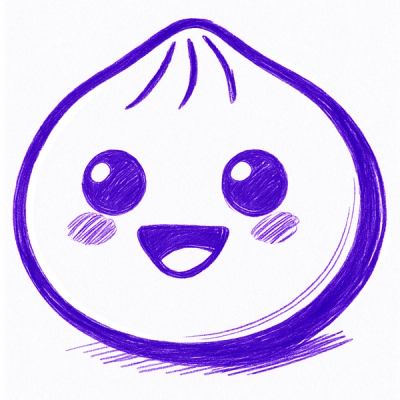
Security News
Bun 1.2.19 Adds Isolated Installs for Better Monorepo Support
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
SQL Syntax Highlighter and Logger. Unadorned and customizable.
$ npm install igniculus
const igniculus = require('igniculus')();
igniculus('SELECT [port] AS Printer, \'on fire\' AS Status ' +
'FROM [Printers] P ' +
'WHERE P."online" = 1 AND P."check" = 1');
A reference to the log function is returned on initialization but can be accessed anywhere through .log
// config.js
const igniculus = require('igniculus');
const options = { ... };
igniculus(options);
// any.js
const igniculus = require('igniculus');
let query = 'SELECT ...';
igniculus.log(query);
A default color scheme is provided. However, you can define the highlight style for each rule when instantiating:
const igniculus = require('igniculus');
/* White constants over red background using inverse mode.
* Gray keywords.
* Prefixed by white '(query)' message.
*/
const options = {
constants: { mode: 'inverse', fg: 'red', bg: 'white' },
standardKeywords: { mode: 'bold', fg: 'black' },
lesserKeywords: { mode: 'bold', fg: 'black' },
prefix: { mode: 'bold', fg: 'white', text: '(query) '}
};
const illumine = igniculus(options);
illumine('SELECT * FROM Student s ' +
'WHERE s.programme = \'IT\' AND EXISTS (' +
'SELECT * FROM Enrolled e ' +
'JOIN Class c ON c.code = e.code ' +
'JOIN Tutor t ON t.tid = c.tid ' +
'WHERE e.sid = s.sid AND t.name LIKE \'%Hoffman\')');
The options argument is optional and each property should be one of the following.
-- comments
or /* Author: undre4m */
'static'
2.5
+
or >=
@name
or @@IDENTITY
[Employee]
or "salary"
INTEGER
or VARCHAR
['SERIAL', 'TIMESTAMP']
'lowercase'
or 'uppercase'
. If not defined data types won't be capitalized.SELECT
or CONSTRAINT
['CLUSTER', 'NATURAL']
'lowercase'
or 'uppercase'
. If not defined standard keywords won't be capitalized.ANY
, AVG
or DESC
['VOLATILE', 'ASYMMETRIC']
'lowercase'
or 'uppercase'
. If not defined lesser keywords won't be capitalized.Executing (default|transaction_id):
This is removed by default by the option prefix: { replace: /.*?: / }
console.log
by default. E.g: process.stdout
, st => st
If defined, the options argument takes precedence over default options. If a rule or it's style is missing it won't be applied. This allows to "enable" or "disable" certain syntax highlighting as you see fit. (Examples below)
A word on types and keywords
Most often, highlighting every reserved keyword can make syntax difficult to read, defeating the purpose altogether. Therefore, three distinct rules are provided: dataTypes, standardKeywords and lesserKeywords. Each of these rules can be customized individually and come with a predefined list of most widely used T-SQL and SQL-92 keywords and data types. Furthermore each of this lists can be customized as described above.
Starting from v1.1.0 types and keywords are no longer uppercased by default. Custom styles should use the
casing: 'uppercase'
option for this behaviour. Predefined style already provides this option so no changes should be required.
All of the previous rule styles can be defined like this:
/* options = {"rule": style, ... } where
* style = { mode: "modifier", fg: "color", bg: "color"}
*/
const options = {
constants: {
mode: 'inverse',
fg: 'red',
bg: 'white'
},
...
};
Each style having an optional:
'bold'
'red'
'black'
These can be one of the following.
reset
bold
dim
italic
underline
blink
inverse
hidden
strikethrough
black
red
green
yellow
blue
magenta
cyan
white
⚠ Be advised
This feature is experimental and should be used with discretion. Custom pattern-matching has the potential to disrupt other rules and induce defects in highlighting.
You can define as many rules as needed. Like built-in rules, an optional style can be set for each one. Every rule can be named as desired, simple names are encouraged to avoid problems though. Option transform is not required, regexp is.
