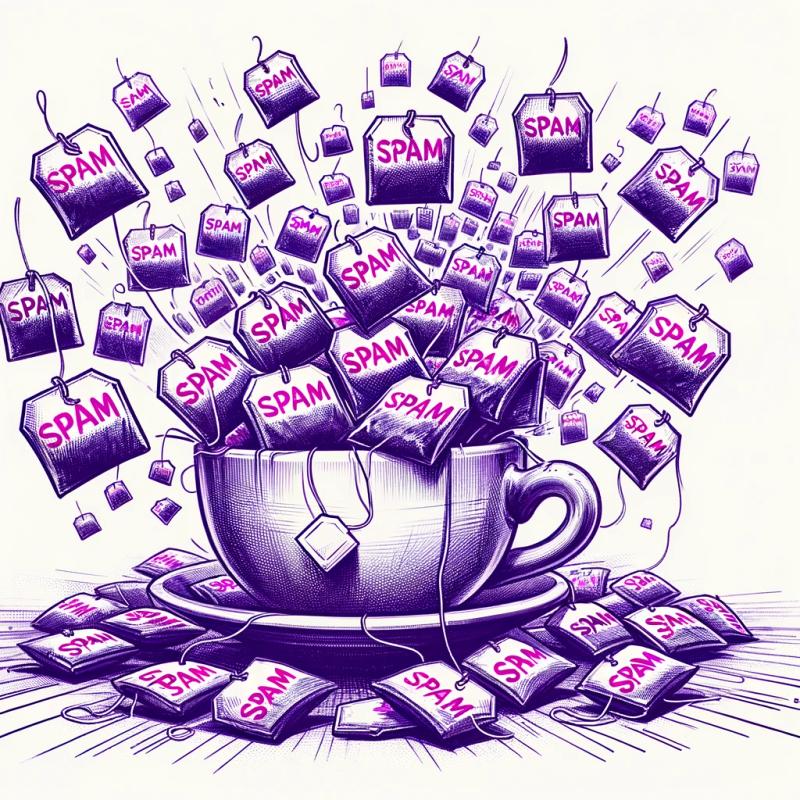
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
http-browserify
Advanced tools
Readme
The http module from node.js, but for browsers.
When you require('http')
in
browserify,
this module will be loaded.
var http = require('http');
http.get({ path : '/beep' }, function (res) {
var div = document.getElementById('result');
div.innerHTML += 'GET /beep<br>';
res.on('data', function (buf) {
div.innerHTML += buf;
});
res.on('end', function () {
div.innerHTML += '<br>__END__';
});
});
var http = require('http');
where opts
are:
opts.method='GET'
- http method verbopts.path
- path string, example: '/foo/bar?baz=555'
opts.headers={}
- as an object mapping key names to string or Array valuesopts.host=window.location.host
- http hostopts.port=window.location.port
- http portopts.responseType
- response type to set on the underlying xhr objectThe callback will be called with the response object.
A shortcut for
options.method = 'GET';
var req = http.request(options, cb);
req.end();
Set an http header.
Get an http header.
Remove an http header.
Write some data to the request body.
If only 1 piece of data is written, data
can be a FormData, Blob, or
ArrayBuffer instance. Otherwise, data
should be a string or a buffer.
Close and send the request body, optionally with additional data
to append.
Return an http header, if set. key
is case-insensitive.
This module has been tested and works with:
Multipart streaming responses are buffered in all versions of Internet Explorer
and are somewhat buffered in Opera. In all the other browsers you get a nice
unbuffered stream of "data"
events when you send down a content-type of
multipart/octet-stream
or similar.
You can do:
var bundle = browserify({
require : { http : 'http-browserify' }
});
in order to map "http-browserify" over require('http')
in your browserified
source.
With npm do:
npm install http-browserify
MIT
FAQs
http module compatability for browserify
The npm package http-browserify receives a total of 109,293 weekly downloads. As such, http-browserify popularity was classified as popular.
We found that http-browserify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.