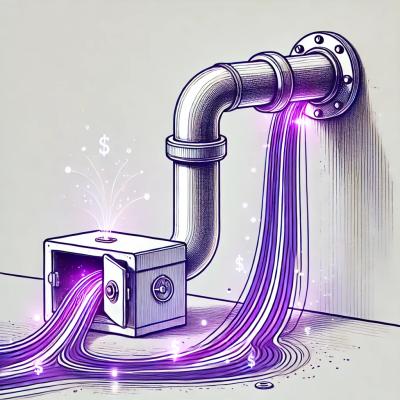
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
image-conversion
Advanced tools
A simple and easy-to-use JS image convert tools, which can specify size to compress the image.
image-conversion is a simple and easy-to-use JS image convert tools, which provides many methods to convert between Image,Canvas,File and dataURL.
In addition,image-conversion can specify size to compress the image (test here).
npm i image-conversion --save
# or
yarn add image-conversion
in browser:
<script src="https://cdn.jsdelivr.net/gh/WangYuLue/image-conversion/build/conversion.js"></script>
in CommonJS:
const imageConversion = require("image-conversion")
in ES6:
import * as imageConversion from 'image-conversion';
or
import {compress, compressAccurately} from 'image-conversion';
<input id="demo" type="file" onchange="view()">
function view(){
const file = document.getElementById('demo').files[0];
console.log(file);
imageConversion.compressAccurately(file,200).then(res=>{
//The res in the promise is a compressed Blob type (which can be treated as a File type) file;
console.log(res);
})
}
// or use an async function
async function view() {
const file = document.getElementById('demo').files[0];
console.log(file);
const res = await imageConversion.compressAccurately(file,200)
console.log(res);
}
function view(){
const file = document.getElementById('demo').files[0];
console.log(file);
imageConversion.compress(file,0.9).then(res=>{
console.log(res);
})
}
image-conversion
provides many methods to convert between Image,Canvas,File and dataURL,as follow:
imagetoCanvas(image[, config]) → {Promise(Canvas)}
Convert an image object into a canvas object.
Name | Type | Attributes | Description |
---|---|---|---|
image | image | a image object | |
config | object | optional | with this config you can zoom in, zoom out, and rotate the image |
imageConversion.imagetoCanvas(image);
//or
imageConversion.imagetoCanvas(image,{
width: 300, //result image's width
height: 200, //result image's height
orientation:2,//image rotation direction
scale: 0.5, //the zoom ratio relative to the original image, range 0-10;
//Setting config.scale will override the settings of
//config.width and config.height;
})
config.orientation
has many options to choose,as follow:
Options | Orientation |
---|---|
1 | 0° |
2 | horizontal flip |
3 | 180° |
4 | vertical flip |
5 | clockwise 90° + horizontal flip |
6 | clockwise 90° |
7 | clockwise 90° + vertical flip |
8 | Counterclockwise 90° |
Promise that contains canvas object.
dataURLtoFile(dataURL[, type]) → {Promise(Blob)}
Convert a dataURL string to a File(Blob) object. you can determine the type of the File object when transitioning.
Name | Type | Attributes | Description |
---|---|---|---|
dataURL | string | a dataURL string | |
type | string | optional | determine the converted image type; the options are "image/png", "image/jpeg", "image/gif". |
Promise that contains a Blob object.
compress(file, config) → {Promise(Blob)}
Compress a File(Blob) object.
Name | Type | Description |
---|---|---|
file | File(Blob) | a File(Blob) object |
config | number | object | if number type, range 0-1, indicate the image quality; if object type,you can pass parameters to the imagetoCanvas and dataURLtoFile method;Reference is as follow: |
If you compress png transparent images, please select 'image/png' type.
// number
imageConversion.compress(file,0.8)
//or
// object
imageConversion.compress(file,{
quality: 0.8,
type: "image/jpeg",
width: 300,
height: 200,
orientation:2,
scale: 0.5,
})
Promise that contains a Blob object.
compressAccurately(file, config) → {Promise(Blob)}
Compress a File(Blob) object based on size.
Name | Type | Description |
---|---|---|
file | File(Blob) | a File(Blob) object |
config | number | object | if number type, specify the size of the compressed image(unit KB); if object type,you can pass parameters to the imagetoCanvas and dataURLtoFile method;Reference is as follow: |
If you compress png transparent images, please select 'image/png' type
// number
imageConversion.compressAccurately(file,100); //The compressed image size is 100kb
// object
imageConversion.compressAccurately(file,{
size: 100, //The compressed image size is 100kb
accuracy: 0.9,//the accuracy of image compression size,range 0.8-0.99,default 0.95;
//this means if the picture size is set to 1000Kb and the
//accuracy is 0.9, the image with the compression result
//of 900Kb-1100Kb is considered acceptable;
type: "image/jpeg",
width: 300,
height: 200,
orientation:2,
scale: 0.5,
})
Promise that contains a Blob object.
canvastoDataURL(canvas[, quality, type]) → {Promise(string)}
Convert a Canvas object into a dataURL string, this method can be compressed.
Name | Type | Attributes | Description |
---|---|---|---|
canvas | canvas | a Canvas object | |
quality | number | optional | range 0-1, indicate the image quality, default 0.92 |
type | string | optional | determine the converted image type; the options are "image/png", "image/jpeg", "image/gif",default "image/jpeg" |
Promise that contains a dataURL string.
canvastoFile(canvas[, quality, type]) → {Promise(Blob)}
Convert a Canvas object into a Blob object, this method can be compressed.
Name | Type | Attributes | Description |
---|---|---|---|
canvas | canvas | a Canvas object | |
quality | number | optional | range 0-1, indicate the image quality, default 0.92 |
type | string | optional | determine the converted image type; the options are "image/png", "image/jpeg", "image/gif",default "image/jpeg" |
Promise that contains a Blob object.
dataURLtoImage(dataURL) → {Promise(Image)}
Convert a dataURL string to a image object.
Name | Type | Description |
---|---|---|
dataURL | string | a dataURL string |
Promise that contains a Image object.
downloadFile(file[, fileName])
Download the image to local.
Name | Type | Attributes | Description |
---|---|---|---|
file | File(Blob) | a File(Blob) object | |
fileName | string | optional | download file name, if none, timestamp named file |
filetoDataURL(file) → {Promise(string)}
Convert a File(Blob) object to a dataURL string.
Name | Type | Description |
---|---|---|
file | File(Blob) | a File(Blob) object |
Promise that contains a dataURL string.
urltoBlob(url) → {Promise(Blob)}
Load the required Blob object through the image url.
Name | Type | Description |
---|---|---|
url | string | image url |
Promise that contains a Blob object.
urltoImage(url) → {Promise(Image)}
Load the required Image object through the image url.
Name | Type | Description |
---|---|---|
url | string | image url |
Promise that contains Image object.
FAQs
A simple and easy-to-use JS image convert tools, which can specify size to compress the image.
The npm package image-conversion receives a total of 6,311 weekly downloads. As such, image-conversion popularity was classified as popular.
We found that image-conversion demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.