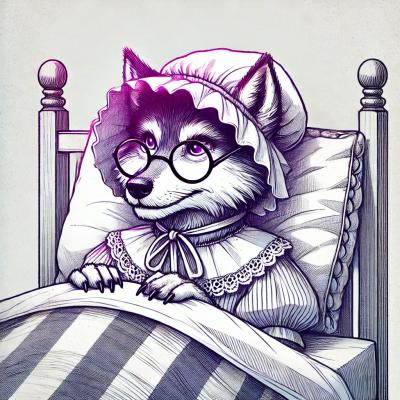
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Interactive Brokers API addon for Node.js compatible with IB API 9.69
This addon uses the latest stable Interactive Brokers POSIX C++ API.
Author: Jae Yang - [dchem] (https://github.com/dchem/)
For direct JavaScript implementation of IB API for Node.js, please visit Pilwon Huh's [node-ib] (https://github.com/pilwon/node-ib).
node-gyp rebuild
node-gyp --msvs_version=2012 rebuild
var addon = require('ibapi');
var obj = new addon.NodeIbapi();
var orderId = -1;
var processIbMsg = function () {
obj.processIbMsg();
}
var addReqId = function () {
obj.addReqId(1);
}
var doReqFunc = function () {
obj.doReqFunc();
}
obj.on('connected', function () {
console.log('connected');
setInterval(processIbMsg,0.1);
obj.funcQueue.push(addReqId);
})
.once('nextValidId', function (data) {
orderId = data.orderId;
console.log('nextValidId: ' + orderId);
setInterval(doReqFunc,100);
})
.on('disconnected', function () {
console.log('disconnected');
process.exit(1);
})
obj.connectToIb('127.0.0.1',7496,0);
The following commands are extended commands in ibapi.js.
.addReqId() // requests nextValidId, wraps reqIds(1)
.doReqFunc() // runs request functions in a function queue
.processIbMsg() // processes incoming and outgoing messages
.connectToIb() // connects to IB and returns a connected or connectionFail event
// Msg processor
// a POSIX implementation of network message handler
.processMsg()
// IB API Commands
// Following commands are used for requesting specific action
// through IB API
// Whenever you need to input contract, it must be js object contract see
// lib/contract.js
// Likewise, for scanner subscription, see lib/scannerSubscription.js
.connect(host,port,clientId)
.disconnect()
.isConnected()
.reqMktData(reqId, contract, genericTickType, snapShot)
.cancelMktData(reqId)
.placeOrder(orderId, contract, action, quantity, orderType, price)
.cancelOrder(orderId)
.reqOpenOrders()
.reqAccountUpdates(subscribe, acctCode)
.reqExecutions(reqId, clientId, acctCode, time, symbol, secType, exchange, side)
.reqIds(1)
.checkMessages()
.reqContractDetails(reqId, contract)
.reqMktDepth(tickerId, contract, numRows )
.cancelMktDepth(tickerId)
.reqNewsBulletins(allMsgs)
.cancelNewsBulletins()
.setServerLogLevel(level)
.reqAutoOpenOrders(bAutoBind)
.reqAllOpenOrders()
.reqManagedAccts()
.requestFA( ) // not yet implemented
.replaceFA( ) // not yet implemented
.reqHistoricalData(id, contract, endDateTime, durationStr, barSizeSetting, whatToShow, useRTH, formatDate)
.exerciseOptions(tickerId, contract, exerciseAction, exerciseQuantity, account, override )
.cancelHistoricalData(tickerId)
.reqRealtimeBars(tickerId, contract, barSize, whatToShow, useRTH)
.cancelRealTimeBars(tickerId)
.cancelScannerSubscription(tickerId)
.reqScannerParameters()
.reqScannerSubscription(tickerId, subscription)
.reqCurrentTime() // not implemented
.reqFundamentalData( reqId, contract, reportType )
.cancelFundamentalData(reqId)
.calculateImpliedVolatility( reqId, contract, optionPrice, underPrice )
.calculateOptionPrice( reqId, contract, volatility, underPrice )
.cancelCalculateImpliedVolatility(reqId)
.cancelCalculateOptionPrice(reqId)
.reqGlobalCancel()
.reqMarketDataType(marketDataType)
.reqPositions()
.cancelPositions()
.reqAccountSummary( reqId, groupName, tags )
.cancelAccountSummary(reqId)
// processIbMsg events - returns arrays
.on('tickPrice', function( tickPrice ) )
.on('tickSize', function( tickSize ) )
.on('tickOptionComputation', function( tickOptionComputation ) )
.on('tickGeneric', function( tickGeneric ) )
.on('tickString', function( tickString ) )
.on('tickEFP', function( tickEFP ) )
.on('orderStatus', function( orderStatus ) )
.on('openOrder', function( openOrder ) )
.on('openOrderEnd', function( openOrderEnd ) )
.on('clientError', function( clientError ) )
.on('connectionClosed', function( connectionClosed ) )
.on('updateAccountValue', function( updateAccountValue ) )
.on('updatePortfolio', function( updatePortfolio ) )
.on('updateAccountTime', function( updateAccountTime ) )
.on('accountDownloadEnd', function( accountDownloadEnd ) )
.on('nextValidId', function( nextValidId ) )
.on('contractDetails', function( contractDetails ) )
.on('bondContractDetails', function( bondContractDetails ) )
.on('contractDetailsEnd', function( contractDetailsEnd ) )
.on('execDetails', function( execDetails ) )
.on('execDetailsEnd', function( execDetailsEnd ) )
.on('svrError', function( svrError ) )
.on('updateMktDepth', function( updateMktDepth ) )
.on('updateMktDepthL2', function( updateMktDepthL2 ) )
.on('updateNewsBulletin', function( updateNewsBulletin ) )
.on('managedAccounts', function( managedAccounts ) )
.on('receiveFA', function( receiveFA ) )
.on('historicalData', function( historicalData ) )
.on('scannerParameters', function( scannerParameters ) )
.on('scannerData', function( scannerData ) )
.on('scannerDataEnd', function( scannerDataEnd ) )
.on('realtimeBar', function( realtimeBar ) )
.on('fundamentalData', function( fundamentalData ) )
.on('deltaNeutralValidation', function( deltaNeutralValidation ) )
.on('tickSnapshotEnd', function( tickSnapshotEnd ) )
.on('marketDataType', function( marketDataType ) )
.on('commissionReport', function( commissionReport ) )
.on('position', function( position ) )
.on('positionEnd', function( positionEnd ) )
.on('accountSummary', function( accountSummary ) )
.on('accountSummaryEnd', function( accountSummaryEnd ) )
.on('nextValidId', function( nextValidId ) )
.on('disconnected', function ())
// connectToIb events
.on('connected', function())
.on('connectionFail' function())
Uses mocha, so install it.
Copyright (c) 2014 Jae Yang. See LICENSE file for license rights and limitations (MIT).
FAQs
Interactive Brokers API addon for NodeJS
The npm package ibapi receives a total of 12 weekly downloads. As such, ibapi popularity was classified as not popular.
We found that ibapi demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.