What is i18next?
i18next is an internationalization-framework written in and for JavaScript. It provides the means to translate your web application on the client or server side. The framework comes with powerful features like pluralization, formatting, and support for multiple languages.
What are i18next's main functionalities?
Basic translation
This feature allows you to translate text. You initialize i18next with a language and resources, then use the `t` function to translate a key.
i18next.init({ lng: 'en', resources: { en: { translation: { 'key': 'hello world' } } } });
console.log(i18next.t('key')); // output: hello world
Pluralization
This feature supports singular and plural forms based on a count. You define keys for singular and plural forms, and i18next chooses the correct form based on the count provided.
i18next.init({ lng: 'en', resources: { en: { translation: { 'key': 'item', 'key_plural': 'items', 'keyWithCount': '{{count}} item', 'keyWithCount_plural': '{{count}} items' } } } });
console.log(i18next.t('keyWithCount', { count: 1 })); // output: 1 item
console.log(i18next.t('keyWithCount', { count: 5 })); // output: 5 items
Contextual translation
This feature allows for translations to change based on context. You define keys with context, and i18next provides the correct translation for the given context.
i18next.init({ lng: 'en', resources: { en: { translation: { 'friend': 'A friend', 'friend_male': 'A boyfriend', 'friend_female': 'A girlfriend' } } } });
console.log(i18next.t('friend', { context: 'male' })); // output: A boyfriend
console.log(i18next.t('friend', { context: 'female' })); // output: A girlfriend
Language fallback
This feature allows you to specify fallback languages in case a translation is missing in the current language. If a key is not found in the current language, i18next will try to find it in the fallback language.
i18next.init({ lng: 'en', fallbackLng: 'fr', resources: { en: { translation: { 'key': 'hello world' } }, fr: { translation: { 'key': 'bonjour le monde' } } } });
console.log(i18next.t('key')); // output: hello world
i18next.changeLanguage('es');
console.log(i18next.t('key')); // output: bonjour le monde
Formatting
This feature allows you to include variables and formatting within your translations. You can pass variables to the translation function and format them within the translation string.
i18next.init({ lng: 'en', resources: { en: { translation: { 'key': 'The current date is {{date, MM/DD/YYYY}}' } } } });
console.log(i18next.t('key', { date: new Date() })); // output: The current date is 04/01/2023
Other packages similar to i18next
react-intl
React Intl is part of the FormatJS suite which provides internationalization support for React applications. It uses a similar approach to i18next with a focus on React components, but it is more tightly integrated with React and includes components like <FormattedMessage> for rendering translated messages.
polyglot.js
Polyglot.js is a tiny I18n helper library that provides simple translation support with variable interpolation. It is smaller and simpler than i18next, but it lacks some of the more advanced features like pluralization and context handling.
vue-i18n
Vue I18n is an internationalization plugin for Vue.js. It is very similar to i18next but is designed specifically for Vue.js applications. It provides many of the same features but integrates more seamlessly with Vue's reactivity system.
globalize
Globalize is a library for internationalization and localization that leverages the official Unicode CLDR JSON data. It is more focused on providing accurate number formatting, date and time formatting, and message formatting across cultures, rather than just translation.
i18next: learn once - translate everywhere 

i18next is a very popular internationalization framework for browser or any other javascript environment (eg. Node.js, Deno).
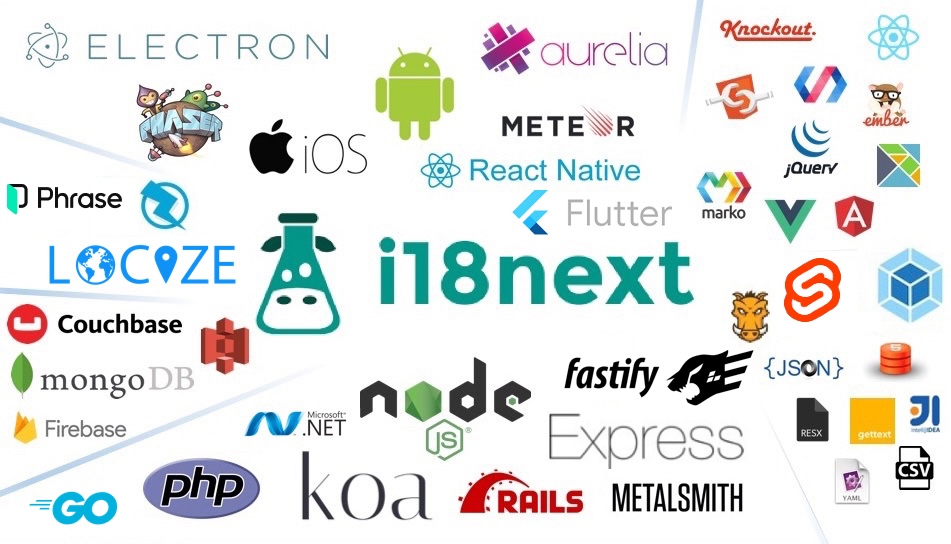
i18next provides:
For more information visit the website:
Our focus is providing the core to building a booming ecosystem. Independent of the building blocks you choose, be it react, angular or even good old jquery proper translation capabilities are just one step away.
Documentation
The general i18next documentation is published on www.i18next.com and PR changes can be supplied here.
The react specific documentation is published on react.i18next.com and PR changes can be supplied here.
From the creators of i18next: localization as a service - locize.com
A translation management system built around the i18next ecosystem - locize.com.

With using locize you directly support the future of i18next.