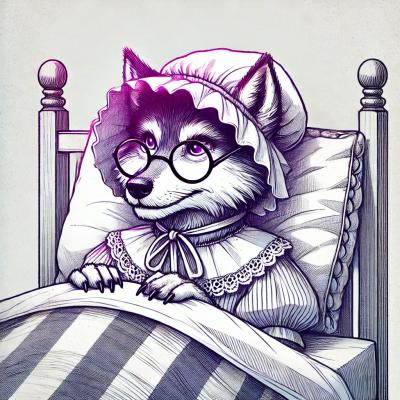
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The i18n npm package is a lightweight internationalization library for Node.js applications. It provides a simple way to manage translations and localization for your application, supporting multiple languages and locales.
Basic Setup
This code demonstrates how to set up the i18n package in a Node.js application. It configures the locales and the directory where translation files are stored, and initializes i18n middleware for use in an Express app.
const i18n = require('i18n');
// Configure i18n
i18n.configure({
locales: ['en', 'es'],
directory: __dirname + '/locales'
});
// Initialize i18n
app.use(i18n.init);
Translation
This feature allows you to translate strings based on the current locale. You can also include variables in the translation strings.
i18n.__('Hello'); // Returns 'Hello' in the current locale
// With variables
i18n.__('Hello %s', 'John'); // Returns 'Hello John' in the current locale
Changing Locale
This code demonstrates how to change the current locale dynamically. After setting the locale to 'es' (Spanish), the translation for 'Hello' will be returned in Spanish.
i18n.setLocale('es');
i18n.__('Hello'); // Returns 'Hola' if the Spanish translation is available
Using in Templates
This feature shows how to use i18n translations within template engines like EJS. The translated string is passed to the template as a variable.
app.get('/', function(req, res) {
res.render('index', { title: res.__('Hello') });
});
i18next is a powerful internationalization framework for JavaScript, including both client-side and server-side support. It offers more advanced features compared to i18n, such as nested translations, pluralization, and context-based translations.
Polyglot.js is a small i18n helper library that provides a simple way to manage translations. It is less feature-rich than i18n but is very lightweight and easy to integrate into small projects.
Node-Polyglot is a Node.js version of Polyglot.js, offering similar functionality for server-side applications. It is a good alternative for projects that require a minimalistic approach to internationalization.
Lightweight simple translation module with dynamic json storage. Uses common __('...') syntax in app and templates. Stores language files in json files compatible to webtranslateit json format. Adds new strings on-the-fly when first used in your app. No extra parsing needed.
npm install i18n
in your app.js
// load modules
var express = require('express'),
i18n = require("i18n");
// register helpers for use in templates
app.helpers({
__: i18n.__
});
// or even a global for use in your app.js
var __= i18n.__;
in your app
var greeting = __('Hello');
in your template (depending on your template compiler)
<%= __('Hello') %>
${__('Hello')}
var greeting = __('Hello %s, how are you today?', 'Marcus');
this puts Hello Marcus, how are you today?. You might add endless arguments and even nest it.
var greeting = __('Hello %s, how are you today? How was your %s.', 'Marcus', __('weekend'));
which puts Hello Marcus, how are you today? How was your weekend.
the above will automatically generate a en.js
by default inside ./locales/
which looks like
{
"Hello":"Hello",
"Hello %s, how are you today?":"Hello %s, how are you today?",
"weekend":"weekend",
"Hello %s, how are you today? How was your %s.":"Hello %s, how are you today? How was your %s."
}
that file can be edited or just uploaded to webtranslateit for any kind of collaborative translation workflow.
FAQs
lightweight translation module with dynamic json storage
The npm package i18n receives a total of 120,270 weekly downloads. As such, i18n popularity was classified as popular.
We found that i18n demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.