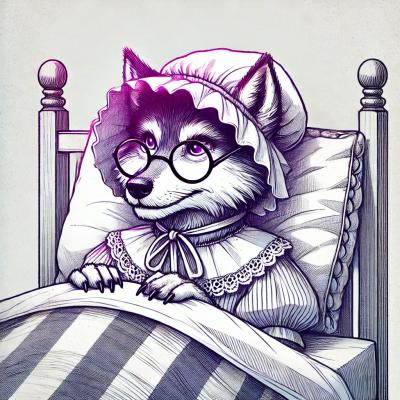
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
instantsearch.js
Advanced tools
instantsearch.js is a library for building performant and instant search experiences with Algolia. It provides a set of widgets and tools to create highly customizable search interfaces.
Search Box
The search box widget allows users to input their search queries. It connects to the Algolia index and fetches results based on the user's input.
const search = instantsearch({
indexName: 'instant_search',
searchClient: algoliasearch('YourApplicationID', 'YourSearchOnlyAPIKey')
});
search.addWidget(
instantsearch.widgets.searchBox({
container: '#searchbox'
})
);
search.start();
Hits
The hits widget displays the search results. It can be customized to show different attributes of the search results and highlight the matching parts of the query.
search.addWidget(
instantsearch.widgets.hits({
container: '#hits',
templates: {
item: '<div>{{#helpers.highlight}}{ "attribute": "name" }{{/helpers.highlight}}</div>'
}
})
);
Refinement List
The refinement list widget allows users to filter search results based on specific attributes, such as categories. It provides checkboxes for each attribute value.
search.addWidget(
instantsearch.widgets.refinementList({
container: '#refinement-list',
attribute: 'categories'
})
);
Pagination
The pagination widget enables users to navigate through pages of search results. It provides controls for moving to the next or previous page and jumping to specific pages.
search.addWidget(
instantsearch.widgets.pagination({
container: '#pagination'
})
);
react-instantsearch is a library for building Algolia search interfaces in React. It provides React components that wrap instantsearch.js widgets, making it easier to integrate with React applications. Compared to instantsearch.js, it is more suitable for React-based projects.
searchkit is a suite of UI components built in React to create search experiences powered by Elasticsearch. It offers a similar set of features to instantsearch.js but is designed to work with Elasticsearch instead of Algolia.
Instant search for everyone, even for your cat 😸.
API is unstable. We welcome any idea.
npm install instantsearch.js --save-dev
<script>
instantsearch.js is available on jsDelivr:
<script src="//cdn.jsdelivr.net/instantsearch.js/0/instantsearch.min.js"></script>
var instantsearch = require('instantsearch.js');
var search = instantsearch({
appId: appId, // Mandatory
apiKey: apiKey, // Mandatory
indexName: indexName, // Mandatory
numberLocale: 'fr-FR' // Optional, defaults to 'en-EN'
});
// add a widget
search.addWidget(
instantsearch.widgets.searchBox({
container: '#search-box',
placeholder: 'Search for libraries in France...'
})
);
// start
search.start();
function mySuperWidget(opts) {
return {
getConfiguration: function(searchParameters) {
return {
// helper params
}
},
init: function(initialState, helper) {
// helper: see http://algolia.github.io/algoliasearch-helper-js/docs/
// called before first `helper.on('result');`
},
render: function(results, state, helper) {
// content: see http://algolia.github.io/algoliasearch-helper-js/docs/SearchResults.html
// helper: see http://algolia.github.io/algoliasearch-helper-js/docs/
// called at each `helper.on('result')`
}
}
}
search.addWidget(mySuperWidget());
Most of the widgets accept a template
or templates
option that let you
change the default rendering.
template
can be defined either as a Mustache (Hogan) string or as a function.
See the documentation of each widget to see which data is passed to the
template.
