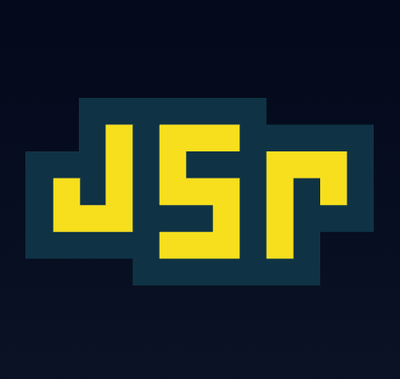
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
html-loader
Advanced tools
The html-loader npm package is used to export HTML as string. When used with webpack, it allows you to require HTML files as modules. It can also minimize the HTML when the webpack 'minimize' option is enabled. It's useful for processing HTML files to include them as part of your JavaScript bundle.
Export HTML as String
Allows you to use HTML files as modules by exporting them as strings. This can be useful when you want to include your HTML in your JavaScript files.
require('html-loader!./file.html')
Interpolate Custom Syntax
Enables interpolation with a custom syntax within the HTML file. This is useful for including images or other assets using a require statement.
require('html-loader?interpolate=require!./file.html')
Minimize HTML
When used with webpack, you can minimize the HTML by setting the 'minimize' option to true. This helps reduce the size of the HTML included in your JavaScript bundle.
module.exports = { module: { rules: [{ test: /\.html$/, use: [{ loader: 'html-loader', options: { minimize: true } }] }] } }
The raw-loader package is similar to html-loader in that it allows you to import files as a string. However, it does not specifically target HTML files and does not have HTML-specific features like minimizing.
The handlebars-loader is used for compiling Handlebars templates and includes them in the webpack bundle. It's similar to html-loader but is tailored for Handlebars template syntax.
The pug-loader package allows you to compile Pug templates and include them in your webpack bundle. It's similar to html-loader but is designed for the Pug templating engine (formerly known as Jade).
Exports HTML as string. HTML is minimized when the compiler demands.
To begin, you'll need to install html-loader
:
npm install --save-dev html-loader
Then add the plugin to your webpack
config. For example:
file.js
import html from './file.html';
webpack.config.js
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
loader: 'html-loader',
},
],
},
};
Name | Type | Default | Description |
---|---|---|---|
attributes | {Boolean|Object} | true | Enables/Disables attributes handling |
preprocessor | {Function} | undefined | Allows pre-processing of content before handling |
minimize | {Boolean|Object} | true in production mode, otherwise false | Tell html-loader to minimize HTML |
esModule | {Boolean} | false | Use ES modules syntax |
attributes
Type: Boolean|Object
Default: true
By default every loadable attributes (for example - <img src="image.png">
) is imported (const img = require('./image.png')
or import img from "./image.png""
).
You may need to specify loaders for images in your configuration (recommended file-loader
or url-loader
).
Supported tags and attributes:
src
attribute of the audio
tagsrc
attribute of the embed
tagsrc
attribute of the img
tagsrcset
attribute of the img
tagsrc
attribute of the input
taghref
attribute of the link
tag (only for stylesheets)data
attribute of the object
tagsrc
attribute of the script
tagsrc
attribute of the source
tagsrcset
attribute of the source
tagsrc
attribute of the track
tagposter
attribute of the video
tagsrc
attribute of the video
tagBoolean
The true
value enables processing of all default elements and attributes, the false
disable processing of all attributes.
webpack.config.js
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
loader: 'html-loader',
options: {
// Disables attributes processing
attributes: false,
},
},
],
},
};
Object
Allows you to specify which tags and attributes to process, filter them, filter urls and process sources starts with /
.
For example:
webpack.config.js
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
loader: 'html-loader',
options: {
attributes: {
list: [
{
tag: 'img',
attribute: 'src',
type: 'src',
},
{
tag: 'img',
attribute: 'srcset',
type: 'srcset',
},
{
tag: 'img',
attribute: 'data-src',
type: 'src',
},
{
tag: 'img',
attribute: 'data-srcset',
type: 'srcset',
},
{
tag: 'link',
attribute: 'href',
type: 'src',
filter: (tag, attribute, attributes) => {
if (!/stylesheet/i.test(attributes.rel)) {
return false;
}
if (
attributes.type &&
attributes.type.trim().toLowerCase() !== 'text/css'
) {
return false;
}
return true;
},
},
// More attributes
],
urlFilter: (attribute, value, resourcePath) => {
// The `attribute` argument contains a name of the HTML attribute.
// The `value` argument contains a value of the HTML attribute.
// The `resourcePath` argument contains a path to the loaded HTML file.
if (/example\.pdf$/.test(value)) {
return false;
}
return true;
},
root: '.',
},
},
},
],
},
};
list
Type: Array
Default: https://github.com/webpack-contrib/html-loader#attributes
Allows to setup which tags and attributes to process and how, and the ability to filter some of them.
