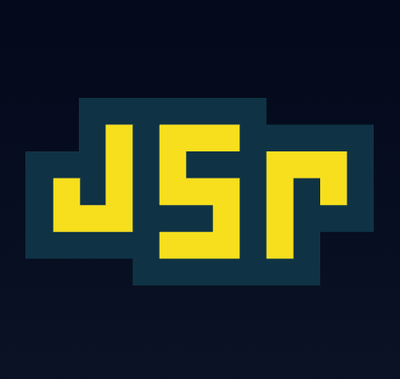
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
A TypeScript Hybrid Public Key Encryption (HPKE) implementation build on top of Web Cryptography API. This library works for both browser-based applications and node.js based applications.
Modes | Browser | Node.js |
---|---|---|
Base | ✅ | ✅ |
PSK | ✅ | ✅ |
Auth | ✅ | ✅ |
AuthPSK |
KEMs | Browser | Node.js |
---|---|---|
DHKEM (P-256, HKDF-SHA256) | ✅ | ✅ |
DHKEM (P-384, HKDF-SHA384) | ✅ | ✅ |
DHKEM (P-521, HKDF-SHA512) | ✅ | ✅ |
DHKEM (X25519, HKDF-SHA256) | ||
DHKEM (X448, HKDF-SHA512) |
KDFs | Browser | Node.js |
---|---|---|
HKDF-SHA256 | ✅ | ✅ |
HKDF-SHA384 | ✅ | ✅ |
HKDF-SHA512 | ✅ | ✅ |
AEADs | Browser | Node.js |
---|---|---|
AES-128-GCM | ✅ | ✅ |
AES-256-GCM | ✅ | ✅ |
ChaCha20Poly1305 | ||
Export Only | ✅ | ✅ |
Install with npm:
npm install hpke-js
This section shows some typical usage examples. See API Documentation for details.
On Node.js:
const { Kem, Kdf, Aead, CipherSuite } = require("hpke-js");
async function doHpke() {
// setup
const suite = new CipherSuite({
kem: Kem.DhkemP256HkdfSha256,
kdf: Kdf.HkdfSha256,
aead: Aead.Aes128Gcm
});
const rkp = await suite.generateKeyPair();
const sender = await suite.createSenderContext({
recipientPublicKey: rkp.publicKey
});
const recipient = await suite.createRecipientContext({
recipientKey: rkp,
enc: sender.enc,
});
// encrypt
const ct = await sender.seal(new TextEncoder().encode("my-secret-message"));
// decrypt
const pt = await recipient.open(ct);
console.log("decrypted: ", new TextDecoder().decode(pt));
// decripted: my-secret-message
}
doHpke();
On Node.js:
const { Kem, Kdf, Aead, CipherSuite } = require("hpke-js");
async function doHpke() {
// setup
const suite = new CipherSuite({
kem: Kem.DhkemP256HkdfSha256,
kdf: Kdf.HkdfSha256,
aead: Aead.Aes128Gcm
});
const rkp = await suite.generateKeyPair();
const sender = await suite.createSenderContext({
recipientPublicKey: rkp.publicKey,
psk: {
id: new TextEncoder().encode("our-pre-shared-key-id"),
key: new TextEncoder().encode("our-pre-shared-key"),
}
});
const recipient = await suite.createRecipientContext({
recipientKey: rkp,
enc: sender.enc,
psk: {
id: new TextEncoder().encode("our-pre-shared-key-id"),
key: new TextEncoder().encode("our-pre-shared-key"),
}
});
// encrypt
const ct = await sender.seal(new TextEncoder().encode("my-secret-message"));
// decrypt
const pt = await recipient.open(ct);
console.log("decrypted: ", new TextDecoder().decode(pt));
// decripted: my-secret-message
}
doHpke();
On Node.js:
const { Kem, Kdf, Aead, CipherSuite } = require("hpke-js");
async function doHpke() {
// setup
const suite = new CipherSuite({
kem: Kem.DhkemP256HkdfSha256,
kdf: Kdf.HkdfSha256,
aead: Aead.Aes128Gcm
});
const rkp = await suite.generateKeyPair();
const skp = await suite.generateKeyPair();
const sender = await suite.createSenderContext({
recipientPublicKey: rkp.publicKey,
senderKey: skp
});
const recipient = await suite.createRecipientContext({
recipientKey: rkp,
enc: sender.enc,
senderPublicKey: skp.publicKey
});
// encrypt
const ct = await sender.seal(new TextEncoder().encode("my-secret-message"));
// decrypt
const pt = await recipient.open(ct);
console.log("decrypted: ", new TextDecoder().decode(pt));
// decripted: my-secret-message
}
doHpke();
On Node.js:
const { Kem, Kdf, Aead, CipherSuite } = require("hpke-js");
async function doHpke() {
// setup
const suite = new CipherSuite({
kem: Kem.DhkemP256HkdfSha256,
kdf: Kdf.HkdfSha256,
aead: Aead.Aes128Gcm
});
const rkp = await suite.generateKeyPair();
const skp = await suite.generateKeyPair();
const sender = await suite.createSenderContext({
recipientPublicKey: rkp.publicKey,
senderKey: skp,
psk: {
id: new TextEncoder().encode("our-pre-shared-key-id"),
key: new TextEncoder().encode("our-pre-shared-key"),
}
});
const recipient = await suite.createRecipientContext({
recipientKey: rkp,
enc: sender.enc,
senderPublicKey: skp.publicKey,
psk: {
id: new TextEncoder().encode("our-pre-shared-key-id"),
key: new TextEncoder().encode("our-pre-shared-key"),
}
});
// encrypt
const ct = await sender.seal(new TextEncoder().encode("my-secret-message"));
// decrypt
const pt = await recipient.open(ct);
console.log("decrypted: ", new TextDecoder().decode(pt));
// decripted: my-secret-message
}
doHpke();
We welcome all kind of contributions, filing issues, suggesting new features or sending PRs.
Version 0.2.2
Released 2022-05-06
FAQs
A Hybrid Public Key Encryption (HPKE) module for various JavaScript runtimes
The npm package hpke-js receives a total of 15,808 weekly downloads. As such, hpke-js popularity was classified as popular.
We found that hpke-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.