// Mustache template example
search.addWidget(
instantsearch.widgets.stats({
container: '#stats',
template: '<div>You have {{nbHits}} results, fetched in {{processingTimeMS}}ms.</div>'
})
);
// Function template example
search.addWidget(
instantsearch.widgets.stats({
container: '#stats',
template: function(data) {
return '<div>You have ' + data.nbHits + 'results, fetched in ' + data.processingTimMS +'ms.</div>'
}
})
);
In order to help you when defining your templates, instantsearch.js
exposes
a few helpers. All helpers are accessible in the Mustache templating through
{{#helpers.nameOfTheHelper}}{{valueToFormat}}{{/helpers.nameOfTheHelper}}
. To
use them in the function templates, you'll have to call
search.templateHelpers.nameOfTheHelper
where search
is your current
instantsearch
instance.
Here is the list of the currently available helpers.
formatNumber
: Will accept a number as input and returned the formatted
version of the number in the locale defined with the numberLocale
config
option (defaults to en-EN
).
eg. 100000
will be formatted as 100 000
with en-EN
Here is the development workflow:
npm run dev
# open http://localhost:8080
# make changes in your widgets, or in example/app.js
npm test # test and lint
npm run test:watch # developer mode, test only
npm run test:coverage
<div id="search-box"></div>
search.addWidget(
instantsearch.widgets.searchBox({
container: '#search-box',
placeholder: 'Search for products',
// cssClass
})
);
<div id="stats"></div>
search.addWidget(
instantsearch.widgets.stats({
container: '#stats',
template: // mustache string or function(stats) with the following keys
// nbHits: number,
// hasNoResults: boolean
// hasOneResult: boolean
// hasManyResults: boolean
// processingTimeMS: number
})
);
This widget will let you change the current index being targeted. This is especially useful for changing the current sort order. If you need your results ordered following a special rule (like price ascending or price descending), you'll need several indices. This widget lets you easily change it.
<div id="index-selector"></div>
search.addWidget(
instantsearch.widgets.indexSelector({
container: '#index-selector',
indices: [
{name: 'instant_search', label: 'Most relevant'},
{name: 'instant_search_price_asc', label: 'Lowest price'},
{name: 'instant_search_price_desc', label: 'Highest price'}
],
cssClass: 'form-control'
})
);
/**
* Instantiate a dropdown element to choose the current targeted index
* @param {String|DOMElement} options.container Valid CSS Selector as a string or DOMElement
* @param {Array} options.indices Array of objects defining the different indices to choose from. Each object must contain a `name` and `label` key.
* @param {String} [options.cssClass] Class name(s) to be added to the generated select element
* @return {Object}
*/
<div id="pagination"></div>
search.addWidget(
instantsearch.widgets.pagination({
container: '#pagination',
// cssClass, // add cssClasses to the main wrapper
// padding: 3, // number of page numbers to show before/after current
// showFirstLast: true, // show or hide first and last links
// maxPages, // automatically computed based on the result set
// labels: {
// prev: '‹', // ‹
// next: '›', // ›
// first: '«', // «
// last: '»' // »
// }
})
);
<div id="hits"></div>
search.addWidget(
instantsearch.widgets.hits({
container: '#hits',
templates: {
empty, // string (mustache format) or function(hit) return string
hit // string (mustache format) or function(hit) return string
},
hitsPerPage: 20,
// cssClass
})
);
This widget is used to add filtering of results on a boolean value. Let's say
you want to only display elements that are eligible to free shipping. You'll
just have to instantiate this widget with a facetName
of free_shipping
(with
free_shipping
being a boolean attribute in your records.
When toggling on this widget, only hits with Free Shipping will be displayed. When switching it off, all items will be displayed.
Note that we are not toggling from true
to false
here, but from true
to
undefined
.
<div id="free_shipping"></div>
search.addWidget(
instantsearch.widgets.toggle({
container: '#free_shipping',
facetName: 'free_shipping',
label: 'Free Shipping',
template: '<label><input type="checkbox" {{#isRefined}}checked{{/isRefined}} />{{label}}</label>'
})
);
/**
* Instantiate the toggling of a boolean facet filter on and off.