For example:
webpack.config.js
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
loader: 'html-loader',
options: {
attributes: {
list: [
{
// Tag name
tag: 'img',
// Attribute name
attribute: 'src',
// Type of processing, can be `src` or `scrset`
type: 'src',
},
{
// Tag name
tag: 'img',
// Attribute name
attribute: 'srcset',
// Type of processing, can be `src` or `scrset`
type: 'srcset',
},
{
tag: 'img',
attribute: 'data-src',
type: 'src',
},
{
tag: 'img',
attribute: 'data-srcset',
type: 'srcset',
},
{
// Tag name
tag: 'link',
// Attribute name
attribute: 'href',
// Type of processing, can be `src` or `scrset`
type: 'src',
// Allow to filter some attributes
filter: (tag, attribute, attributes, resourcePath) => {
// The `tag` argument contains a name of the HTML tag.
// The `attribute` argument contains a name of the HTML attribute.
// The `attributes` argument contains all attributes of the tag.
// The `resourcePath` argument contains a path to the loaded HTML file.
if (/my-html\.html$/.test(resourcePath)) {
return false;
}
if (!/stylesheet/i.test(attributes.rel)) {
return false;
}
if (
attributes.type &&
attributes.type.trim().toLowerCase() !== 'text/css'
) {
return false;
}
return true;
},
},
],
},
},
},
],
},
};
urlFilter
Type: Function
Default: undefined
Allow to filter urls. All filtered urls will not be resolved (left in the code as they were written).
All non requestable sources (for example <img src="javascript:void(0)">
) do not handle by default.
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
loader: 'html-loader',
options: {
attributes: {
urlFilter: (attribute, value, resourcePath) => {
// The `attribute` argument contains a name of the HTML attribute.
// The `value` argument contains a value of the HTML attribute.
// The `resourcePath` argument contains a path to the loaded HTML file.
if (/example\.pdf$/.test(value)) {
return false;
}
return true;
},
},
},
},
],
},
};
root
Type: String
Default: undefined
For urls that start with a /
, the default behavior is to not translate them.
If a root
query parameter is set, however, it will be prepended to the url and then translated.
webpack.config.js
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
loader: 'html-loader',
options: {
attributes: {
root: '.',
},
},
},
],
},
};
preprocessor
Type: Function
Default: undefined
Allows pre-processing of content before handling.
⚠ You should always return valid HTML
file.hbs
<div>
<p>{{firstname}} {{lastname}}</p>
<img src="image.png" alt="alt" />
<div>
webpack.config.js
const Handlebars = require('handlebars');
module.exports = {
module: {
rules: [
{
test: /\.hbs$/i,
loader: 'html-loader',
options: {
preprocessor: (content, loaderContext) => {
let result;
try {
result = Handlebars.compile(content)({
firstname: 'Value',
lastname: 'OtherValue',
});
} catch (error) {
loaderContext.emitError(error);
return content;
}
return result;
},
},
},
],
},
};
minimize
Type: Boolean|Object
Default: true
in production mode, otherwise false
Tell html-loader
to minimize HTML.
Boolean
The enabled rules for minimizing by default are the following ones:
collapseWhitespace
conservativeCollapse
keepClosingSlash
minifyCSS
minifyJS
removeAttributeQuotes
removeComments
removeScriptTypeAttributes
removeStyleTypeAttributes
useShortDoctype
webpack.config.js
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
loader: 'html-loader',
options: {
minimize: true,
},
},
],
},
};
Object
webpack.config.js
See html-minifier-terser's documentation for more information on the available options.
The rules can be disabled using the following options in your webpack.conf.js
webpack.config.js
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
loader: 'html-loader',
options: {
minimize: {
removeComments: false,
collapseWhitespace: false,
},
},
},
],
},
};
esModule
Type: Boolean
Default: false
By default, html-loader
generates JS modules that use the CommonJS modules syntax.
There are some cases in which using ES modules is beneficial, like in the case of module concatenation and tree shaking.
