvable to calculated output.
import { Branch } from "Horpyna";
const mainBranch = new Branch({
name: "mainBranch",
condition: value => value > 10,
action: value => value + 1,
});
mainBranch.execute(11)
.then(console.log)//12
MIT
FAQs
utility to manage async processes
The npm package horpyna receives a total of 14 weekly downloads. As such, horpyna popularity was classified as not popular.
We found that horpyna demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.