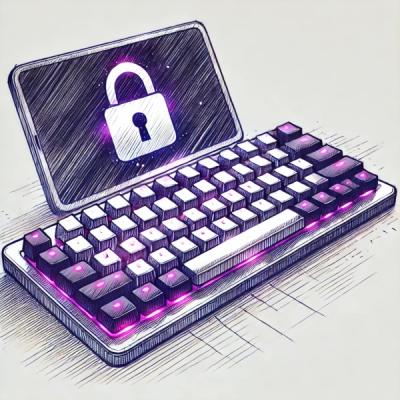
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
hotfile
Advanced tools
Readme
const directory = '/some/directory/path'
const file = '/some/file/path.ext'
const HF = require('hotfile')
const someAsyncFunction = async () => {
const hotfiles = await HF.map(directory)
const hotfile = new HF(file)
const hotdir = hotfiles[0]
const newdir = await hotdir.appendDirectory('subtitles')
}
const hotdir = new HF(directory)
await hotdir.rename('newfilename', 'ext')
const hotfile = new HF(file)
await hotfile.moveTo(hotdir)
const items = await hotfile.map(someFolderPath, {
exclude: /(^|\/)\.[^\/\.]/g,
model: SomeModelThatInheritsFromHotfile
})
Note that all wrapper class objects must inherit from the Hotfile class.
const HF = require('hotfile')
class SomeName extends HF {
constructor(path){
super(path)
// write your code here ...
}
}
const HF = require('hotfile')
class SomeName extends HF {
constructor(path){
super(path)
// write your code here ...
}
filename(name, ext){
if(ext) this.ext = ext
return [
name || this.name,
this.ext && this.ext.replace('.','') || null
].filter(e => e != null).join('.')
}
/* rewriting map function with default settings */
static async map(path, options = {}){
return await super.map(path, {
exclude: /(^|\/)\.[^\/\.]/g,
model: this
})
}
async rename(name, ext){
if(ext) this.ext = ext
const toPath = this.parent + '/' + this.filename(name)
return await this.move(toPath)
}
async moveTo(toPath){
if(toPath instanceof Hotfile && toPath.isDirectory) toPath = toPath.path
toPath = toPath + '/' + this.filename()
return fs.promises.rename(this.path, toPath).then(async () => {
await Object.assign(this, new Hotfile(toPath))
return true
}).catch(() => false)
}
async move(toPath){
return fs.promises.rename(this.path, toPath).then(async () => {
await Object.assign(this, new Hotfile(toPath))
return true
}).catch((err) => {
console.log(err)
return false
})
}
async appendDirectory(name){
if(this.isFile) throw('append only works on directories')
let path = this.path + '/' + name
return fs.promises.mkdir(path, { recursive: true })
.then(() => {
const dir = new Hotfile(path)
this.children.push(dir)
return dir
}).catch(() => false)
}
async delete(){
return fs.promises.unlink(this.path).then(async () => {
await Object.assign(this,{parent: null, path: null, name: null, metadata: null})
return true
}).catch(() => false)
}
}
FAQs
Unknown package
We found that hotfile demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.