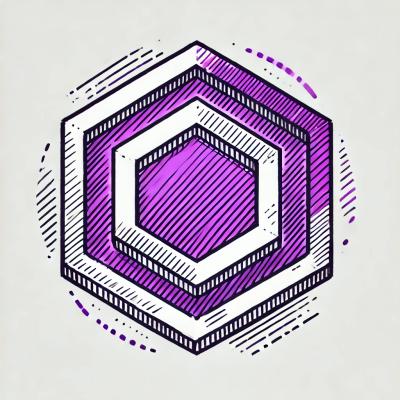
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
A minimal library for object-oriented JavaScript development.
Add hydrogen
to your dependencies, then run:
npm install
Then add:
var h = require('hydrogen');
<script type="text/javascript" src="path/to/hydrogen.js"></script>
See sample/index.html for the examples below plus a few more.
h.create()
can be used to create a prototypical object, or extend one prototypical object from another.
function Character(name, mediumName, otherName) {
this.name = name;
this.mediumName = mediumName;
this.otherName = otherName;
}
h.create(Character, {
getDescription: function() {
return this.getName() + ' is from "' + this.mediumName + '"';
},
getName: function() {
if (this.otherName) {
return this.name + ' (a.k.a. ' + this.otherName + ')';
} else {
return this.name;
}
}
});
function Villain(name, mediumName, otherName, wearsMask) {
this.super.constructor.call(this, name, mediumName, otherName);
this.wearsMask = wearsMask;
}
h.create(Villain, Character, {
getDescription: function() {
return this.getName() + ' is from "' + this.mediumName + '". Mwahahaha!';
}
});
var mcclane = Character.makeInst('John McClane', 'Die Hard');
mcclane.name; // John McClane
mcclane.getDescription(); // John McClane is from "Die Hard"
var vader = Villain.makeInst('Darth Vader', 'Star Wars', 'Anakin Skywalker', true);
vader.getDescription(); // Darth Vader (a.k.a. Anakin Skywalker) is from "Star Wars". Mwahahaha!
h.attach()
adds a closure to an object, which can be used to contain private variables and functions.
function Weapon(name) {
this.name = name;
}
h.attach(Weapon, function() {
var users = [];
return {
addUser: function(user) {
users.push(user);
},
getUsers: function() {
return users;
}
};
});
var bullwhip = Weapon.makeInst('Bullwhip');
bullwhip.users; // undefined
bullwhip.addUser('Indiana Jones');
bullwhip.addUser('Catwoman');
bullwhip.getUsers(); // Indiana Jones,Catwoman
Properties returned by attached closure can be overridden in a child object via either h.create()
or h.attach()
. See sample/index.html for examples.
FAQs
A minimal library for object-oriented JavaScript development
The npm package hydrogen receives a total of 0 weekly downloads. As such, hydrogen popularity was classified as not popular.
We found that hydrogen demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.