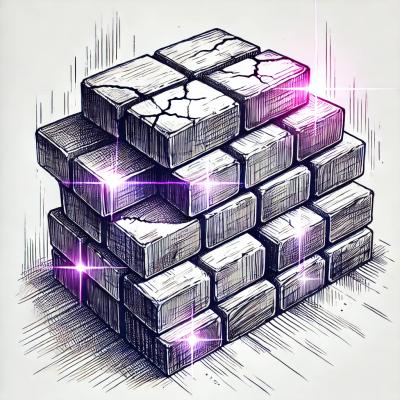
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
idb-pconnector
Advanced tools
Project Status: (production ready as a "technology preview")
Objective: provide a promise-based database connector for DB2 on IBM i.
This project is a promise-based wrapper over the idb-connector
project that enables the use of modern JavaScript's async/await syntax.
Connection Pooling is supported by using the DBPool
class.
The DBPool
class includes integrated aggregates (runSql and prepareExecute) to simplify your code.
npm install idb-pconnector
NOTE
This package only installs on IBM i systems.
Using exec
method to run a select statement and return the result set:
const { Connection, Statement, } = require('idb-pconnector');
async function execExample() {
const connection = new Connection({ url: '*LOCAL' });
const statement = new Statement(connection);
const results = await statement.exec('SELECT * FROM QIWS.QCUSTCDT');
console.log(`results:\n ${JSON.stringify(results)}`);
}
execExample().catch((error) => {
console.error(error);
});
Using prepare
, bind
, and execute
methods to insert data:
const {
Connection, Statement, IN, NUMERIC, CHAR,
} = require('idb-pconnector');
async function pbeExample() {
const connection = new Connection({ url: '*LOCAL' });
const statement = new Statement(connection);
const sql = 'INSERT INTO QIWS.QCUSTCDT VALUES (?,?,?,?,?,?,?,?,?,?,?) with NONE';
await statement.prepare(sql);
await statement.bindParam([
[9997, IN, NUMERIC],
['Johnson', IN, CHAR],
['A J', IN, CHAR],
['453 Example', IN, CHAR],
['Fort', IN, CHAR],
['TN', IN, CHAR],
[37211, IN, NUMERIC],
[1000, IN, NUMERIC],
[1, IN, NUMERIC],
[150, IN, NUMERIC],
[0.00, IN, NUMERIC],
]);
await statement.execute();
}
pbeExample().catch((error) => {
console.error(error);
});
Using DBPool
to return a connection then call a stored procedure:
const { DBPool } = require('idb-pconnector');
async function poolExample() {
const pool = new DBPool();
const connection = pool.attach();
const statement = connection.getStatement();
const sql = `CALL QSYS2.SET_PASE_SHELL_INFO('*CURRENT', '/QOpenSys/pkgs/bin/bash')`
await statement.prepare(sql);
await statement.execute();
if (results) {
console.log(`results:\n ${JSON.stringify(results)}`);
}
await pool.detach(connection);
}
poolExample().catch((error) => {
console.error(error);
});
Using prepareExecute
method to insert data:
const { DBPool } = require('idb-pconnector');
async function prepareExecuteExample() {
/*
* Prepare and execute an SQL statement.
* Params are passed as an array values.
* The order of the params indexed in the array
* should map to the order of the parameter markers
*/
const pool = new DBPool();
const sql = 'INSERT INTO QIWS.QCUSTCDT VALUES (?,?,?,?,?,?,?,?,?,?,?) with NONE';
const params = [4949, 'Johnson', 'T J', '452 Broadway', 'MSP', 'MN',
9810, 2000, 1, 250, 0.00];
await pool.prepareExecute(sql, params);
}
prepareExecuteExample().catch((error) => {
console.error(error);
});
Using runSql
method to directly execute a select statement:
NOTE
This method will not work with stored procedures use prepareExecute() instead.
const { DBPool } = require('idb-pconnector');
async function runSqlExample() {
/*
* Directly execute a statement by providing the SQL to the runSql() function.
*/
const pool = new DBPool();
const results = await pool.runSql('SELECT * FROM QIWS.QCUSTCDT');
if (results) {
console.log(`results:\n ${JSON.stringify(results)}`);
}
}
runSqlExample().catch((error) => {
console.error(error);
});
Change to system naming and set the library list (using CHGLIBL
) of the connection.
const { Connection } = require('idb-pconnector');
async function setLibListExample() {
const connection = new Connection({ url: '*LOCAL' });
await connection.setLibraryList(['QIWS', 'QXMLSERV']);
const statement = connection.getStatement();
const results = await statement.exec('SELECT * FROM QCUSTCDT');
console.log(`results:\n ${JSON.stringify(results)}`);
await statement.close();
}
setLibListExample().catch((error) => {
console.error(error);
});
Please read the docs.
Please read the contribution guidelines.
1.0.7
Fix up setLibraryList example require statement see 03ce835
FAQs
Promise based DB2 Connector for IBM i
The npm package idb-pconnector receives a total of 62 weekly downloads. As such, idb-pconnector popularity was classified as not popular.
We found that idb-pconnector demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.