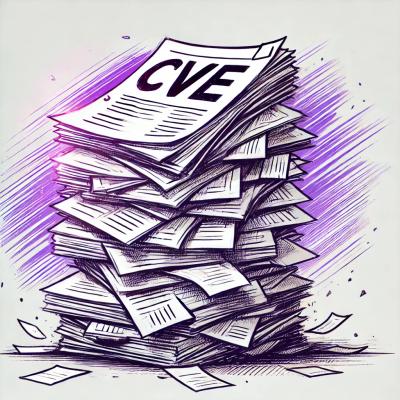
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
integer-prebuild
Advanced tools
Native 64-bit integers with overflow protection.
Native 64-bit signed integers in Node.js.
npm install --save integer
You must be using Node.js v10 or above. Prebuilt binaries are available for LTS versions + Linux/OSX.
var Integer = require('integer');
var a = Integer('7129837312139827189');
var b = a.subtract(1).shiftRight(3);
assert(b.equals('891229664017478398'));
We will not let you perform operations that would result in overflow. If you try to create an Integer
that cannot be represented in 64-bits (signed), we will throw a RangeError
.
// These will each throw a RangeError
var tooBig = Integer(13897283129).multiply(13897283129);
var tooSmall = Integer.MIN_VALUE.subtract(1);
var divideByZero = Integer(123).divide(0);
var alsoTooBig = Integer('4029384203948203948923');
// You are also protected against two's complement overflow (this will throw a RangeError)
var twosComplement = Integer.MIN_VALUE.divide(-1);
It's easy to convert between me and regular JavaScript numbers.
var int = Integer(12345);
assert(int instanceof Integer);
var num = Number(int); // same as int.toNumber()
assert(typeof num === 'number');
However, we will prevent you from converting an Integer
to an unsafe number, and vice-versa. To learn more about unsafe numbers, click here.
// This will throw a RangeError
var unsafe = Integer(Number.MAX_SAFE_INTEGER + 1);
// This is okay
var int = Integer(Number.MAX_SAFE_INTEGER).plus(1);
// But this will throw a RangeError
var unsafe = int.toNumber();
Casts a value to an Integer
. If the value cannot be converted safely and losslessly, a RangeError
is thrown.
var a = Integer();
var b = Integer(12345);
var c = Integer('12345');
assert(a.equals(0));
assert(b.equals(c));
Casts a regular number to an Integer
.
If the number is unsafe the defaultValue
is used instead (or a RangeError
is thrown if no defaultValue
was provided).
Integer.fromNumber(12345, 0); // results in Integer(12345)
Integer.fromNumber(Number.MAX_SAFE_INTEGER + 1, 0); // results in Integer(0)
Casts a string to an Integer
. The string is assumed to be base-10 unless a different radix
is specified.
If conversions fails the defaultValue
is used instead (or a RangeError
is thrown if no defaultValue
was provided).
var hexColor = 'ff55dd';
var int = Integer.fromString(hexColor, 16, 'ffffff');
Creates an Integer
by concatenating two regular 32-bit signed integers. The highBits
are optional and default to 0
.
var int = Integer.fromBits(0x40, 0x20);
int.toString(16); // => '2000000040'
Performs the arithmetic operation and returns a new Integer
. The argument must either be a number, a base-10 string, or an Integer
. If the operation results in overflow, a RangeError
is thrown.
Returns the unary negation (-value
) of the Integer
.
Returns the absolute value of the Integer
.
Performs the bitwise operation and returns a new Integer
. The argument must either be a number, a base-10 string, or an Integer
.
Shifts the Integer
by specified number of bits and returns the result.
Performs the logical operation and returns true
or false
. The argument must either be a number, a base-10 string, or an Integer
.
Compares the value of the Integer
and other
, resulting in:
-1
if this
is less than other
1
if this
is greater than other
0
if this
is equal to other
Converts the Integer
to a string. A base-10 string is returned unless a different radix
is specified.
Converts the Integer
to a regular number. If the Integer
is not within the safe range, a RangeError
is thrown.
Converts the Integer
to a regular number, even if the conversion would result in a loss of precision. This method will never throw an error.
Returns the number of bits necessary to hold the absolute value of the Integer
.
Integer(0).bitSizeAbs(); // => 1
Integer(128).bitSizeAbs(); // => 8
Integer(-255).bitSizeAbs(); // => 8
Integer.fromString('4fffffffffff', 16).bitSizeAbs(); // => 47
These methods are self-explanatory.
Returns whether or not the Integer
is within the safe range. If it's not within the safe range, trying to convert it to a regular number would result in a RangeError
being thrown.
The safe range is defined as n >= Number.MIN_SAFE_INTEGER && n <= Number.MAX_SAFE_INTEGER
.
Determines if the given value is an Integer
object.
Integer
Integer
Integer
Integer
Integer
with a value of 0
Integer
with a value of 1
Integer
with a value of -1
FAQs
Native 64-bit integers with overflow protection.
The npm package integer-prebuild receives a total of 0 weekly downloads. As such, integer-prebuild popularity was classified as not popular.
We found that integer-prebuild demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.