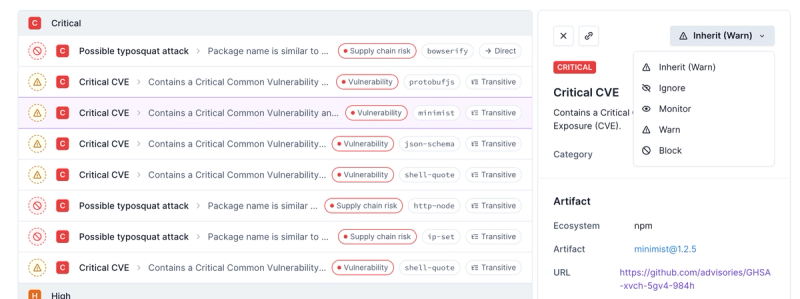
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
imgbb-uploader
Advanced tools
Changelog
1.3.5
Readme
Lightweight Nodejs module to upload local pictures files to imgbb API and get display URLs in response.
Primary use is letting imgBB handle the hosting & serving of images.
Node >= 8 ( async/await )
Care: this module uses fs
under the hood. It WON'T work outside the node environment !
I want to use this client-side
Formats supported by ImgBB API: .jpg
, .png
,.bmp
,.gif
, base64
, url
.
npm install imgbb-uploader
const imgbbUploader = require("imgbb-uploader");
imgbbUploader("your-imgbb-api-key-string", "path/to/your/image.png")
.then((response) => console.log(response))
.catch((error) => console.error(error));
.then((response) => console.log(response))
output example :{
id: '26Sy9tM',
title: '5e7599f65f27',
url_viewer: 'https://ibb.co/26Sy9tM',
url: 'https://i.ibb.co/z5FrMR2/5e7599f65f27.png',
display_url: 'https://i.ibb.co/z5FrMR2/5e7599f65f27.png',
size: 260258,
time: '1609336605',
expiration: '0',
image: {
filename: '5e7599f65f27.png',
name: '5e7599f65f27',
mime: 'image/png',
extension: 'png',
url: 'https://i.ibb.co/z5FrMR2/5e7599f65f27.png'
},
thumb: {
filename: '5e7599f65f27.png',
name: '5e7599f65f27',
mime: 'image/png',
extension: 'png',
url: 'https://i.ibb.co/26Sy9tM/5e7599f65f27.png'
},
medium: {
filename: '5e7599f65f27.png',
name: '5e7599f65f27',
mime: 'image/png',
extension: 'png',
url: 'https://i.ibb.co/14kK0tt/5e7599f65f27.png'
},
delete_url: 'https://ibb.co/26Sy9tM/087a7edaaac26e1c940283df07d0b1d7'
}
This async function returns a promise:
console.log(imgbbUploader(myKey, myPath)) // Promise { <pending> }
Use await
or .then
as shown above.
Note about imgBB API: the medium
Object will only be returned for .png
and base64
files !
From version 1.2.0 onward, you can also pass an options object as param.
Use it to customize filename and/or a set duration after which the image will be deleted, cf their docs.
The key you'll use for your image depends on its nature. One of these must be defined:
imagePath
in case of a local fileimageUrl
in case of an URL stringbase64string
in case of base64-encoded imageconst imgbbUploader = require("imgbb-uploader");
const options = {
apiKey: process.env.IMGBB_API_KEY, // MANDATORY
imagePath: "./your/image/path", // OPTIONAL: pass a local file (max 32Mb)
name: "yourCustomFilename", // OPTIONAL: pass a custom filename to imgBB API
expiration: 3600 /* OPTIONAL: pass a numeric value in seconds.
It must be in the 60-15552000 range (POSIX time ftw).
Enable this to force your image to be deleted after that time. */,
imageUrl: "https://placekitten.com/500/500", // OPTIONAL: pass an URL to imgBB (max 32Mb)
base64string:
"iVBORw0KGgoAAAANSUhEUgAAAAIAAAACCAYAAABytg0kAAAAEklEQVR42mNcLVNbzwAEjDAGACcSA4kB6ARiAAAAAElFTkSuQmCC",
/* OPTIONAL: pass base64-encoded image (max 32Mb)
Enable this to upload base64-encoded image directly as string. (available from 1.3.0 onward)
Allows to work with RAM directly for increased performance (skips fs I/O calls).
Beware: options.imagePath will be ignored as long as options.base64string is defined!
*/
};
imgbbUploader(options)
.then((response) => console.log(response))
.catch((error) => console.error(error));
This module is tiny & totally unlicensed: to better fit your need, please fork away !
Basic instructions for tweaking
Using options.base64string:
const imgbbUploader = require("imgbb-uploader");
// Some promise of base64 data
const base64str = () =>
new Promise((resolve) => {
return setTimeout(() => {
resolve(
"iVBORw0KGgoAAAANSUhEUgAAAAIAAAACCAYAAABytg0kAAAAEklEQVR42mNcLVNbzwAEjDAGACcSA4kB6ARiAAAAAElFTkSuQmCC",
);
}, 1000);
});
// Your barebone async function
const myUrl = async (name) => {
return await imgbbUploader({
apiKey: "definitely-not-a-valid-key",
base64string: await base64str(),
name: name,
})
.then((res) => {
console.log(`Handle success: ${res.url}`);
return res.url;
})
.catch((e) => {
console.error(`Handle error: ${e}`);
return "http://placekitten.com/300/300";
});
};
myUrl("Dolunay_Obruk-Sama_<3");
This module doesn't support array uploads. For heavy duty, you'll probably have to work with fs.readdir and maybe async forEach. For example, you can create a baseDir.js
file wherever it suits you:
module.exports = require("path").join(__dirname);
Then you can require it elsewhere and use something like path.join(myDirpath, "subfolder")
to dig into directories programmatically.
Once there you can for example fs.readdir
and iterate forEach
file of that directory.
See fs
documentation and Stack Overflow for more inspiration on the matter.
FAQs
Lightweight module to upload images through imgBB and other chevereto-based APIs.
The npm package imgbb-uploader receives a total of 4,410 weekly downloads. As such, imgbb-uploader popularity was classified as popular.
We found that imgbb-uploader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.