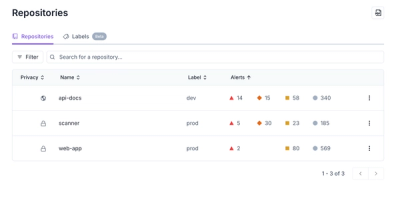
Product
Redesigned Repositories Page: A Faster Way to Prioritize Security Risk
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
ink-select-input
Advanced tools
The ink-select-input package is a React component for Ink, a React renderer for the terminal. It allows developers to create interactive command-line interfaces with selectable input options.
Basic Select Input
This feature allows you to create a basic select input component where users can navigate through options using arrow keys and select an option by pressing Enter.
const { h, render, Component } = require('ink');
const SelectInput = require('ink-select-input').default;
class App extends Component {
handleSelect = item => {
console.log(item.label);
};
render() {
const items = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Option 3', value: 'option3' }
];
return <SelectInput items={items} onSelect={this.handleSelect} />;
}
}
render(<App />);
Customizing Item Rendering
This feature allows you to customize how each item in the select input is rendered. In this example, a custom render function is used to add a '>' symbol next to the selected item.
const { h, render, Component } = require('ink');
const SelectInput = require('ink-select-input').default;
class App extends Component {
handleSelect = item => {
console.log(item.label);
};
renderItem = (item, { isSelected }) => (
<div>
{isSelected ? '>' : ' '} {item.label}
</div>
);
render() {
const items = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Option 3', value: 'option3' }
];
return <SelectInput items={items} onSelect={this.handleSelect} itemComponent={this.renderItem} />;
}
}
render(<App />);
Handling Input Change
This feature allows you to handle changes in the highlighted item. The onHighlight prop is used to execute a function whenever the highlighted item changes.
const { h, render, Component } = require('ink');
const SelectInput = require('ink-select-input').default;
class App extends Component {
handleSelect = item => {
console.log(item.label);
};
handleChange = item => {
console.log('Changed to:', item.label);
};
render() {
const items = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Option 3', value: 'option3' }
];
return <SelectInput items={items} onSelect={this.handleSelect} onHighlight={this.handleChange} />;
}
}
render(<App />);
Ink is a React renderer for the terminal. It allows you to build and render command-line applications using React components. While ink-select-input is a specific component for creating selectable inputs, Ink provides the foundational framework for building the entire CLI application.
Inquirer is a collection of common interactive command-line user interfaces. It provides a variety of prompts, including list, checkbox, and input prompts. Compared to ink-select-input, Inquirer offers a broader range of prompt types but is not built on React.
Blessed is a curses-like library for building terminal user interfaces. It provides a wide range of widgets and is highly customizable. Unlike ink-select-input, which is a React component, Blessed is a more general-purpose library for building terminal UIs.
Select input component for Ink
npm install ink-select-input
import React from 'react';
import {render} from 'ink';
import SelectInput from 'ink-select-input';
const Demo = () => {
const handleSelect = item => {
// `item` = { label: 'First', value: 'first' }
};
const items = [
{
label: 'First',
value: 'first'
},
{
label: 'Second',
value: 'second'
},
{
label: 'Third',
value: 'third'
}
];
return <SelectInput items={items} onSelect={handleSelect} />;
};
render(<Demo />);
Type: array
Default: []
Items to display in a list. Each item must be an object and have label
and value
props, it may also optionally have a key
prop.
If no key
prop is provided, value
will be used as the item key.
Type: boolean
Default: true
Listen to user's input. Useful in case there are multiple input components at the same time and input must be "routed" to a specific component.
Type: number
Default: 0
Index of initially-selected item in items
array.
Type: number
Number of items to display.
Type: Component
Custom component to override the default indicator component.
Type: Component
Custom component to override the default item component.
Type: function
Function to call when user selects an item. Item object is passed to that function as an argument.
Type: function
Function to call when user highlights an item. Item object is passed to that function as an argument.
FAQs
Select input component for Ink
The npm package ink-select-input receives a total of 204,750 weekly downloads. As such, ink-select-input popularity was classified as popular.
We found that ink-select-input demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
Security News
Multiple deserialization flaws in PyTorch Lightning could allow remote code execution when loading untrusted model files, affecting versions up to 2.4.0.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.