Comparing version 1.0.11 to 1.0.12-rc.0
@@ -1,2 +0,2 @@ | ||
import { Apply, Call, Call2, Call3, Call4, Call5, Eval, Fn, Pipe, PipeRight, Compose, ComposeLeft, Constant, Identity, PartialApply, _, arg, arg0, arg1, arg2, arg3, args } from "./internals/core/Core"; | ||
import { Apply, Call, Fn, Pipe, PipeRight, Compose, ComposeLeft, Constant, Identity, PartialApply, _, arg, arg0, arg1, arg2, arg3, args } from "./internals/core/Core"; | ||
import { Functions } from "./internals/functions/Functions"; | ||
@@ -10,2 +10,2 @@ import { Numbers } from "./internals/numbers/Numbers"; | ||
import { Match } from "./internals/match/Match"; | ||
export { _, arg, arg0, arg1, arg2, arg3, args, Fn, Pipe, PipeRight, Call, Call2, Call3, Call4, Call5, Compose, ComposeLeft, Constant, Identity, PartialApply, Apply, Eval, Match, Booleans, Objects, Unions, Strings, Numbers, Tuples, Functions, Booleans as B, Objects as O, Unions as U, Strings as S, Numbers as N, Tuples as T, Functions as F, }; | ||
export { _, arg, arg0, arg1, arg2, arg3, args, Fn, Pipe, PipeRight, Call, Call as $, Apply, Compose, ComposeLeft, Constant, Identity, PartialApply, Match, Booleans, Objects, Unions, Strings, Numbers, Tuples, Functions, Booleans as B, Objects as O, Unions as U, Strings as S, Numbers as N, Tuples as T, Functions as F, }; |
@@ -1,1 +0,18 @@ | ||
export {}; | ||
"use strict"; | ||
var __defProp = Object.defineProperty; | ||
var __getOwnPropDesc = Object.getOwnPropertyDescriptor; | ||
var __getOwnPropNames = Object.getOwnPropertyNames; | ||
var __hasOwnProp = Object.prototype.hasOwnProperty; | ||
var __copyProps = (to, from, except, desc) => { | ||
if (from && typeof from === "object" || typeof from === "function") { | ||
for (let key of __getOwnPropNames(from)) | ||
if (!__hasOwnProp.call(to, key) && key !== except) | ||
__defProp(to, key, { get: () => from[key], enumerable: !(desc = __getOwnPropDesc(from, key)) || desc.enumerable }); | ||
} | ||
return to; | ||
}; | ||
var __toCommonJS = (mod) => __copyProps(__defProp({}, "__esModule", { value: true }), mod); | ||
// src/index.ts | ||
var src_exports = {}; | ||
module.exports = __toCommonJS(src_exports); |
@@ -1,2 +0,2 @@ | ||
import { MergeArgs } from "./impl/MergeArgs"; | ||
import { ExcludePlaceholders, MergeArgs } from "./impl/MergeArgs"; | ||
import { Head } from "../helpers"; | ||
@@ -6,12 +6,21 @@ declare const rawArgs: unique symbol; | ||
/** | ||
* Base type for all functions | ||
* @description You need to extend this type to create a new function that can be used in the HOTScript library. | ||
* usually you will just convert some typescript utility time you already have to a hotscript function. | ||
* This way you can use the HOTScript library to create more complex functions. | ||
* Base interface for all functions. | ||
* | ||
* @description You need to extend this interface to create a function | ||
* that can be composed with other HOTScript functions. | ||
* Usually you will just convert some utility type you already have | ||
* by wrapping it inside a HOTScript function. | ||
* | ||
* Use `this['args']`, `this['arg0']`, `this['arg1']` etc to access | ||
* function arguments. | ||
* | ||
* The `return` property is the value returned by your function. | ||
* | ||
* @example | ||
* ```ts | ||
* export interface CustomOmitFn extends Fn { | ||
* return: this[args] extends [infer obj, infer keys] ? Omit<obj, keys> : never; | ||
* return: Omit<this['arg0'], this['arg1']> | ||
* } | ||
* | ||
* type T = Call<CustomOmitFn, { a, b, c }, 'a'> // { b, c } | ||
* ``` | ||
@@ -28,10 +37,12 @@ */ | ||
} | ||
declare const unset: unique symbol; | ||
declare const _: unique symbol; | ||
/** | ||
* A placeholder type that can be used to indicate that a parameter is not set. | ||
*/ | ||
export type unset = "@hotscript/unset"; | ||
export type unset = typeof unset; | ||
/** | ||
* A placeholder type that can be used to indicate that a parameter is to partially applied. | ||
*/ | ||
export type _ = "@hotscript/placeholder"; | ||
export type _ = typeof _; | ||
export interface arg<Index extends number, Constraint = unknown> extends Fn { | ||
@@ -63,89 +74,24 @@ return: this["args"][Index] extends infer arg extends Constraint ? arg : never; | ||
/** | ||
* Call a HOTScript function with the only one argument. | ||
* Calls a HOTScript function. | ||
* | ||
* @param fn - The function to call. | ||
* @param arg1 - The argument to pass to the function. | ||
* @returns The result of the function. | ||
* @param ...args - optional arguments | ||
* | ||
* @example | ||
* ```ts | ||
* type T0 = Call<Numbers.Negate, 1>; // -1 | ||
* type T0 = Call<Numbers.Add<1, 2>>; // 3 | ||
* type T1 = Call<Numbers.Add<1>, 2>; // 3 | ||
* type T2 = Call<Numbers.Add, 1, 2>; // 3 | ||
* type T3 = Call< | ||
* Tuples.Map<Strings.Split<".">, ["a.b", "b.c"]> | ||
* >; // [["a", "b"], ["b", "c"]] | ||
* ``` | ||
*/ | ||
export type Call<fn extends Fn, arg1> = (fn & { | ||
[rawArgs]: [arg1]; | ||
export type Call<fn extends Fn, arg0 = _, arg1 = _, arg2 = _, arg3 = _> = (fn & { | ||
[rawArgs]: ExcludePlaceholders<[arg0, arg1, arg2, arg3]>; | ||
})["return"]; | ||
/** | ||
* Call a HOTScript function like a normal Utility type. | ||
* | ||
* @param fn - The function to call. | ||
* | ||
* @example | ||
* ```ts | ||
* type T0 = Eval<Numbers.Add<1, 2>>; // 3 | ||
* type T1 = Eval<Numbers.Negate<1>>; // -1 | ||
* ``` | ||
*/ | ||
export type Eval<fn extends Fn> = (fn & { | ||
[rawArgs]: []; | ||
})["return"]; | ||
/** | ||
* Call a HOTScript function with the two arguments. | ||
* | ||
* @param fn - The function to call. | ||
* @param arg1 - The first argument to pass to the function. | ||
* @param arg2 - The second argument to pass to the function. | ||
* @returns The result of the function. | ||
* | ||
* @example | ||
* ```ts | ||
