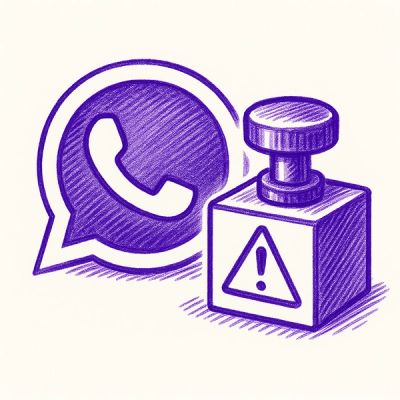
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
immutable-form
Advanced tools
[](https://www.npmjs.com/package/immutable-form) [](https://circleci.com/gh/Intelight/immutable-form)
Install the package
yarn install immutable-form
To install the latest development version
yarn install immutable-form@devel
The intent of this package is to provide a simple to use implementation of managing form state for React components, streamlining common requirements such as validation, error handling, data fetching and submission.
This package consists of three modules, Form
, FormCollection
, and connectForm
.
Form
is a class which provides an API to read and write form's state. The state is stored within a Immutable object which in turn is resides within a Redux store.
FormCollection
holds references to all existing forms. This is useful if you're trying to access form values across different components.
connectForm
is a React higher order component which passes down form
and field
props into your components and re-renders on the form's state changes.
import { Form, connectForm } from 'immutable-form';
// This is just a mock promise for the sake of this code example, in reality this promise should originate from performing an async call to some api.
const createUserPromise = Promise.resolve({
userId: '123',
});
// These props could be anything. This function returns a new Form as a function of props.
const mapPropsToForm = ({
hasUsername,
hasPassword,
}) => new Form('userForm', {
fields: {
username: (hasUsername || undefined) && {
// All these fields are optional,
value: 'some value', // Set an initial value
// Any validation function in validate which returns a string will cause a validation error. Each validation function receives the field value and the form reference as parameters.
validate: [
username => username.trim().length === 0 && 'Username cannot be be empty',
],
},
password: (hasPassword || undefined) && {
validate: [
password => password.trim().length < 6 && 'Password must be longer than 6 characters',
],
},
},
})
// Send a request to the server
.setSubmit((form) => createUserPromise)
// If the promise resolves, do something with the results
.setOnSuccess(({userId}, form) => userId)
// If the promise is rejected, do something.
.setOnFailure((err, form) => err);
const UserForm = ({
// fields is a an Immutable Map
fields,
// This is the `Form` object, you can use it do extra actions such submitting the form.
form,
}) => (
<div>
<input
type="text"
onChange={(e) => form.setField('username', e.target.value)}
value={fields.getIn(['username', 'value'])}
/>
{fields.getIn(['username', 'errors'].first())}
<input
type="text"
onChange={(e) => form.setField('password', e.target.value)}
value={fields.getIn(['password', 'value'])}
/>
{fields.getIn(['password', 'errors'].first())}
<button onClick={() => form.submit()} />
</div>
);
export default connectForm(mapPropsToForm)(UserForm);
import { Form, connectForm } from 'immutable-form';
// This is just a mock promise for the sake of this code example, in reality this promise should originate from performing an async call to some api.
const loadFormPromise = Promise.resolve({
username: 'someusername',
password: 'somepassword'
});
// Note that the below example does not use a function to create a new Form. See above for that use case.
const userForm = new Form('userForm', {
fields: {
username: {
validate: [
username => username.trim().length === 0 && 'Username cannot be be empty',
],
},
password: {
validate: [
password => password.trim().length < 6 && 'Password must be longer than 6 characters',
],
},
},
})
.setLoad(form => loadFormPromise.then(({ username, password }) => {
// When the promise resolves with the requested data
// you need to manually wire it into the form.
form.setField('username', username);
form.setField('email', email);
// To set the loaded data as the form's initial state
form.saveInitialState();
// This allows you call form.reset() and reset to the loaded values instead of an empty form.
}));
If using connectForm
the load promise will be called automatically in the component's lifecycle. To trigger a manual load, call form.load()
.
import { Form, connectForm } from 'immutable-form';
const Form = new Form('form', {}, {
logger: true, // Will enable redux-logger (false by default)
addToFormCollection: true, // Refrains from adding a reference to FormCollection, (true by default)
});
FAQs
[](https://www.npmjs.com/package/immutable-form) [](https://circleci.com/gh/Intelight/immutable-form)
The npm package immutable-form receives a total of 40 weekly downloads. As such, immutable-form popularity was classified as not popular.
We found that immutable-form demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.