
html-attribute-sorter
An html attribute sorter.
You can sort attributes by alphabetical, code guide or idiomatic order.
Installation
$ npm install html-attribute-sorter
$ yarn add html-attribute-sorter
Usage
const { sortAttributes } = require("html-attribute-sorter");
const sorted = sortAttributes(`<img src="foo" class="img b-30" id="img_10">`);
console.log(sorted);
const sorted = sortAttributes(
`<img src="foo" alt="title" class="img b-30" id="img_10">`,
{
order: "alphabetical",
}
);
console.log(sorted);
const sorted = sortAttributes(
`<img src="foo" alt="title" class="img b-30" id="img_10">`,
{
order: "idiomatic",
}
);
console.log(sorted);
const sorted = sortAttributes(
`<img src="foo" alt="title" class="img b-30" id="img_10">`,
{
order: "code_guide",
}
);
console.log(sorted);
API
functions
sortAttributes(body: string, options: ISortOption)
body
: html tag string e.g. <img src="..." alt="title" class="pg-10">
Interfaces
ISortOption
export interface ISortOption {
order: string;
}
key | value |
---|
order | An order to sort attributes. You can specify alphabetical , code_guide or idiomatic . default: code_guide |
Testing
$ yarn install
$ yarn run test
Benchmarking
$ yarn run benchmark
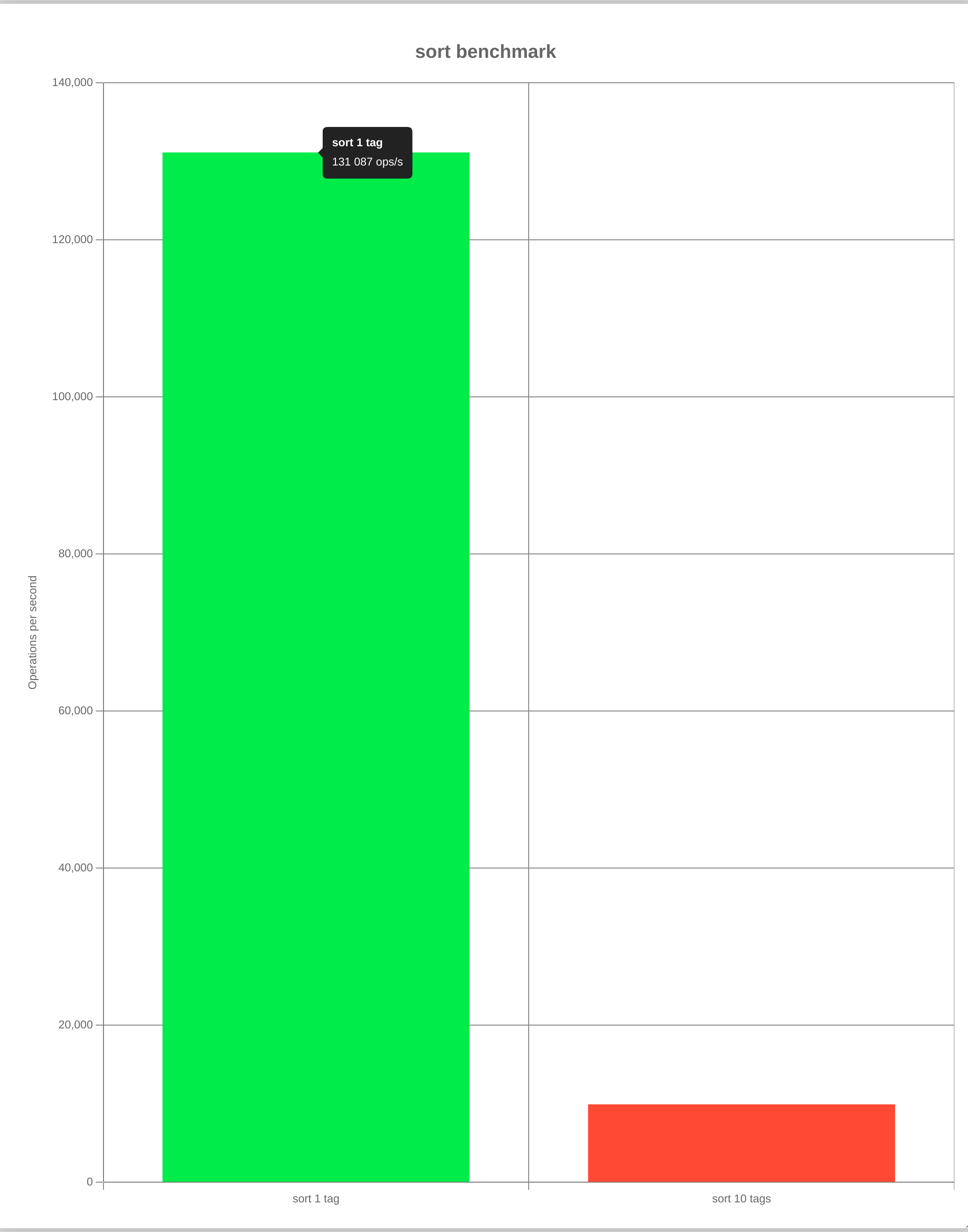
Contributing
- Fork it
- Create your feature branch (
git checkout -b my-new-feature
) - Commit your changes (
git commit -am 'Add some feature'
) - Push to the branch (
git push origin my-new-feature
) - Create new Pull Request
LICENSE
MIT