* type T0 = Call2<Numbers.Add, 1, 2>; // 3 | ||
* ``` | ||
*/ | ||
export type Call2<fn extends Fn, arg1, arg2> = (fn & { | ||
[rawArgs]: [arg1, arg2]; | ||
})["return"]; | ||
/** | ||
* Call a HOTScript function with the three arguments. | ||
* | ||
* @param fn - The function to call. | ||
* @param arg1 - The first argument to pass to the function. | ||
* @param arg2 - The second argument to pass to the function. | ||
* @param arg3 - The third argument to pass to the function. | ||
* @returns The result of the function. | ||
*/ | ||
export type Call3<fn extends Fn, arg1, arg2, arg3> = (fn & { | ||
[rawArgs]: [arg1, arg2, arg3]; | ||
})["return"]; | ||
/** | ||
* Call a HOTScript function with the four arguments. | ||
* | ||
* @param fn - The function to call. | ||
* @param arg1 - The first argument to pass to the function. | ||
* @param arg2 - The second argument to pass to the function. | ||
* @param arg3 - The third argument to pass to the function. | ||
* @param arg4 - The fourth argument to pass to the function. | ||
* @returns The result of the function. | ||
*/ | ||
export type Call4<fn extends Fn, arg1, arg2, arg3, arg4> = (fn & { | ||
[rawArgs]: [arg1, arg2, arg3, arg4]; | ||
})["return"]; | ||
/** | ||
* Call a HOTScript function with the five arguments. | ||
* | ||
* @param fn - The function to call. | ||
* @param arg1 - The first argument to pass to the function. | ||
* @param arg2 - The second argument to pass to the function. | ||
* @param arg3 - The third argument to pass to the function. | ||
* @param arg4 - The fourth argument to pass to the function. | ||
* @param arg5 - The fifth argument to pass to the function. | ||
* @returns The result of the function. | ||
*/ | ||
export type Call5<fn extends Fn, arg1, arg2, arg3, arg4, arg5> = (fn & { | ||
args: [arg1, arg2, arg3, arg4, arg5]; | ||
})["return"]; | ||
/** | ||
* Pipe a value through a list of functions. | ||
* @description This is the same as the pipe operator in other languages. | ||
* Evaluates the first function with the initial value, then passes the result to the second function, and so on. | ||
* Calls the first function with the initial value, then passes the result to the second function, and so on. | ||
* | ||
@@ -168,3 +114,3 @@ * @param acc - The initial value to pass to the first function. | ||
* @description This is the same as the pipe operator in other languages. | ||
* Evaluates the last function with the initial value, then passes the result to the second to last function, and so on. | ||
* Calls the last function with the initial value, then passes the result to the second to last function, and so on. | ||
* | ||
@@ -240,5 +186,8 @@ * @param xs - The list of functions to pipe the value through. | ||
/** | ||
* Partially applies the passed arguments to the function and returns a new function. | ||
* The new function will have the applied arguments passed to the original function | ||
* `PartialApply` Pre applies some arguments to a function. | ||
* it takes a `Fn`, and a list of pre applied arguments, | ||
* and returns a new function taking the rest of these arguments. | ||
* | ||
* Most functions in HOTScript are already partially applicable (curried). | ||
* | ||
* @param fn - The function to partially apply. | ||
@@ -250,3 +199,11 @@ * @param partialArgs - The arguments to partially apply. | ||
* ```ts | ||
* type T0 = Call<PartialApply<Parameter, [_, (a: number, b: string) => void]>, 1> ; // [b: string] | ||
* interface Append extends Fn { | ||
* return: [...this['arg1'], this['arg0']] | ||
* } | ||
* | ||
* type Append1 = PartialApply<Append, [1]> | ||
* type T0 = Call<Append1, [0]>; // [0, 1] | ||
* | ||
* type AppendTo123 = PartialApply<Append, [_, [1, 2, 3]]> | ||
* type T1 = Call<AppendTo123, 4>; // [1, 2, 3, 4] | ||
*/ | ||
@@ -253,0 +210,0 @@ export interface PartialApply<fn extends Fn, partialArgs extends unknown[]> extends Fn { |
import { unset, _ } from "../../core/Core"; | ||
import { IsNever } from "../../helpers"; | ||
type ExcludePlaceholders<xs, output extends any[] = []> = xs extends [ | ||
import { Equal, IsNever } from "../../helpers"; | ||
export type ExcludePlaceholders<xs, output extends any[] = []> = xs extends [ | ||
infer first, | ||
...infer rest | ||
] ? first extends _ ? ExcludePlaceholders<rest, output> : ExcludePlaceholders<rest, [...output, first]> : output; | ||
] ? Equal<first, _> extends true ? ExcludePlaceholders<rest, output> : ExcludePlaceholders<rest, [...output, first]> : output; | ||
type MergeArgsRec<pipedArgs extends any[], partialArgs extends any[], output extends any[] = []> = partialArgs extends [infer partialFirst, ...infer partialRest] ? IsNever<partialFirst> extends true ? MergeArgsRec<pipedArgs, partialRest, [...output, partialFirst]> : [partialFirst] extends [_] ? pipedArgs extends [infer pipedFirst, ...infer pipedRest] ? MergeArgsRec<pipedRest, partialRest, [...output, pipedFirst]> : [...output, ...ExcludePlaceholders<partialRest>] : MergeArgsRec<pipedArgs, partialRest, [...output, partialFirst]> : [...output, ...pipedArgs]; | ||
@@ -8,0 +8,0 @@ type EmptyIntoPlaceholder<x> = IsNever<x> extends true ? never : [x] extends [unset] ? _ : x; |
@@ -32,4 +32,4 @@ import { Fn, PartialApply, unset, _ } from "../core/Core"; | ||
* type T0 = Call<Parameter<1>, (a: number, b: string) => void>; // number | ||
* type T1 = Call2<Parameter, (a: number, b: string) => void, 1>; // string | ||
* type T2 = Eval<Parameter<(a: number, b: string) => void, 0>>; // number | ||
* type T1 = Call<Parameter, 1, (a: number, b: string) => void>; // string | ||
* type T2 = Call<Parameter<0, (a: number, b: string) => void>>; // number | ||
*/ | ||
@@ -47,3 +47,3 @@ export type Parameter<N extends number | _ | unset = unset, fn extends ((...args: any[]) => any) | _ | unset = unset> = PartialApply<ParameterFn, [fn, N]>; | ||
