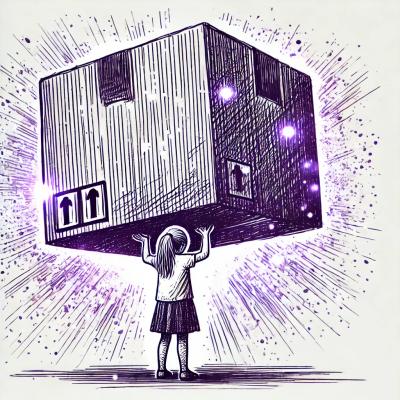
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
instagram-client
Advanced tools
Endpoint
Client.comments
// example
Client.comments.create("MEDIAID", {
text: "some comment",
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Endpoint
Client.likes
// example
Client.likes.getByMedia("MEDIAID", {
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Endpoint
Client.locations
// example
Client.locations.getByID("LOCATIONID", {
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Endpoint
Client.media
// example
Client.media.getByID("SOMEMEDIAID", {
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Endpoint
Client.oauth
// example
Client.oauth.getAccessToken({
grantType: "",
redirectURI: "",
code: "",
})
.then(result => {})
Endpoint
Client.subscriptions
// example
Client.subscriptions.create({
object: "",
aspect: "",
verifyToken: "",
callbackURL: "",
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Endpoint
Client.tags
// example
Client.tags.getByName("travel", {
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Endpoint
Client.users
// example
Client.users.getSelf({
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
InstagramClient
InstagramClient V1
const Instagram = require("instragram-client").v1
const Client = new Instagram({
CLIENT_ID: "SOMECLIENTID",
CLIENT_SECRET: "SOMECLIENTSECRET",
})
const Client = new Instagram({
CLIENT_ID: "SOMECLIENTID",
CLIENT_SECRET: "SOMECLIENTSECRET",
})
Endpoint
Client.comments
// example
Client.comments.create("MEDIAID", {
text: "some comment",
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Kind: global class
Extends: Endpoint
Creates a comment for a media by media id
Kind: instance method of Comments
Param | Type | Description |
---|---|---|
id | string | the media ID |
opts | object | the options object { accessToken, sign, text } |
cb | function | callback called if paseed, otherwise returns a promise |
Deletes a comment for a media by media id
Kind: instance method of Comments
Param | Type | Description |
---|---|---|
id | string | the media ID |
opts | object | the options object { accessToken, sign, text } |
cb | function | callback called if paseed, otherwise returns a promise |
Get comments for a media by media id
Kind: instance method of Comments
Param | Type | Description |
---|---|---|
id | string | the media ID |
opts | object | the options object { accessToken, sign, text } |
cb | function | callback called if paseed, otherwise returns a promise |
Endpoint
Client.likes
// example
Client.likes.getByMedia("MEDIAID", {
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Kind: global class
Extends: Endpoint
Gets likes for a media by media id
Kind: instance method of Likes
Param | Type | Description |
---|---|---|
id | string | the media ID |
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Like a media by media id with user from accessToken
Kind: instance method of Likes
Param | Type | Description |
---|---|---|
id | string | the media ID |
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Unlike a media by media id with user from accessToken
Kind: instance method of Likes
Param | Type | Description |
---|---|---|
id | string | the media ID |
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Endpoint
Client.locations
// example
Client.locations.getByID("LOCATIONID", {
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Kind: global class
Extends: Endpoint
Gets a location by id
Kind: instance method of Locations
Param | Type | Description |
---|---|---|
id | string | the location ID |
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets recent media for location by location id
Kind: instance method of Locations
Param | Type | Description |
---|---|---|
id | string | the location ID |
opts | object | the options object { accessToken, sign, maxTagID, minTagID } |
cb | function | callback called if paseed, otherwise returns a promise |
Search locations
Kind: instance method of Locations
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign, lat, lng, facebookPlacesID } |
cb | function | callback called if paseed, otherwise returns a promise |
Endpoint
Client.media
// example
Client.media.getByID("SOMEMEDIAID", {
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Kind: global class
Extends: Endpoint
Gets a media by id
Kind: instance method of Media
Param | Type | Description |
---|---|---|
id | string | the media ID |
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets a media by shortcode
Kind: instance method of Media
Param | Type | Description |
---|---|---|
id | string | the media shortcode |
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Search media by locations
Kind: instance method of Media
Param | Type | Description |
---|---|---|
id | string | the media shortcode |
opts | object | the options object { accessToken, sign, lng, lat, distance } |
cb | function | callback called if paseed, otherwise returns a promise |
Endpoint
Client.oauth
// example
Client.oauth.getAccessToken({
grantType: "",
redirectURI: "",
code: "",
})
.then(result => {})
Kind: global class
Extends: Endpoint
Endpoint
It returns the accessToken
Kind: instance method of OAuth
Param | Type | Description |
---|---|---|
opts | object | the options object { code, grantType, redirectURI } |
cb | function | callback called if paseed, otherwise returns a promise |