* Note that it will not toggle between `true` and `false, but between `true`
* and `undefined`.
* @param {String|DOMElement} options.container Valid CSS Selector as a string or DOMElement
* @param {String} options.facetName Name of the attribute for faceting (eg. "free_shipping")
* @param {String} options.label Human-readable name of the filter (eg. "Free Shipping")
* @param {String|Function} [options.template] Item template, provided with `label` and `isRefined`
* @return {Object}
*/
/**
* Instantiate a list of refinements based on a facet
* @param {String|DOMElement} options.container Valid CSS Selector as a string or DOMElement
* @param {String} options.facetName Name of the attribute for faceting
* @param {String} options.operator How to apply refinements. Possible values: `or`, `and`
* @param {String[]} [options.sortBy=['count:desc']] How to sort refinements. Possible values: `count|isRefined|name:asc|desc`
* @param {String} [options.limit=100] How much facet values to get.
* @param {String|String[]} [options.rootClass=null] CSS class(es) for the root `<ul>` element
* @param {String|String[]} [options.itemClass=null] CSS class(es) for the item `<li>` element
* @param {String|Function} [options.template] Item template, provided with `name`, `count`, `isRefined`
* @param {String|Function} [options.singleRefine=true] Are multiple refinements allowed or only one at the same time. You can use this
* to build radio based refinement lists for example.
* @return {Object}
*/
<div id="brands"></div>
search.addWidget(
instantsearch.widgets.refinementList({
container: '#brands',
facetName: 'brands',
operator: 'or'
})
);
/**
* Create a menu out of a facet
* @param {String|DOMElement} options.container Valid CSS Selector as a string or DOMElement
* @param {String} options.facetName Name of the attribute for faceting
* @param {String[]} [options.sortBy=['count:desc']] How to sort refinements. Possible values: `count|isRefined|name:asc|desc`
* @param {String} [options.limit=100] How much facet values to get.
* @param {String|String[]} [options.rootClass=null] CSS class(es) for the root `<ul>` element
* @param {String|String[]} [options.itemClass=null] CSS class(es) for the item `<li>` element
* @param {String|Function} [options.template] Item template, provided with `name`, `count`, `isRefined`
* @return {Object}
*/
<div id="categories"></div>
search.addWidget(
instantsearch.widgets.menu({
container: '#categories',
facetName: 'categories'
})
);
/**
* Instantiate a slider based on a numeric attribute
* @param {String|DOMElement} options.container Valid CSS Selector as a string or DOMElement
* @param {String} options.facetName Name of the attribute for faceting
* @param {Boolean|Object} [options.tooltips=true] Should we show tooltips or not.
* The default tooltip will show the formatted corresponding value without any other token.
* You can also provide
* tooltips: {format: function(formattedValue, rawValue) {return '$' + formattedValue}}
* So that you can format the tooltip display value as you want
* @return {Object}
*/
<div id="price"></div>
search.addWidget(
instantsearch.widgets.rangeSlider({
container: '#price',
facetName: 'price',
tooltips: {
format: function(formattedValue) {
return '$' + formattedValue;
}
}
})
);
We support IE9+ and all other modern browsers.
To get IE8 support, please insert this in the <head>
:
<meta http-equiv="X-UA-Compatible" content="IE=Edge">
<!--[if lte IE 8]>
<script src="https://cdnjs.cloudflare.com/ajax/libs/aight/1.2.2/aight.min.js"></script>
<![endif]-->
We use the shawnbot/aight polyfill.
Always put the <script>
after any jQuery
library, see https://github.com/shawnbot/aight/issues/42.
FAQs
InstantSearch.js is a JavaScript library for building performant and instant search experiences with Algolia.
The npm package instantsearch.js receives a total of 200,892 weekly downloads. As such, instantsearch.js popularity was classified as popular.
We found that instantsearch.js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 13 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.