* type T0 = Call<ReturnType, (a: number, b: string) => number>; // number | ||
* type T1 = Eval<ReturnType<(a: number, b: string) => number>>; // number | ||
* type T1 = Call<ReturnType<(a: number, b: string) => number>>; // number | ||
* ``` | ||
@@ -50,0 +50,0 @@ */ |
@@ -1,2 +0,2 @@ | ||
import { arg, Eval, Fn, PartialApply, unset } from "../../core/Core"; | ||
import { arg, Call, Fn, PartialApply, unset } from "../../core/Core"; | ||
import { Primitive, UnionToIntersection } from "../../helpers"; | ||
@@ -37,3 +37,3 @@ type GetWithDefault<Obj, K, Def> = K extends keyof Obj ? Obj[K] : Def; | ||
...infer restPatterns extends With<any, any>[] | ||
] ? DoesMatch<value, pattern> extends true ? handler extends Fn ? Eval<PartialApply<Extract<handler, Fn>, ExtractArgs<value, pattern>>> : handler : Match<value, restPatterns> : never; | ||
] ? DoesMatch<value, pattern> extends true ? handler extends Fn ? Call<PartialApply<Extract<handler, Fn>, ExtractArgs<value, pattern>>> : handler : Match<value, restPatterns> : never; | ||
export type With<pattern, handler> = { | ||
@@ -40,0 +40,0 @@ pattern: pattern; |
@@ -12,4 +12,4 @@ import { Fn, PartialApply, unset, _ } from "../core/Core"; | ||
* ```ts | ||
* type T0 = Eval<Numbers.Add<1, 2>>; // 3 | ||
* type T1 = Eval<Numbers.Add<999999999999999999999999999n, 2>>; // 1000000000000000000000000001n | ||
* type T0 = Call<Numbers.Add<1, 2>>; // 3 | ||
* type T1 = Call<Numbers.Add<999999999999999999999999999n, 2>>; // 1000000000000000000000000001n | ||
* ``` | ||
@@ -33,4 +33,4 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Sub<1, 2>>; // -1 | ||
* type T1 = Eval<Numbers.Sub<1000000000000000000000000001n, 2>>; // 999999999999999999999999999n | ||
* type T0 = Call<Numbers.Sub<1, 2>>; // -1 | ||
* type T1 = Call<Numbers.Sub<1000000000000000000000000001n, 2>>; // 999999999999999999999999999n | ||
* ``` | ||
@@ -54,4 +54,4 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Mul<99, 3>>; // 297 | ||
* type T1 = Eval<Numbers.Mul<999999999999999999999999999n, 2>>; // 1999999999999999999999999998n | ||
* type T0 = Call<Numbers.Mul<99, 3>>; // 297 | ||
* type T1 = Call<Numbers.Mul<999999999999999999999999999n, 2>>; // 1999999999999999999999999998n | ||
* ``` | ||
@@ -75,4 +75,4 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Div<99, 3>>; // 33 | ||
* type T1 = Eval<Numbers.Div<999999999999999999999999999n, 4>>; // 249999999999999999999999999n | ||
* type T0 = Call<Numbers.Div<99, 3>>; // 33 | ||
* type T1 = Call<Numbers.Div<999999999999999999999999999n, 4>>; // 249999999999999999999999999n | ||
* ``` | ||
@@ -96,4 +96,4 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Mod<100, 3>>; // 1 | ||
* type T1 = Eval<Numbers.Mod<999999999999999999999999999n, 4>>; // 3n | ||
* type T0 = Call<Numbers.Mod<100, 3>>; // 1 | ||
* type T1 = Call<Numbers.Mod<999999999999999999999999999n, 4>>; // 3n | ||
* ``` | ||
@@ -116,4 +116,4 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Negate<1>>; // -1 | ||
* type T1 = Eval<Numbers.Negate<999999999999999999999999999n>>; // -999999999999999999999999999n | ||
* type T0 = Call<Numbers.Negate<1>>; // -1 | ||
* type T1 = Call<Numbers.Negate<999999999999999999999999999n>>; // -999999999999999999999999999n | ||
* ``` | ||
@@ -132,4 +132,4 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Abs<-1>>; // 1 | ||
* type T1 = Eval<Numbers.Abs<999999999999999999999999999n>>; // 999999999999999999999999999n | ||
* type T0 = Call<Numbers.Abs<-1>>; // 1 | ||
* type T1 = Call<Numbers.Abs<999999999999999999999999999n>>; // 999999999999999999999999999n | ||
* ``` | ||
@@ -150,3 +150,3 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Max<1, 2>>; // 2 | ||
* type T0 = Call<Numbers.Max<1, 2>>; // 2 | ||
* ``` | ||
@@ -165,3 +165,3 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Min<1, 2>>; // 1 | ||
* type T0 = Call<Numbers.Min<1, 2>>; // 1 | ||
* ``` | ||
@@ -181,3 +181,3 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Power<2, 128>>; // 340282366920938463463374607431768211456 | ||
* type T0 = Call<Numbers.Power<2, 128>>; // 340282366920938463463374607431768211456 | ||
* ``` | ||
@@ -201,5 +201,5 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Compare<1, 2>>; // -1 | ||
* type T1 = Eval<Numbers.Compare<999999999999999999999999999n, 4>>; // 1 | ||
* type T2 = Eval<Numbers.Compare<999999999999999999999999999n, 999999999999999999999999999n>>; // 0 | ||
* type T0 = Call<Numbers.Compare<1, 2>>; // -1 | ||
* type T1 = Call<Numbers.Compare<999999999999999999999999999n, 4>>; // 1 | ||
* type T2 = Call<Numbers.Compare<999999999999999999999999999n, 999999999999999999999999999n>>; // 0 | ||
* ``` | ||
@@ -223,4 +223,4 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.Equal<1, 2>>; // false | ||
* type T1 = Eval<Numbers.Equal<2, 2>>; // true | ||
* type T0 = Call<Numbers.Equal<1, 2>>; // false | ||
* type T1 = Call<Numbers.Equal<2, 2>>; // true | ||
* ``` | ||
@@ -244,4 +244,4 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.NotEqual<1, 2>>; // true | ||
* type T1 = Eval<Numbers.NotEqual<2, 2>>; // false | ||
* type T0 = Call<Numbers.NotEqual<1, 2>>; // true | ||
* type T1 = Call<Numbers.NotEqual<2, 2>>; // false | ||
* ``` | ||
@@ -265,5 +265,5 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.LessThan<1, 2>>; // true | ||
* type T1 = Eval<Numbers.LessThan<2, 2>>; // false | ||
* type T2 = Eval<Numbers.LessThan<3, 2>>; // false | ||
* type T0 = Call<Numbers.LessThan<1, 2>>; // true | ||
* type T1 = Call<Numbers.LessThan<2, 2>>; // false | ||
* type T2 = Call<Numbers.LessThan<3, 2>>; // false | ||
* ``` | ||
@@ -287,5 +287,5 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.LessThanOrEqual<1, 2>>; // true | ||
* type T1 = Eval<Numbers.LessThanOrEqual<2, 2>>; // true | ||
* type T2 = Eval<Numbers.LessThanOrEqual<3, 2>>; // false | ||
