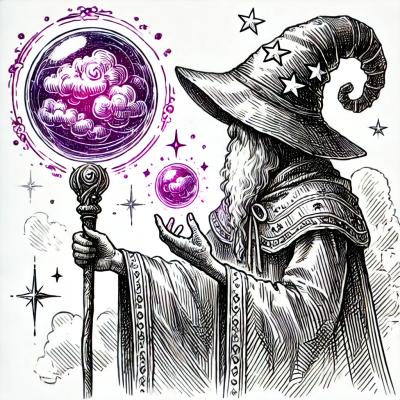
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
imgbb-uploader
Advanced tools
Node >= 8 recommended ( this module uses async/await )
npm install imgbb-uploader
var imgbbUploader = require('imgbb-uploader');
imgbbUploader("your-imgbb-api-key-string", "home/absolute/path/to/your/image/image.png")
.then(response => console.log(response))
.catch(error => console.log(error))
.then(response => console.log(response))
output example :{
id: '5jKj6XV',
url_viewer: 'https://ibb.co/5jKj6XV',
url: 'https://i.ibb.co/94Z4Nmj/test.jpg',
display_url: 'https://i.ibb.co/94Z4Nmj/test.jpg',
title: 'test',
time: '1574431312',
image: {
filename: 'test.jpg',
name: 'test',
mime: 'image/jpeg',
extension: 'jpg',
url: 'https://i.ibb.co/94Z4Nmj/test.jpg',
size: 91264
},
thumb: {
filename: 'test.jpg',
name: 'test',
mime: 'image/jpeg',
extension: 'jpg',
url: 'https://i.ibb.co/5jKj6XV/test.jpg',
size: '12875'
},
delete_url: 'https://ibb.co/5jKj6XV/ffd8ef0b1c803f02443553535cf4a5f4'
}
This function returns a promise, this is why :
console.log(imgbbUploader(myKey, myPath)) // output : Promise { <pending> }
Your data is available in the .then(response => response)
as shown above.
This module doesn't support array uploads and other fancy stuff. For heavy duty uploads, you'll probably have to work with fs.readdir and forEach methods ; in that case you may also be interested in path.
For example, you can create a baseDir.js
file wherever it suits you:
// baseDir.js
const path = require('path');
const dirPath = path.join(__dirname);
module.exports = dirPath;
Then you can require this file elsewhere, and use something like path.join(myDirpath, "subfolder", "subsubfolder")
to dig into directories programmatically. Once you're there you can fs.readdir
and iterate forEach
file of that directory, then do whatever you want.
That is just a simple example, see fs
documentation and Stack Overflow for more inspiration.
FAQs
Lightweight module to upload images through imgBB and other chevereto-based APIs.
The npm package imgbb-uploader receives a total of 4,311 weekly downloads. As such, imgbb-uploader popularity was classified as popular.
We found that imgbb-uploader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.