You can enable a ES module syntax using:
webpack.config.js
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
loader: 'html-loader',
options: {
esModule: true,
},
},
],
},
};
webpack.config.js
module.exports = {
module: {
rules: [
{ test: /\.jpg$/, loader: 'file-loader' },
{ test: /\.png$/, loader: 'url-loader' },
],
},
output: {
publicPath: 'http://cdn.example.com/[hash]/',
},
};
<!-- file.html -->
<img src="image.png" data-src="image2x.png" />
require('html-loader!./file.html');
// => '<img src="http://cdn.example.com/49eba9f/a992ca.png" data-src="image2x.png">'
require('html-loader?attributes[]=img:data-src!./file.html');
// => '<img src="image.png" data-src="data:image/png;base64,..." >'
require('html-loader?attributes[]=img:src&attributes[]=img:data-src!./file.html');
// => '<img src="http://cdn.example.com/49eba9f/a992ca.png" data-src="data:image/png;base64,..." >'
require('html-loader?-attributes!./file.html');
// => '<img src="image.jpg" data-src="image2x.png" >'
:warning:
-attributes
it is set attributes: false
'<img src=http://cdn.example.com/49eba9f/a9f92ca.jpg
data-src=data:image/png;base64,...>'
script
and link
tagsscript.file.js
console.log(document);
style.file.css
a {
color: red;
}
file.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Title of the document</title>
<link rel="stylesheet" type="text/css" href="./style.file.css" />
</head>
<body>
Content of the document......
<script src="./script.file.js"></script>
</body>
</html>
webpack.config.js
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
use: ['file-loader?name=[name].[ext]', 'extract-loader', 'html-loader'],
},
{
test: /\.js$/i,
exclude: /\.file.js$/i,
loader: 'babel-loader',
},
{
test: /\.file.js$/i,
loader: 'file-loader',
},
{
test: /\.css$/i,
exclude: /\.file.css$/i,
loader: 'css-loader',
},
{
test: /\.file.css$/i,
loader: 'file-loader',
},
],
},
};
With the same configuration as above:
file.html
<img src="/image.jpg" />
scripts.js
require('html-loader!./file.html');
// => '<img src="/image.jpg">'
other-scripts.js
require('html-loader?root=.!./file.html');
// => '<img src="http://cdn.example.com/49eba9f/a992ca.jpg">'
You can use any template system. Below is an example for handlebars.
file.hbs
<div>
<p>{{firstname}} {{lastname}}</p>
<img src="image.png" alt="alt" />
<div>
webpack.config.js
const Handlebars = require('handlebars');
module.exports = {
module: {
rules: [
{
test: /\.hbs$/i,
loader: 'html-loader',
options: {
preprocessor: (content, loaderContext) => {
let result;
try {
result = Handlebars.compile(content)({
firstname: 'Value',
lastname: 'OtherValue',
});
} catch (error) {
loaderContext.emitError(error);
return content;
}
return result;
},
},
},
],
},
};
You can use PostHTML without any additional loaders.
file.html
<img src="image.jpg" />
webpack.config.js
const posthtml = require('posthtml');
const posthtmlWebp = require('posthtml-webp');
module.exports = {
module: {
rules: [
{
test: /\.hbs$/i,
loader: 'html-loader',
options: {
preprocessor: () => {
let result;
try {
result = posthtml()
.use(plugin)
.process(content, { sync: true });
} catch (error) {
loaderContext.emitError(error);
return content;
}
return result.html;
},
},
},
],
},
};
A very common scenario is exporting the HTML into their own .html file, to serve them directly instead of injecting with javascript. This can be achieved with a combination of 3 loaders:
The html-loader will parse the URLs, require the images and everything you expect. The extract loader will parse the javascript back into a proper html file, ensuring images are required and point to proper path, and the file loader will write the .html file for you. Example:
webpack.config.js
module.exports = {
module: {
rules: [
{
test: /\.html$/i,
use: ['file-loader?name=[name].[ext]', 'extract-loader', 'html-loader'],
},
],
},
};
Please take a moment to read our contributing guidelines if you haven't yet done so.
1.0.0 (2020-03-19)
htmlparser2
packageattrs
option was renamed to the attributes
optioninterpolate
option was removed, please consider migration on the preprocessor
minimize
option is true
by default in production
mode. You need to list all options for html-minifier
if you use object
notation.root
option was moved under the attributes
option, please look at the documentationsrc
attribute of the audio
tagsrc
attribute of the embed
tagsrc
attribute of the img
tagsrcset
attribute of the img
tagsrc
attribute of the input
taghref
attribute of the link
tag (only for stylesheets)data
attribute of the object
tagsrc
attribute of the script
tagsrc
attribute of the source
tagsrcset
attribute of the source
tagsrc
attribute of the track
tagposter
attribute of the video
tagsrc
attribute of the video
tagattributes
option should be Boolean
or Object
, please look at the documentationexportAsDefault
option were removed in favor the esModules
optionexportAsEs6Default
option were removed in favor the esModules
optionpreprocessor
optionesModule
optionrulFilter
option for filtering some of urls, please look at the documentation\u2028
and \u2029
charactersimport
/require
count#hash
in sourcesscript
tags when minimize<a name="0.5.5"></a>
FAQs
Html loader module for webpack
The npm package html-loader receives a total of 938,786 weekly downloads. As such, html-loader popularity was classified as popular.
We found that html-loader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.