It returns the authURL
Kind: instance method of OAuth
Param | Type | Description |
---|---|---|
opts | object | the options object { CLIENT_ID, CLIENT_SECRET, responseType, redirectURI, scope } |
Endpoint
Client.subscriptions
// example
Client.subscriptions.create({
object: "",
aspect: "",
verifyToken: "",
callbackURL: "",
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Kind: global class
Extends: Endpoint
Creates a subscription
Kind: instance method of Subscriptions
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign, object, aspect, verifyToken, callbackURL } |
cb | function | callback called if paseed, otherwise returns a promise |
Lists the subscriptions for the client
Kind: instance method of Subscriptions
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign, object, aspect, verifyToken, callbackURL } |
cb | function | callback called if paseed, otherwise returns a promise |
Deletes subscriptions for the client
Kind: instance method of Subscriptions
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign, object, aspect, verifyToken, callbackURL } |
cb | function | callback called if paseed, otherwise returns a promise |
Endpoint
Client.tags
// example
Client.tags.getByName("travel", {
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Kind: global class
Extends: Endpoint
Endpoint
Gets a tag by name
Kind: instance method of Tags
Param | Type | Description |
---|---|---|
tagName | string | the tag name |
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets a tag's recent media by tag name
Kind: instance method of Tags
Param | Type | Description |
---|---|---|
tagName | string | the tag name |
opts | object | the options object { accessToken, sign, minTagID, maxTaxID, count } |
cb | function | callback called if paseed, otherwise returns a promise |
Searches a tag by tag name
Kind: instance method of Tags
Param | Type | Description |
---|---|---|
tagName | string | the tag name |
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Endpoint
Client.users
// example
Client.users.getSelf({
accessToken: "SOMEACCESSTOKEN",
})
.then(result => {})
Kind: global class
Extends: Endpoint
Endpoint
Gets the user from the access token
Kind: instance method of Users
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets the users followed by the user from the access token
Kind: instance method of Users
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets the user's follower of the user from the access token
Kind: instance method of Users
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets the pending follow requests of the user from the access token
Kind: instance method of Users
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets the relationship between a user and the user from the access token
Kind: instance method of Users
Param | Type | Description |
---|---|---|
id | string | the ID of the user to check the relatioship with the accessToken user |
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Updates the relationship between a user and the user from the access token
Kind: instance method of Users
Param | Type | Description |
---|---|---|
id | string | the ID of the user to check the relatioship with the accessToken user |
opts | object | the options object { accessToken, sign, action } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets a user by ID
Kind: instance method of Users
Param | Type | Description |
---|---|---|
id | string | the ID of the user to get |
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets a user's recent media by user ID
Kind: instance method of Users
Param | Type | Description |
---|---|---|
id | string | the ID of the user to get |
opts | object | the options object { accessToken, sign, count } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets a self recent media from the accessToken
Kind: instance method of Users
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Gets a self media liked from the accessToken
Kind: instance method of Users
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign } |
cb | function | callback called if paseed, otherwise returns a promise |
Search a users
Kind: instance method of Users
Param | Type | Description |
---|---|---|
opts | object | the options object { accessToken, sign, q } |
cb | function | callback called if paseed, otherwise returns a promise |
InstagramClient
InstagramClient V1
const Instagram = require("instragram-client").v1
const Client = new Instagram({
CLIENT_ID: "SOMECLIENTID",
CLIENT_SECRET: "SOMECLIENTSECRET",
})
Kind: global class
Extends: InstagramClient
InstagramClient
Param | Type | Description |
---|---|---|
opts | object | the options object { CLIENT_ID, CLIENT_SECRET } |
Kind: instance method of V1
Param | Type |
---|---|
endpoint | string |
opts | object |
cb | function |
Kind: instance method of V1
Param | Type |
---|---|
endpoint | string |
opts | object |
formData | object |
cb | function |
Kind: instance method of V1
Param | Type |
---|---|
endpoint | string |
opts | object |
formData | object |
cb | function |
FAQs
Instagram API client for node, supports both promises and callbacks
The npm package instagram-client receives a total of 0 weekly downloads. As such, instagram-client popularity was classified as not popular.
We found that instagram-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.