* type T0 = Call<Numbers.LessThanOrEqual<1, 2>>; // true | ||
* type T1 = Call<Numbers.LessThanOrEqual<2, 2>>; // true | ||
* type T2 = Call<Numbers.LessThanOrEqual<3, 2>>; // false | ||
* ``` | ||
@@ -309,5 +309,5 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.GreaterThan<1, 2>>; // false | ||
* type T1 = Eval<Numbers.GreaterThan<2, 2>>; // false | ||
* type T2 = Eval<Numbers.GreaterThan<3, 2>>; // true | ||
* type T0 = Call<Numbers.GreaterThan<1, 2>>; // false | ||
* type T1 = Call<Numbers.GreaterThan<2, 2>>; // false | ||
* type T2 = Call<Numbers.GreaterThan<3, 2>>; // true | ||
* ``` | ||
@@ -331,5 +331,5 @@ */ | ||
* ```ts | ||
* type T0 = Eval<Numbers.GreaterThanOrEqual<1, 2>>; // false | ||
* type T1 = Eval<Numbers.GreaterThanOrEqual<2, 2>>; // true | ||
* type T2 = Eval<Numbers.GreaterThanOrEqual<3, 2>>; // true | ||
* type T0 = Call<Numbers.GreaterThanOrEqual<1, 2>>; // false | ||
* type T1 = Call<Numbers.GreaterThanOrEqual<2, 2>>; // true | ||
* type T2 = Call<Numbers.GreaterThanOrEqual<3, 2>>; // true | ||
* ``` | ||
@@ -336,0 +336,0 @@ */ |
import { IsArrayStrict, Prettify } from "../helpers"; | ||
import { Call, Call2, Fn, PartialApply, unset, _ } from "../core/Core"; | ||
import { Call, Fn, PartialApply, unset, _ } from "../core/Core"; | ||
import { Std } from "../std/Std"; | ||
@@ -32,3 +32,3 @@ import { Strings } from "../strings/Strings"; | ||
type MapValuesImpl<T, fn extends Fn> = { | ||
[K in keyof T]: Call2<fn, T[K], K>; | ||
[K in keyof T]: Call<fn, T[K], K>; | ||
}; | ||
@@ -242,3 +242,3 @@ /** | ||
type PickByImpl<T, fn extends Fn> = Impl.FromEntries<PickEntriesImpl<Impl.Entries<T>, fn>>; | ||
type PickEntriesImpl<entries extends [PropertyKey, any], fn extends Fn> = entries extends any ? Call2<fn, entries[1], entries[0]> extends true ? entries : never : never; | ||
type PickEntriesImpl<entries extends [PropertyKey, any], fn extends Fn> = entries extends any ? Call<fn, entries[1], entries[0]> extends true ? entries : never : never; | ||
/** | ||
@@ -262,3 +262,3 @@ * Only keep keys of an object if the predicate function | ||
type OmitByImpl<T, fn extends Fn> = Impl.FromEntries<OmitEntriesImpl<Impl.Entries<T>, fn>>; | ||
type OmitEntriesImpl<entries extends [PropertyKey, any], fn extends Fn> = entries extends any ? Call2<fn, entries[1], entries[0]> extends true ? never : entries : never; | ||
type OmitEntriesImpl<entries extends [PropertyKey, any], fn extends Fn> = entries extends any ? Call<fn, entries[1], entries[0]> extends true ? never : entries : never; | ||
/** | ||
@@ -271,4 +271,4 @@ * Merge several objects together | ||
* type T0 = Call<Objects.Assign<{ a: string }>, { b: number }>; // { a: string, b: number } | ||
* type T1 = Eval<Objects.Assign<{ a: string }, { b: number }>>; // { a: string, b: number } | ||
* type T2 = Eval<Objects.Assign<{ a: 1 }, { b: 1 }, { c: 1 }>>; // { a: 1, b: 1, c: 1 } | ||
* type T1 = Call<Objects.Assign<{ a: string }, { b: number }>>; // { a: string, b: number } | ||
* type T2 = Call<Objects.Assign<{ a: 1 }, { b: 1 }, { c: 1 }>>; // { a: 1, b: 1, c: 1 } | ||
* ``` | ||
@@ -288,3 +288,3 @@ */ | ||
* type T0 = Call<Objects.Readonly, { a: 1; b: true }>; // { readonly a:1; readonly b: true} | ||
* type T1 = Eval<Objects.Readonly<{ a: 1; b: true }>>; // { readonly a:1; readonly b: true} | ||
* type T1 = Call<Objects.Readonly<{ a: 1; b: true }>>; // { readonly a:1; readonly b: true} | ||
* ``` | ||
@@ -304,3 +304,3 @@ */ | ||
* type T0 = Call<Objects.Required, { a?: 1; b?: true }>; // { a:1; b: true} | ||
* type T1 = Eval<Objects.Required<{ a?: 1; b?: true }>>; // { a:1; b: true} | ||
* type T1 = Call<Objects.Required<{ a?: 1; b?: true }>>; // { a:1; b: true} | ||
* ``` | ||
@@ -320,3 +320,3 @@ */ | ||
* type T0 = Call<Objects.Partial, { a: 1; b: true }>; // { a?:1; b?: true} | ||
* type T1 = Eval<Objects.Partial<{ a: 1; b: true }>>; // { a?:1; b?: true} | ||
* type T1 = Call<Objects.Partial<{ a: 1; b: true }>>; // { a?:1; b?: true} | ||
* ``` | ||
@@ -323,0 +323,0 @@ */ |
@@ -1,2 +0,2 @@ | ||
import { Call2 } from "../../core/Core"; | ||
import { Call } from "../../core/Core"; | ||
import { Numbers } from "../../numbers/Numbers"; | ||
@@ -226,3 +226,3 @@ import { StringToTuple } from "./split"; | ||
}; | ||
type CharacterCompare<Char1 extends string, Char2 extends string> = Char1 extends Char2 ? 0 : Char1 extends keyof ascii ? Char2 extends keyof ascii ? Call2<Numbers.Compare, ascii[Char1], ascii[Char2]> : 1 : -1; | ||
type CharacterCompare<Char1 extends string, Char2 extends string> = Char1 extends Char2 ? 0 : Char1 extends keyof ascii ? Char2 extends keyof ascii ? Call<Numbers.Compare, ascii[Char1], ascii[Char2]> : 1 : -1; | ||
type CharactersCompare<T extends string[], U extends string[]> = T extends [ | ||
@@ -229,0 +229,0 @@ infer N1 extends string, |
@@ -10,5 +10,5 @@ import { Call, ComposeLeft, Fn, PartialApply, unset, _ } from "../core/Core"; | ||
* Get the length of a string. | ||
* @warning - 🔥🔥🔥does not work with emojis since they are multiple characters🔥🔥🔥 | ||
* @param args[0] - The string to get the length of. | ||
* @returns The length of the string. | ||
* @warning - 🔥 does not work with emojis since they are multiple characters 🔥 | ||
* @example | ||
@@ -22,5 +22,5 @@ * ```ts | ||
* Get the length of a string. | ||
* @warning - 🔥🔥🔥does not work with emojis since they are multiple characters🔥🔥🔥 | ||
* @param args[0] - The string to get the length of. | ||
* @returns The length of the string. | ||
* @warning - 🔥 does not work with emojis since they are multiple characters 🔥 | ||
* @example | ||
@@ -112,3 +112,2 @@ * ```ts | ||
* Cut a slice of a string out from a start index to an end index. | ||
* @warning - 🔥🔥🔥does not work with emojis since they are multiple characters🔥🔥🔥 | ||
* @param args[0] - The string to slice. | ||
