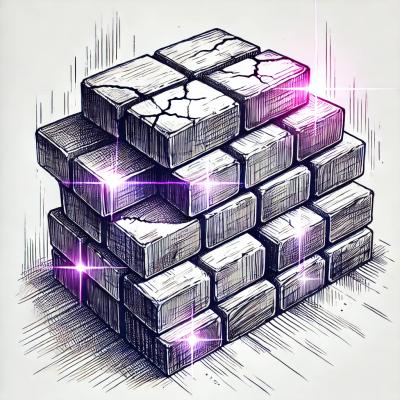
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
https-node.js
Advanced tools
A simple JavaScript HTTP request library for making GET, POST, PUT, DELETE, OPTIONS, HEAD, CONNECT, TRACE, and PATCH requests using the Fetch API.
You can install JS-HTTP using npm:
npm install https-node.js
The project structure is organized as follows:
js-http/
|-- dist/
|-- src/
| |-- js-http.js
|-- examples/
| |-- index.html
|-- tests/
| |-- test-js-http.js
|-- CODE_OF_CONDUCT.md
|-- CONTRIBUTING.md
|-- LEARN.md
|-- README.md
|-- LICENSE
|-- package.json
|-- webpack.config.js
|-- .gitignore
dist/
: Contains the distribution version of the library.src/
: Contains the source code of the library.examples/
: Includes HTML examples demonstrating library usage.tests/
: Contains test files for the library.CODE_OF_CONDUCT.md
: Guidelines for community behavior.CONTRIBUTING.md
: Information on how to contribute to the project.LEARN.md
: Additional resources and learning materials.README.md
: This README file.LICENSE
: The license file for the project.package.json
: Configuration file for npm.webpack.config.js
: Configuration for bundling the library..gitignore
: Specifies files and directories to be ignored by Git.JS-HTTP is a lightweight JavaScript library that simplifies making various HTTP requests in your web applications. It provides a straightforward API for making GET, POST, PUT, DELETE, OPTIONS, HEAD, CONNECT, TRACE, and PATCH requests using the Fetch API.
You can find usage examples in the examples/
directory. To run the examples, open the HTML files in your browser.
Here's how you can use JS-HTTP in your JavaScript code:
// Import the JS-HTTP library
const JSHTTP = require('https-node.js);
Make a GET
request
JSHTTP.get('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
console.log('GET Response:', response);
})
.catch(error => {
console.error('GET Error:', error);
});
Make a POST
request
const data = { userId: 1, id: 101, title: 'foo', body: 'bar' };
JSHTTP.post('https://jsonplaceholder.typicode.com/posts', data)
.then(response => {
console.log('POST Response:', response);
})
.catch(error => {
console.error('POST Error:', error);
});
Make a PUT
request
const putData = { userId: 1, id: 1, title: 'updated title', body: 'updated body' };
JSHTTP.put('https://jsonplaceholder.typicode.com/posts/1', putData)
.then(response => {
console.log('PUT Response:', response);
})
.catch(error => {
console.error('PUT Error:', error);
});
Make a DELETE
request
JSHTTP.delete('https://jsonplaceholder.typicode.com/posts/101')
.then(response => {
console.log('DELETE Response:', response);
})
.catch(error => {
console.error('DELETE Error:', error);
});
Make an OPTIONS
request
const optionsData = { someOption: 'value' };
JSHTTP.options('https://jsonplaceholder.typicode.com/some-resource', optionsData)
.then(response => {
console.log('OPTIONS Response:', response);
})
.catch(error => {
console.error('OPTIONS Error:', error);
});
Make a HEAD
request
const headData = { someHeader: 'value' };
JSHTTP.head('https://jsonplaceholder.typicode.com/some-resource', headData)
.then(response => {
console.log('HEAD Response:', response);
})
.catch(error => {
console.error('HEAD Error:', error);
});
Make a CONNECT
request
const connectData = { someData: 'value' };
JSHTTP.connect('https://jsonplaceholder.typicode.com/some-resource', connectData)
.then(response => {
console.log('CONNECT Response:', response);
})
.catch(error => {
console.error('CONNECT Error:', error);
});
Make a TRACE
request
const traceData = { someData: 'value' };
JSHTTP.trace('https://jsonplaceholder.typicode.com/some
-resource', traceData)
.then(response => {
console.log('TRACE Response:', response);
})
.catch(error => {
console.error('TRACE Error:', error);
});
Make a PATCH
request
const patchData = { someData: 'value' };
JSHTTP.patch('https://jsonplaceholder.typicode.com/some-resource', patchData)
.then(response => {
console.log('PATCH Response:', response);
})
.catch(error => {
console.error('PATCH Error:', error);
});
This project is licensed under the MIT License - see the LICENSE file for details.
Feel free to reach out for questions, feedback, or collaboration opportunities.
FAQs
A simple HTTP request library for JavaScript.
The npm package https-node.js receives a total of 0 weekly downloads. As such, https-node.js popularity was classified as not popular.
We found that https-node.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.