/(https?|ftp):\/\/[^\s/$.?#].[^\s]*/g
'hidden'
or match => match.trim()
/* Predifined style */
const defaults = {
comments: { mode: 'dim', fg: 'white' },
constants: { mode: 'dim', fg: 'red' },
delimitedIdentifiers: { mode: 'dim', fg: 'yellow' },
variables: { mode: 'dim', fg: 'magenta' },
dataTypes: { mode: 'dim', fg: 'green', casing: 'uppercase' },
standardKeywords: { mode: 'dim', fg: 'cyan', casing: 'uppercase' },
lesserKeywords: { mode: 'bold', fg: 'black', casing: 'uppercase' },
prefix: { replace: /.*?: / }
};
const igniculus = require('igniculus')(
{
constants: { mode: 'bold', fg: 'yellow' },
numbers: { mode: 'bold', fg: 'magenta' },
delimitedIdentifiers: { mode: 'bold', fg: 'red' },
standardKeywords: { mode: 'bold', fg: 'blue' }
}
);
igniculus("INSERT INTO [Printers] ([port], [name], [ready], [online], [check]) " +
"VALUES ('lp0', 'Bob Marley', 0, 1, 1)");
const igniculus = require('igniculus');
const options = {
delimitedIdentifiers: {
fg: 'yellow'
},
dataTypes: {
fg: 'magenta',
types: ['VARBINARY']
},
standardKeywords: {
fg: 'red',
keywords: ['CREATE', 'PRIMARY', 'KEY']
},
lesserKeywords: {
mode: 'bold',
fg: 'black',
keywords: ['TABLE', 'NOT', 'NULL']
},
prefix: {
text: '\n'
}
};
igniculus(options);
igniculus.log('CREATE TABLE User (' +
'[username] VARCHAR(20) NOT NULL, ' +
'[password] BINARY(64) NOT NULL, ' +
'[avatar] VARBINARY(MAX), PRIMARY KEY ([username]))');
const igniculus = require('igniculus');
const log = igniculus({
constants: { fg: 'red' },
delimitedIdentifiers: { mode: 'bold', fg: 'cyan' },
standardKeywords: { fg: 'blue', casing: 'uppercase' },
own: {
_: {
mode: 'bold',
fg: 'white',
regexp: /^/,
transform: '█ '
},
comments: {
regexp: /(-{2}.*)|(\/\*(.|[\r\n])*?\*\/)[\r\n]*/g,
transform: ''
},
UUIDv4s: {
mode: 'bold',
fg: 'black',
regexp: /'[A-F\d]{8}-[A-F\d]{4}-4[A-F\d]{3}-[89AB][A-F\d]{3}-[A-F\d]{12}'/gi,
transform: (uuid) => uuid.replace(/\w{1}/g, 'x')
}
}
});
log("/* May 13th, 2018 | 06:09:28.262 | http://server.local:8000 */" +
"select [username], [password] from Users where [_uuid] = '4072FA1B-D9E7-4F0E-9553-5F2CFFE6CC7A'");
Igniculus' logger is a drop in replacement on any tool that passes the log function either a string
or Object
paramater. In the latest case the toString()
method will be called to obtain a string
primitive.
Using igniculus with sequelize is straightforward.
const Sequelize = require('sequelize');
const igniculus = require('igniculus')();
const sequelize = new Sequelize('database', 'username', 'password', {
logging: igniculus
});
/* Or add some customizations */
const Sequelize = require('sequelize');
const igniculus = require('igniculus')(
{
constants: { fg: 'red' },
delimitedIdentifiers: { fg: 'yellow' },
dataTypes: { fg: 'red' },
standardKeywords: { fg: 'magenta' },
lesserKeywords: { mode: 'bold', fg: 'black' },
prefix: {
mode: 'bold',
fg: 'white',
replace: /.*?:/,
text: '(Sequelize)'
},
postfix: { text: '\r\n' }
}
);
const sequelize = new Sequelize('database', 'username', 'password', {
logging: igniculus
});
...
sequelize.sync({ logging: igniculus});
For a full list of changes please refer to the changelog.
1.5.0 ~ 24 Jun 2018
FAQs
SQL Syntax Highlighter and Logger. Unadorned and customizable.
The npm package igniculus receives a total of 24,402 weekly downloads. As such, igniculus popularity was classified as popular.
We found that igniculus demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
Security News
Popular npm packages like eslint-config-prettier were compromised after a phishing attack stole a maintainer’s token, spreading malicious updates.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.