@@ -118,2 +117,3 @@ * @param start - The start index. | ||
* @returns The sliced string. | ||
* @warning - 🔥 does not work with emojis since they are multiple characters 🔥 | ||
* @example | ||
@@ -131,6 +131,6 @@ * ```ts | ||
* Split a string into a tuple of strings. | ||
* @warning - 🔥🔥🔥using an empty sep with emojis in the string will destroy the emoji🔥🔥🔥 | ||
* @param args[0] - The string to split. | ||
* @param sep - The separator to split the string with. | ||
* @returns The split string. | ||
* @warning - 🔥 using an empty sep with emojis in the string will destroy the emoji 🔥 | ||
* @example | ||
@@ -194,5 +194,5 @@ * ```ts | ||
* Split a string into a tuple of each character. | ||
* @warning - 🔥🔥🔥does not work with emojis since they are multiple characters🔥🔥🔥 | ||
* @param args[0] - The string to split. | ||
* @returns The splited string. | ||
* @warning - 🔥 does not work with emojis since they are multiple characters 🔥 | ||
* @example | ||
@@ -356,5 +356,5 @@ * ```ts | ||
* ```ts | ||
* type T0 = Call2<Strings.Compare,"abc","def">; // -1 | ||
* type T1 = Call2<Strings.Compare,"def","abc">; // 1 | ||
* type T2 = Call2<Strings.Compare,"abc","abc">; // 0 | ||
* type T0 = Call<Strings.Compare,"abc","def">; // -1 | ||
* type T1 = Call<Strings.Compare,"def","abc">; // 1 | ||
* type T2 = Call<Strings.Compare,"abc","abc">; // 0 | ||
* ``` | ||
@@ -379,5 +379,5 @@ */ | ||
* ```ts | ||
* type T0 = Call2<Strings.LessThan,"abc","def">; // true | ||
* type T1 = Call2<Strings.LessThan,"def","abc">; // false | ||
* type T2 = Call2<Strings.LessThan,"abc","abc">; // false | ||
* type T0 = Call<Strings.LessThan,"abc","def">; // true | ||
* type T1 = Call<Strings.LessThan,"def","abc">; // false | ||
* type T2 = Call<Strings.LessThan,"abc","abc">; // false | ||
* ``` | ||
@@ -402,5 +402,5 @@ */ | ||
* ```ts | ||
* type T0 = Call2<Strings.LessThanOrEqual,"abc","def">; // true | ||
* type T1 = Call2<Strings.LessThanOrEqual,"def","abc">; // false | ||
* type T2 = Call2<Strings.LessThanOrEqual,"abc","abc">; // true | ||
* type T0 = Call<Strings.LessThanOrEqual,"abc","def">; // true | ||
* type T1 = Call<Strings.LessThanOrEqual,"def","abc">; // false | ||
* type T2 = Call<Strings.LessThanOrEqual,"abc","abc">; // true | ||
*/ | ||
@@ -424,5 +424,5 @@ export type LessThanOrEqual<n1 extends string | _ | unset = unset, n2 extends string | _ | unset = unset> = PartialApply<LessThanOrEqualFn, n2 extends unset ? [unset, n1] : [n1, n2]>; | ||
* ```ts | ||
* type T0 = Call2<Strings.GreaterThan,"abc","def">; // false | ||
* type T1 = Call2<Strings.GreaterThan,"def","abc">; // true | ||
* type T2 = Call2<Strings.GreaterThan,"abc","abc">; // false | ||
* type T0 = Call<Strings.GreaterThan,"abc","def">; // false | ||
* type T1 = Call<Strings.GreaterThan,"def","abc">; // true | ||
* type T2 = Call<Strings.GreaterThan,"abc","abc">; // false | ||
* ``` | ||
@@ -447,5 +447,5 @@ */ | ||
* ```ts | ||
* type T0 = Call2<Strings.GreaterThanOrEqual,"abc","def">; // false | ||
* type T1 = Call2<Strings.GreaterThanOrEqual,"def","abc">; // true | ||
* type T2 = Call2<Strings.GreaterThanOrEqual,"abc","abc">; // true | ||
* type T0 = Call<Strings.GreaterThanOrEqual,"abc","def">; // false | ||
* type T1 = Call<Strings.GreaterThanOrEqual,"def","abc">; // true | ||
* type T2 = Call<Strings.GreaterThanOrEqual,"abc","abc">; // true | ||
* ``` | ||
@@ -452,0 +452,0 @@ */ |
import { Numbers as N, Numbers } from "../numbers/Numbers"; | ||
import { Apply, args, Call, Call2, Eval, Fn, PartialApply, Pipe, unset, _ } from "../core/Core"; | ||
import { Apply, args, Call, Fn, PartialApply, Pipe, unset, _ } from "../core/Core"; | ||
import { Iterator, Prettify, Stringifiable } from "../helpers"; | ||
@@ -18,4 +18,4 @@ import { Objects } from "../objects/Objects"; | ||
* ```ts | ||
* type T0 = Call2<Tuples.At, 1, ["a", "b", "c"]>; // "b" | ||
* type T1 = Eval<Tuples.At<1, ["a", "b", "c"]>>; // "b" | ||
* type T0 = Call<Tuples.At, 1, ["a", "b", "c"]>; // "b" | ||
* type T1 = Call<Tuples.At<1, ["a", "b", "c"]>>; // "b" | ||
* type T2 = Call<Tuples.At<1>, ["a", "b", "c"]>; // "b" | ||
@@ -41,3 +41,3 @@ * ``` | ||
* type T1 = Call<Tuples.IsEmpty, [1, 2, 3]>; // false | ||
* type T2 = Eval<Tuples.IsEmpty<[]>>; // true | ||
* type T2 = Call<Tuples.IsEmpty<[]>>; // true | ||
* ``` | ||
@@ -56,3 +56,3 @@ */ | ||
* type T0 = Call<Tuples.ToUnion, [1, 2, 3]>; // 1 | 2 | 3 | ||
* type T1 = Eval<Tuples.ToUnion<[1, 2, 3]>>; // 1 | 2 | 3 | ||
* type T1 = Call<Tuples.ToUnion<[1, 2, 3]>>; // 1 | 2 | 3 | ||
* ``` | ||
@@ -74,3 +74,3 @@ */ | ||
interface ToIntersectionFn extends Fn { | ||
return: this["args"] extends [infer tuples extends readonly any[], ...any] ? Eval<Tuples.Reduce<IntersectFn, unknown, tuples>> : never; | ||
return: this["args"] extends [infer tuples extends readonly any[], ...any] ? Call<Tuples.Reduce<IntersectFn, unknown, tuples>> : never; | ||
} | ||
@@ -169,9 +169,9 @@ interface IntersectFn extends Fn { | ||
interface FlatMapFn extends Fn { | ||
return: ReduceImpl<this["arg1"], [ | ||
], FlatMapReducer<Extract<this["arg0"], Fn>>>; | ||
return: ReduceImpl<FlatMapReducer<Extract<this["arg0"], Fn>>, [ | ||
], this["arg1"]>; | ||
} | ||
type ReduceImpl<xs, acc, fn extends Fn> = xs extends [ | ||
type ReduceImpl<fn extends Fn, acc, xs> = xs extends [ | ||
infer first, | ||
...infer rest | ||
] ? ReduceImpl<rest, Call2<fn, acc, first>, fn> : xs extends readonly [infer first, ...infer rest] ? ReduceImpl<rest, Call2<fn, acc, first>, fn> : acc; | ||
] ? ReduceImpl<fn, Call<fn, acc, first>, rest> : xs extends readonly [infer first, ...infer rest] ? ReduceImpl<fn, Call<fn, acc, first>, rest> : acc; | ||
/** | ||
@@ -191,3 +191,3 @@ * Apply a reducer function to each element of a tuple starting from the first and return the accumulated result. | ||
interface ReduceFn extends Fn { | ||
