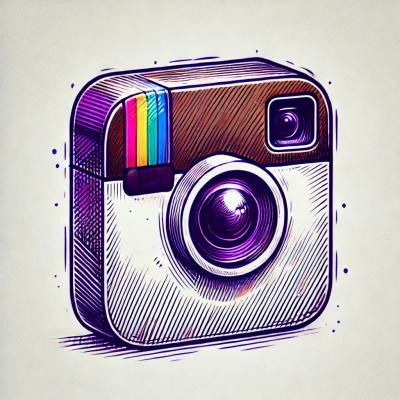
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
ical-generator
Advanced tools
ical-generator is a small piece of code which generates ical calendar files
Supply Chain Security
Vulnerability
Quality
Maintenance
License
The ical-generator npm package is a powerful tool for creating iCalendar (.ics) files in Node.js. It allows developers to generate calendar events programmatically, which can be used for scheduling, reminders, and other time-based functionalities.
Create a Calendar
This feature allows you to create a new calendar instance with a specified domain and name. The `toString` method converts the calendar object to an iCalendar string format.
const ical = require('ical-generator');
const calendar = ical({
domain: 'example.com',
name: 'My Calendar'
});
console.log(calendar.toString());
Add Events
This feature allows you to add events to the calendar. You can specify the start and end times, summary, description, and location of the event.
const ical = require('ical-generator');
const calendar = ical();
calendar.createEvent({
start: new Date(),
end: new Date(new Date().getTime() + 3600000),
summary: 'Meeting',
description: 'Discuss project updates',
location: 'Office'
});
console.log(calendar.toString());
Set Timezone
This feature allows you to set the timezone for the calendar and its events. This is useful for ensuring that events are displayed at the correct local time.
const ical = require('ical-generator');
const calendar = ical({
timezone: 'America/New_York'
});
calendar.createEvent({
start: new Date(),
end: new Date(new Date().getTime() + 3600000),
summary: 'Meeting',
timezone: 'America/New_York'
});
console.log(calendar.toString());
Generate .ics File
This feature allows you to generate an .ics file from the calendar object. The .ics file can be shared and imported into various calendar applications.
const ical = require('ical-generator');
const fs = require('fs');
const calendar = ical();
calendar.createEvent({
start: new Date(),
end: new Date(new Date().getTime() + 3600000),
summary: 'Meeting'
});
fs.writeFileSync('calendar.ics', calendar.toString());
node-ical is a package for parsing iCalendar files. Unlike ical-generator, which focuses on creating iCalendar files, node-ical is used for reading and parsing existing .ics files. It can extract events, todos, and other components from an iCalendar file.
icalendar is a comprehensive library for both creating and parsing iCalendar files. It offers more extensive functionality compared to ical-generator, including support for more iCalendar components like VTODO and VJOURNAL. However, it may have a steeper learning curve due to its broader feature set.
ical.js is a low-level library for parsing and manipulating iCalendar data. It provides fine-grained control over iCalendar components and properties, making it suitable for advanced use cases. However, it requires more effort to use compared to ical-generator, which offers a simpler API for common tasks.
ical-generator
is a small but fine library with which you can very easily create a valid iCal calendars, for example
to generate subscriptionable calendar feeds.
npm install ical-generator
import ical, { ICalCalendarMethod } from 'ical-generator';
import http from 'node:http';
const calendar = ical({ name: 'my first iCal' });
// A method is required for outlook to display event as an invitation
calendar.method(ICalCalendarMethod.REQUEST);
const startTime = new Date();
const endTime = new Date();
endTime.setHours(startTime.getHours() + 1);
calendar.createEvent({
start: startTime,
end: endTime,
summary: 'Example Event',
description: 'It works ;)',
location: 'my room',
url: 'http://sebbo.net/',
});
http.createServer((req, res) => {
res.writeHead(200, {
'Content-Type': 'text/calendar; charset=utf-8',
'Content-Disposition': 'attachment; filename="calendar.ics"',
});
res.end(calendar.toString());
}).listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
See the examples folder for more examples.
ical-generator supports native Date,
Day.js, Luxon's DateTime
and the older moment.js, moment-timezone
objects and TZDate. You can also pass a string which is then passed to javascript's Date
internally.
It is recommended to use UTC time as far as possible. ical-generator
will output all time information as UTC time as
long as no time zone is defined. For day.js, a plugin is necessary for this, which is a prerequisite. If a time zone is
set, ical-generator
assumes that the given time matches the time zone. If a time zone is used, it is also recommended
to use a VTimezone generator. Such a function generates a VTimezone entry and returns it. For example, ical-timezones can
be used for this:
import { ICalCalendar } from 'ical-generator';
import { getVtimezoneComponent } from '@touch4it/ical-timezones';
const cal = new ICalCalendar();
cal.timezone({
name: 'FOO',
generator: getVtimezoneComponent,
});
cal.createEvent({
start: new Date(),
timezone: 'Europe/London',
});
If a moment-timezone
object or Luxon's setZone
method works, ical-generator
sets it according to the time zone set
in the calendar/event.
npm test
npm run coverage
It's here. If you need the changelog for
ical-generator
1.x.x and older, you'll find it here.
ReferenceError: TextEncoder is not defined
error (in some browsers)This library uses TextEncoder
, which
is available in node.js ≥ 11.0.0 and all modern browsers.
Outdated browsers may not have the necessary API and generate this error when generating the calendar.
Copyright (c) Sebastian Pekarek under the MIT license.
FAQs
ical-generator is a small piece of code which generates ical calendar files
The npm package ical-generator receives a total of 159,149 weekly downloads. As such, ical-generator popularity was classified as popular.
We found that ical-generator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.