return: ReduceImpl<this["arg2"], this["arg1"], Extract<this["arg0"], Fn>>; | ||
return: ReduceImpl<Extract<this["arg0"], Fn>, this["arg1"], this["arg2"]>; | ||
} | ||
@@ -197,3 +197,3 @@ type ReduceRightImpl<xs, acc, fn extends Fn> = xs extends [ | ||
infer last | ||
] ? ReduceRightImpl<rest, Call2<fn, acc, last>, fn> : acc; | ||
] ? ReduceRightImpl<rest, Call<fn, acc, last>, fn> : acc; | ||
/** | ||
@@ -231,4 +231,4 @@ * Apply a reducer function to each element of a tuple starting from the last and return the accumulated result. | ||
export interface FilterFn extends Fn { | ||
return: ReduceImpl<this["arg1"], [ | ||
], FilterReducer<Extract<this["arg0"], Fn>>>; | ||
return: ReduceImpl<FilterReducer<Extract<this["arg0"], Fn>>, [ | ||
], this["arg1"]>; | ||
} | ||
@@ -238,3 +238,3 @@ type FindImpl<xs, fn extends Fn, index extends any[] = []> = xs extends [ | ||
...infer rest | ||
] ? Call2<fn, first, index["length"]> extends true ? first : FindImpl<rest, fn, [...index, any]> : never; | ||
] ? Call<fn, first, index["length"]> extends true ? first : FindImpl<rest, fn, [...index, any]> : never; | ||
/** | ||
@@ -269,3 +269,3 @@ * Apply a predicate function to each element of a tuple and return the first element that satisfies the predicate. | ||
interface SumFn extends Fn { | ||
return: ReduceImpl<this["arg0"], 0, N.Add>; | ||
return: ReduceImpl<N.Add, 0, this["arg0"]>; | ||
} | ||
@@ -312,3 +312,3 @@ type DropImpl<xs extends readonly any[], n extends any[]> = Iterator.Get<n> extends 0 ? xs : xs extends readonly [any, ...infer tail] ? DropImpl<tail, Iterator.Prev<n>> : []; | ||
} | ||
type TakeWhileImpl<xs extends readonly any[], fn extends Fn, index extends any[] = [], output extends any[] = []> = xs extends readonly [infer head, ...infer tail] ? Call2<fn, head, index["length"]> extends true ? TakeWhileImpl<tail, fn, [...index, any], [...output, head]> : output : output; | ||
type TakeWhileImpl<xs extends readonly any[], fn extends Fn, index extends any[] = [], output extends any[] = []> = xs extends readonly [infer head, ...infer tail] ? Call<fn, head, index["length"]> extends true ? TakeWhileImpl<tail, fn, [...index, any], [...output, head]> : output : output; | ||
/** | ||
@@ -372,3 +372,3 @@ * Take the first elements of a tuple that satisfy a predicate function. | ||
...infer tail | ||
] ? Eval<Tuples.Partition<PartialApply<predicateFn, [_, head]>, tail>> extends [infer left extends any[], infer right extends any[]] ? [...SortImpl<left, predicateFn>, head, ...SortImpl<right, predicateFn>] : never : []; | ||
] ? Call<Tuples.Partition<PartialApply<predicateFn, [_, head]>, tail>> extends [infer left extends any[], infer right extends any[]] ? [...SortImpl<left, predicateFn>, head, ...SortImpl<right, predicateFn>] : never : []; | ||
/** | ||
@@ -402,4 +402,4 @@ * Sort a tuple. | ||
* type T0 = Call<Tuples.Join<",">,["a","b","c"]>; // "a,b,c" | ||
* type T1 = Call2<Tuples.Join,",",["a","b","c"]>; // "a,b,c" | ||
* type T2 = Eval<Tuples.Join<",",["a","b","c"]>>; // "a,b,c" | ||
* type T1 = Call<Tuples.Join,",",["a","b","c"]>; // "a,b,c" | ||
* type T2 = Call<Tuples.Join<",",["a","b","c"]>>; // "a,b,c" | ||
* ``` | ||
@@ -409,3 +409,3 @@ */ | ||
interface JoinFn extends Fn { | ||
return: this["args"] extends [infer Sep extends string, infer Tuple] ? ReduceImpl<Tuple, "", JoinReducer<Sep>> : never; | ||
return: this["args"] extends [infer Sep extends string, infer Tuple] ? ReduceImpl<JoinReducer<Sep>, "", Tuple> : never; | ||
} | ||
@@ -453,3 +453,3 @@ /** | ||
* ```ts | ||
* type T0 = Call2<Tuples.Concat, [1], [2, 3]>; // [1, 2, 3] | ||
* type T0 = Call<Tuples.Concat, [1], [2, 3]>; // [1, 2, 3] | ||
* ``` | ||
@@ -490,4 +490,30 @@ */ | ||
} | ||
/** | ||
* SplitAt takes an index and a tuple, splits the tuple | ||
* at the provided index and returns the list of elements | ||
* before this index and the list of elements after this | ||
* index as a [before[], after[]] tuple. | ||
* | ||
* @param index - the index at which to split the list | ||
* @param tuple - The list to split | ||
* @returns A [before[], after[]] tuple. | ||
* @example | ||
* ```ts | ||
* type T0 = Call<Tuples.SplitAt<2>, [1, 2, 3, 4]>; // [[1, 2], [3, 4]] | ||
* type T1 = Call<Tuples.SplitAt<2>, [1]>; // [[1], []] | ||
* ``` | ||
*/ | ||
export type SplitAt<index extends number | unset | _ = unset, tuple extends unknown[] | unset | _ = unset> = PartialApply<SplitAtFn, [index, tuple]>; | ||
export interface SplitAtFn extends Fn { | ||
return: this["args"] extends [ | ||
infer index extends number, | ||
infer tuple extends any[], | ||
...any | ||
] ? [ | ||
TakeImpl<tuple, Iterator.Iterator<index>>, | ||
DropImpl<tuple, Iterator.Iterator<index>> | ||
] : never; | ||
} | ||
interface ZipWithMapper<fn extends Fn, arrs extends unknown[][]> extends Fn { | ||
return: this["args"] extends [infer Index extends number, ...any] ? Apply<fn, Eval<Tuples.Map<Tuples.At<Index>, arrs>>> : never; | ||
return: this["args"] extends [infer Index extends number, ...any] ? Apply<fn, Call<Tuples.Map<Tuples.At<Index>, arrs>>> : never; | ||
} | ||
@@ -513,4 +539,4 @@ interface ZipWithFn<fn extends Fn> extends Fn { | ||
* ```ts | ||
* type T0 = Call2<Tuples.Zip, [1, 2, 3], [10, 2, 5]>; // [[1, 10], [2, 2], [3, 5]] | ||
* type T1 = Eval<Tuples.Zip<[1, 2, 3], [10, 2, 5]>>; // [[1, 10], [2, 2], [3, 5]] | ||
* type T0 = Call<Tuples.Zip, [1, 2, 3], [10, 2, 5]>; // [[1, 10], [2, 2], [3, 5]] | ||
* type T1 = Call<Tuples.Zip<[1, 2, 3], [10, 2, 5]>>; // [[1, 10], [2, 2], [3, 5]] | ||
* ``` | ||
@@ -542,5 +568,5 @@ */ | ||
* ```ts | ||
* type T0 = Call2<Tuples.ZipWith<args>, [1, 2, 3], [10, 2, 5]>; // [[1, 10], [2, 2], [3, 5]] | ||
* type T1 = Eval<Tuples.ZipWith<args, [1, 2, 3], [10, 2, 5]>>; // [[1, 10], [2, 2], [3, 5]] | ||
* type T3 = Call2<Tuples.ZipWith<N.Add>, [1, 2, 3], [10, 2, 5]>; // [11, 4, 8] | ||
* type T0 = Call<Tuples.ZipWith<args>, [1, 2, 3], [10, 2, 5]>; // [[1, 10], [2, 2], [3, 5]] | ||
* type T1 = Call<Tuples.ZipWith<args, [1, 2, 3], [10, 2, 5]>>; // [[1, 10], [2, 2], [3, 5]] | ||
* type T3 = Call<Tuples.ZipWith<N.Add>, [1, 2, 3], [10, 2, 5]>; // [11, 4, 8] | ||
* ``` | ||
@@ -600,5 +626,5 @@ */ | ||
* type T0 = Call<Tuples.Range<3>, 7>; // [3, 4, 5, 6, 7] | ||
* type T1 = Eval<Tuples.Range<_, 10>, 5>; // [5, 6, 7, 8, 9, 10] | ||
* type T3 = Eval<Tuples.Range< -2, 2>, 5>; // [-2, 1, 0, 1, 2] | ||
* type T4 = Eval<Tuples.Range< -5, -2>, 5>; // [-5, -4, -3, -2] | ||
* type T1 = Call<Tuples.Range<_, 10>, 5>; // [5, 6, 7, 8, 9, 10] | ||
* type T3 = Call<Tuples.Range< -2, 2>, 5>; // [-2, 1, 0, 1, 2] | ||
* type T4 = Call<Tuples.Range< -5, -2>, 5>; // [-5, -4, -3, -2] | ||
* ``` | ||
@@ -611,3 +637,3 @@ */ | ||
infer end extends number | ||
] ? Call2<Numbers.LessThanOrEqual, start, end> extends true ? Pipe<start, [ | ||
] ? Call<Numbers.LessThanOrEqual, start, end> extends true ? Pipe<start, [ | ||
Numbers.Sub<end, _>, | ||
@@ -620,3 +646,3 @@ Numbers.Add<1>, | ||
...output, | ||
Eval<Numbers.Add<start, output["length"]>> | ||
Call<Numbers.Add<start, output["length"]>> | ||
]>; | ||
@@ -630,4 +656,4 @@ /** | ||
* type T0 = Call<Tuples.Length, [1, 2, 3]>; // 3 | ||
* type T1 = Eval<Tuples.Length, []>; 0 | ||
* type T2 = Eval<Tuples.Length, ['a']>; 1 | ||
* type T1 = Call<Tuples.Length, []>; 0 | ||
* type T2 = Call<Tuples.Length, ['a']>; 1 | ||
* ``` | ||
@@ -647,3 +673,3 @@ */ | ||
* type T1 = Call<Tuples.Min, [-1, -2, -3]>; // -3 | ||
* type T2 = Eval<Tuples.Min, []>; never | ||
* type T2 = Call<Tuples.Min, []>; never | ||
* ``` | ||
@@ -670,3 +696,3 @@ */ | ||
* type T1 = Call<Tuples.Max, [-1, -2, -3]>; // -1 | ||
* type T2 = Eval<Tuples.Max, []>; never | ||
* type T2 = Call<Tuples.Max, []>; never | ||
* ``` | ||
@@ -673,0 +699,0 @@ */ |
@@ -1,2 +0,2 @@ | ||
import { Call, Eval, Fn, PartialApply, unset, _ } from "../core/Core"; | ||
import { Call, Fn, PartialApply, unset, _ } from "../core/Core"; | ||
import { UnionToIntersection, UnionToTuple } from "../helpers"; | ||
@@ -36,5 +36,5 @@ import { Std } from "../std/Std"; | ||
* type T0 = Call<Unions.Range<3>, 7>; // 3 | 4 | 5 | 6 | 7 | ||
* type T1 = Eval<Unions.Range<_, 10>, 5>; // 5 | 6 | 7 | 8 | 9 | 10 | ||
* type T3 = Eval<Unions.Range< -2, 2>, 5>; // -2 | 1 | 0 | 1 | 2 | ||
* type T4 = Eval<Unions.Range< -5, -2>, 5>; // -5 | -4 | -3 | -2 | ||
* type T1 = Call<Unions.Range<_, 10>, 5>; // 5 | 6 | 7 | 8 | 9 | 10 | ||
* type T3 = Call<Unions.Range< -2, 2>, 5>; // -2 | 1 | 0 | 1 | 2 | ||
* type T4 = Call<Unions.Range< -5, -2>, 5>; // -5 | -4 | -3 | -2 | ||
* ``` | ||
@@ -47,3 +47,3 @@ */ | ||
infer end extends number | ||
] ? Eval<Tuples.Range<start, end>>[number] : never; | ||
] ? Call<Tuples.Range<start, end>>[number] : never; | ||
} | ||
@@ -72,3 +72,3 @@ /** | ||
* type T1 = Pipe<"a" | 1 | null | undefined, [U.NonNullable]>; // 1 | "a" | ||
* type T2 = Eval<Unions.NonNullable<"a" | 1 | null | undefined>>; // 1 | "a" | ||
* type T2 = Call<Unions.NonNullable<"a" | 1 | null | undefined>>; // 1 | "a" | ||
* ``` | ||
@@ -75,0 +75,0 @@ */ |
{ | ||
"name": "hotscript", | ||
"version": "1.0.11", | ||
"version": "1.0.12-rc.0", | ||
"description": "Type-level madness", | ||
"type": "module", | ||
"source": "src/index.ts", | ||
"main": "./dist/index.js", | ||
"main": "./dist/index.cjs", | ||
"module": "./dist/index.js", | ||
"types": "dist/index.d.ts", | ||
"scripts": { | ||
"build": "tsc src/index.ts -d --emitDeclarationOnly --outDir dist", | ||
"build": "tsup src/index.ts --format esm,cjs && tsc src/index.ts --declaration --emitDeclarationOnly --outDir dist", | ||
"prepublishOnly": "npm run test && npm run build", | ||
@@ -36,4 +35,5 @@ "test": "jest", | ||
"ts-jest": "^29.0.5", | ||
"tsup": "^6.7.0", | ||
"typescript": "^4.9.5" | ||
} | ||
} |
287
README.md
# Higher-Order TypeScript (HOTScript) | ||
A library of composable functions for the type-level! Transform your TypeScript types in any way you want using functions you already know. | ||
A library of composable functions for the type level! | ||
Transform your TypeScript types in any way you want using functions you already know. | ||
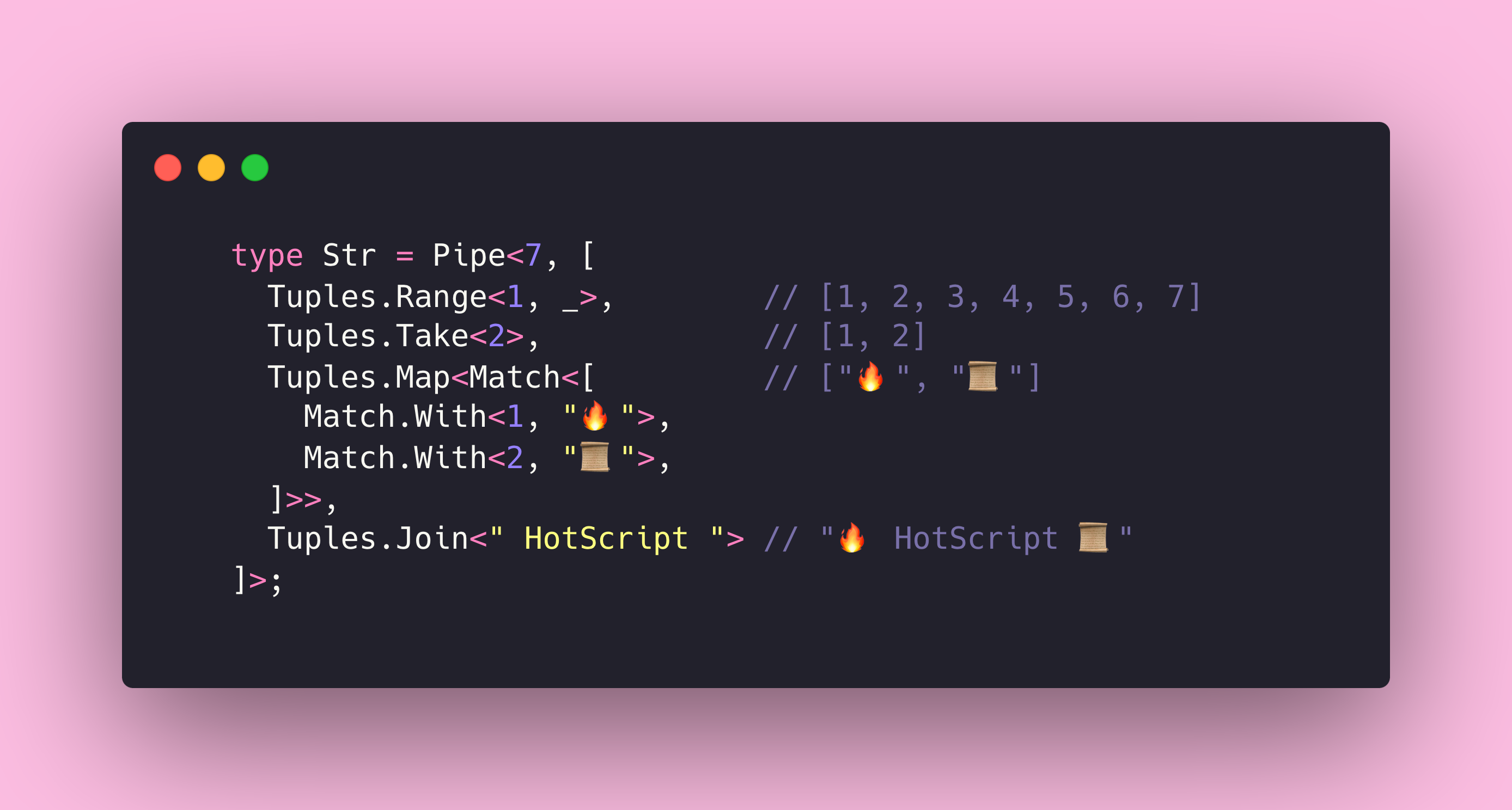 | ||
@@ -32,16 +34,16 @@ | ||
<!-- prettier-ignore --> | ||
```ts | ||
import { Pipe, Tuples, Strings, Numbers } from "hotscript"; | ||
// prettier-ignore | ||
type res1 = Pipe< | ||
// ^? 95 | ||
[1, 2, 3, 4, 3, 4], | ||
// ^? 62 | ||
[1, 2, 3, 4], | ||
[ | ||
Tuples.Map<Numbers.Add<3>>, | ||
Tuples.Join<".">, | ||
Strings.Split<".">, | ||
Tuples.Map<Strings.ToNumber>, | ||
Tuples.Map<Numbers.Add<10>>, | ||
Tuples.Sum | ||
Tuples.Map<Numbers.Add<3>>, // [4, 5, 6, 7] | ||
Tuples.Join<".">, // "4.5.6.7" | ||
Strings.Split<".">, // ["4", "5", "6", "7"] | ||
Tuples.Map<Strings.Prepend<"1">>, // ["14", "15", "16", "17"] | ||
Tuples.Map<Strings.ToNumber>, // [14, 15, 16, 17] | ||
Tuples.Sum // 62 | ||
] | ||
@@ -126,140 +128,141 @@ >; | ||
## TODO | ||
## API | ||
- [ ] Core | ||
- [x] Pipe | ||
- [x] PipeRight | ||
- [x] Call | ||
- [x] Apply | ||
- [x] PartialApply | ||
- [x] Compose | ||
- [x] ComposeLeft | ||
- [ ] Function | ||
- [x] ReturnType | ||
- [x] Parameters | ||
- [x] Parameter n | ||
- [x] Core | ||
- [x] `Pipe<Input, Fn[]>` | ||
- [x] `PipeRight<Fn[], Input>` | ||
- [x] `Call<Fn, ...Arg>` | ||
- [x] `Apply<Fn, Arg[]>` | ||
- [x] `PartialApply<Fn, Arg[]>` | ||
- [x] `Compose<Fn[]>` | ||
- [x] `ComposeLeft<Fn[]>` | ||
- [x] Function | ||
- [x] `ReturnType<Fn>` | ||
- [x] `Parameters<Fn>` | ||
- [x] `Parameter<N, Fn>` | ||
- [ ] Tuples | ||
- [x] Create | ||
- [x] Partition | ||
- [x] IsEmpty | ||
- [x] Zip | ||
- [x] ZipWith | ||
- [x] Sort | ||
- [x] Head | ||
- [x] At | ||
- [x] Tail | ||
- [x] Last | ||
- [x] FlatMap | ||
- [x] Find | ||
- [x] Drop n | ||
- [x] Take n | ||
- [x] TakeWhile | ||
- [x] GroupBy | ||
- [x] Join separator | ||
- [x] Map | ||
- [x] Filter | ||
- [x] Reduce | ||
- [x] ReduceRight | ||
- [x] Every | ||
- [x] Some | ||
- [x] ToUnion | ||
- [x] ToIntersection | ||
- [x] Prepend | ||
- [x] Append | ||
- [x] Concat | ||
- [x] Min | ||
- [x] Max | ||
- [x] Sum | ||
- [x] `Create<X> -> [X]` | ||
- [x] `Partition<Tuple>` | ||
- [x] `IsEmpty<Tuple>` | ||
- [x] `Zip<...Tuple[]>` | ||
- [x] `ZipWith<Fn, ...Tuple[]>` | ||
- [x] `Sort<Tuple>` | ||
- [x] `Head<Tuple>` | ||
- [x] `Tail<Tuple>` | ||
- [x] `At<N, Tuple>` | ||
- [x] `Last<Tuple>` | ||
- [x] `FlatMap<Fn, Tuple>` | ||
- [x] `Find<Fn, Tuple>` | ||
- [x] `Drop<N, Tuple>` | ||
- [x] `Take<N, Tuple>` | ||
- [x] `TakeWhile<Fn, Tuple>` | ||
- [x] `GroupBy<Fn, Tuple>` | ||
- [x] `Join<Str, Tuple>` | ||
- [x] `Map<Fn, Tuple>` | ||
- [x] `Filter<Fn, Tuple>` | ||
- [x] `Reduce<Fn, Init, Tuple>` | ||
- [x] `ReduceRight<Fn, Init, Tuple>` | ||
- [x] `Every<Fn, Tuple>` | ||
- [x] `Some<Fn, Tuple>` | ||
- [x] `SplitAt<N, Tuple>` | ||
- [x] `ToUnion<Tuple>` | ||
- [x] `ToIntersection<Tuple>` | ||
- [x] `Prepend<X, Tuple>` | ||
- [x] `Append<X, Tuple>` | ||
- [x] `Concat<T1, T2>` | ||
- [x] `Min<Tuple>` | ||
- [x] `Max<Tuple>` | ||
- [x] `Sum<Tuple>` | ||
- [ ] Object | ||
- [x] Readonly | ||
- [x] Mutable | ||
- [x] Required | ||
- [x] Partial | ||
- [x] ReadonlyDeep | ||
- [x] MutableDeep | ||
- [x] RequiredDeep | ||
- [x] PartialDeep | ||
- [x] Update | ||
- [x] Record | ||
- [x] Keys | ||
- [x] Values | ||
- [x] AllPaths | ||
- [x] Create | ||
- [x] Get | ||
- [x] FromEntries | ||
- [x] Entries | ||
- [x] MapValues | ||
- [x] MapKeys | ||
- [x] Assign | ||
- [x] Pick | ||
- [x] PickBy | ||
- [x] Omit | ||
- [x] OmitBy | ||
- [x] CamelCase | ||
- [x] CamelCaseDeep | ||
- [x] SnakeCase | ||
- [x] SnakeCaseDeep | ||
- [x] KebabCase | ||
- [x] KebabCaseDeep | ||
- [x] `Readonly<Obj>` | ||
- [x] `Mutable<Obj>` | ||
- [x] `Required<Obj>` | ||
- [x] `Partial<Obj>` | ||
- [x] `ReadonlyDeep<Obj>` | ||
- [x] `MutableDeep<Obj>` | ||
- [x] `RequiredDeep<Obj>` | ||
- [x] `PartialDeep<Obj>` | ||
- [x] `Update<Path, Fn | V, Obj>` | ||
- [x] `Record<Key, Value>` | ||
- [x] `Keys<Obj>` | ||
- [x] `Values<Obj>` | ||
- [x] `AllPaths<Obj>` | ||
- [x] `Create<Pattern, X>` | ||
- [x] `Get<Path, Obj>` | ||
- [x] `FromEntries<[Key, Value]>` | ||
- [x] `Entries<Obj>` | ||
- [x] `MapValues<Fn, Obj>` | ||
- [x] `MapKeys<Fn, Obj>` | ||
- [x] `Assign<...Obj>` | ||
- [x] `Pick<Key, Obj>` | ||
- [x] `PickBy<Fn, Obj>` | ||
- [x] `Omit<Key, Obj>` | ||
- [x] `OmitBy<Fn, Obj>` | ||
- [x] `CamelCase<Obj>` | ||
- [x] `CamelCaseDeep<Obj>` | ||
- [x] `SnakeCase<Obj>` | ||
- [x] `SnakeCaseDeep<Obj>` | ||
- [x] `KebabCase<Obj>` | ||
- [x] `KebabCaseDeep<Obj>` | ||
- [ ] Union | ||
- [x] Map | ||
- [x] Extract | ||
- [x] ExtractBy | ||
- [x] Exclude | ||
- [x] ExcludeBy | ||
- [x] NonNullable | ||
- [x] ToTuple | ||
- [x] ToIntersection | ||
- [x] `Map<Fn, U>` | ||
- [x] `Extract<T, U>` | ||
- [x] `ExtractBy<Fn, U>` | ||
- [x] `Exclude<T, U>` | ||
- [x] `ExcludeBy<Fn, U>` | ||
- [x] `NonNullable<U>` | ||
- [x] `ToTuple<U>` | ||
- [x] `ToIntersection<U>` | ||
- [ ] String | ||
- [x] Length | ||
- [x] TrimLeft | ||
- [x] TrimRight | ||
- [x] Trim | ||
- [x] Join | ||
- [x] Replace | ||
- [x] Slice | ||
- [x] Split | ||
- [x] Repeat | ||
- [x] StartsWith | ||
- [x] EndsWith | ||
- [x] ToTuple | ||
- [x] ToNumber | ||
- [x] ToString | ||
- [x] Prepend | ||
- [x] Append | ||
- [x] Uppercase | ||
- [x] Lowercase | ||
- [x] Capitalize | ||
- [x] Uncapitalize | ||
- [x] SnakeCase | ||
- [x] CamelCase | ||
- [x] KebabCase | ||
- [x] Compare | ||
- [x] Equal | ||
- [x] NotEqual | ||
- [x] LessThan | ||
- [x] LessThanOrEqual | ||
- [x] GreaterThan | ||
- [x] GreaterThanOrEqual | ||
- [x] `Length<Str>` | ||
- [x] `TrimLeft<Str>` | ||
- [x] `TrimRight<Str>` | ||
- [x] `Trim<Str>` | ||
- [x] `Join<Sep, Str>` | ||
- [x] `Replace<From, To, Str>` | ||
- [x] `Slice<Start, End, Str>` | ||
- [x] `Split<Sep, Str>` | ||
- [x] `Repeat<N, Str>` | ||
- [x] `StartsWith<S, Str>` | ||
- [x] `EndsWith<E, Str>` | ||
- [x] `ToTuple<Str>` | ||
- [x] `ToNumber<Str>` | ||
- [x] `ToString<Str>` | ||
- [x] `Prepend<Start, Str>` | ||
- [x] `Append<End, Str>` | ||
- [x] `Uppercase<Str>` | ||
- [x] `Lowercase<Str>` | ||
- [x] `Capitalize<Str>` | ||
- [x] `Uncapitalize<Str>` | ||
- [x] `SnakeCase<Str>` | ||
- [x] `CamelCase<Str>` | ||
- [x] `KebabCase<Str>` | ||
- [x] `Compare<Str, Str>` | ||
- [x] `Equal<Str, Str>` | ||
- [x] `NotEqual<Str, Str>` | ||
- [x] `LessThan<Str, Str>` | ||
- [x] `LessThanOrEqual<Str, Str>` | ||
- [x] `GreaterThan<Str, Str>` | ||
- [x] `GreaterThanOrEqual<Str, Str>` | ||
- [ ] Number | ||
- [x] Add | ||
- [x] Multiply | ||
- [x] Subtract | ||
- [x] Negate | ||
- [x] Power | ||
- [x] Div | ||
- [x] Mod | ||
- [x] Abs | ||
- [x] Compare | ||
- [x] GreaterThan | ||
- [x] GreaterThanOrEqual | ||
- [x] LessThan | ||
- [x] LessThanOrEqual | ||
- [x] `Add<N, M>` | ||
- [x] `Multiply<N, M>` | ||
- [x] `Subtract<N, M>` | ||
- [x] `Negate<N>` | ||
- [x] `Power<N, M>` | ||
- [x] `Div<N, M>` | ||
- [x] `Mod<N, M>` | ||
- [x] `Abs<N>` | ||
- [x] `Compare<N, M>` | ||
- [x] `GreaterThan<N, M>` | ||
- [x] `GreaterThanOrEqual<N, M>` | ||
- [x] `LessThan<N, M>` | ||
- [x] `LessThanOrEqual<N, M>` | ||
- [ ] Boolean | ||
- [x] And | ||
- [x] Or | ||
- [x] XOr | ||
- [x] Not | ||
- [x] Extends | ||
- [x] Equals | ||
- [x] DoesNotExtend | ||
- [x] `And<Bool, Bool>` | ||
- [x] `Or<Bool, Bool>` | ||
- [x] `XOr<Bool, Bool>` | ||
- [x] `Not<Bool>` | ||
- [x] `Extends<A, B>` | ||
- [x] `Equals<A, B>` | ||
- [x] `DoesNotExtend<A, B>` |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
267
167125
6
43
4391
1
No