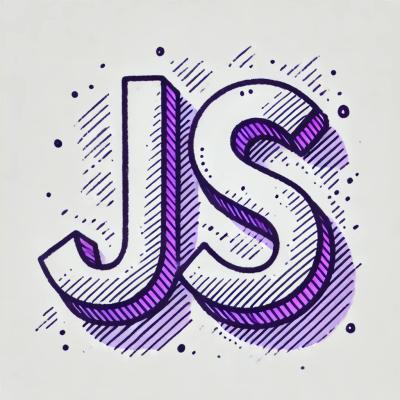
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
A powerful and lightweight inversion of control container for JavaScript and Node.js apps powered by TypeScript.
Inversify is a powerful and flexible inversion of control (IoC) container for JavaScript and TypeScript applications. It helps in managing dependencies and promoting decoupling in your codebase by using dependency injection (DI).
Dependency Injection
This code demonstrates how to use Inversify for dependency injection. It defines a `Katana` class and a `Ninja` class, where `Ninja` depends on `Katana`. The `Container` is used to bind these classes to their respective types, and the `Ninja` instance is resolved from the container.
const { Container, injectable, inject } = require('inversify');
const TYPES = { Warrior: Symbol.for('Warrior'), Weapon: Symbol.for('Weapon') };
@injectable()
class Katana {
hit() {
return 'cut!';
}
}
@injectable()
class Ninja {
constructor(@inject(TYPES.Weapon) weapon) {
this._weapon = weapon;
}
fight() {
return this._weapon.hit();
}
}
const container = new Container();
container.bind(TYPES.Weapon).to(Katana);
container.bind(TYPES.Warrior).to(Ninja);
const ninja = container.get(TYPES.Warrior);
console.log(ninja.fight()); // Output: cut!
Auto-Wiring
This code demonstrates the auto-wiring feature of Inversify. By setting `autoBindInjectable` to true, you can automatically bind classes marked with `@injectable()` without explicitly binding them in the container.
const { Container, injectable, inject } = require('inversify');
const TYPES = { Warrior: Symbol.for('Warrior'), Weapon: Symbol.for('Weapon') };
@injectable()
class Shuriken {
throw() {
return 'thrown!';
}
}
@injectable()
class Samurai {
constructor(@inject(TYPES.Weapon) weapon) {
this._weapon = weapon;
}
attack() {
return this._weapon.throw();
}
}
const container = new Container({ autoBindInjectable: true });
container.bind(TYPES.Weapon).to(Shuriken);
const samurai = container.get(Samurai);
console.log(samurai.attack()); // Output: thrown!
Middleware
This code demonstrates how to use middleware in Inversify. Middleware can be applied to the container to intercept and log actions during the resolution of dependencies.
const { Container, injectable, inject } = require('inversify');
const TYPES = { Warrior: Symbol.for('Warrior'), Weapon: Symbol.for('Weapon') };
@injectable()
class Bow {
shoot() {
return 'arrow shot!';
}
}
@injectable()
class Archer {
constructor(@inject(TYPES.Weapon) weapon) {
this._weapon = weapon;
}
attack() {
return this._weapon.shoot();
}
}
const container = new Container();
container.applyMiddleware((planAndResolve) => {
return (args) => {
console.log('Middleware: Resolving dependencies...');
return planAndResolve(args);
};
});
container.bind(TYPES.Weapon).to(Bow);
container.bind(TYPES.Warrior).to(Archer);
const archer = container.get(TYPES.Warrior);
console.log(archer.attack()); // Output: Middleware: Resolving dependencies... arrow shot!
Awilix is a lightweight dependency injection container for JavaScript and TypeScript. It offers a similar feature set to Inversify, including support for class-based and function-based dependency injection. Awilix is known for its simplicity and ease of use, making it a good alternative for smaller projects or those who prefer a less verbose API.
TypeDI is a dependency injection tool for TypeScript and JavaScript. It provides decorators and a service container to manage dependencies. TypeDI is similar to Inversify in its use of decorators and TypeScript support, but it is generally considered to be more lightweight and easier to set up.
TSyringe is a lightweight dependency injection container for TypeScript. It uses decorators and reflection to manage dependencies, similar to Inversify. TSyringe is known for its minimalistic design and ease of integration with existing TypeScript projects.
A powerful and lightweight inversion of control container for JavaScript & Node.js apps powered by TypeScript.
InversifyJS is a lightweight (4KB) inversion of control (IoC) container for TypeScript and JavaScript apps. An IoC container uses a class constructor to identify and inject its dependencies. InversifyJS has a friendly API and encourages the usage of the best OOP and IoC practices.
JavaScript now supports object oriented (OO) programming with class based inheritance. These features are great but the truth is that they are also dangerous.
We need a good OO design (SOLID, Composite Reuse, etc.) to protect ourselves from these threats. The problem is that OO design is difficult and that is exactly why we created InversifyJS.
InversifyJS is a tool that helps JavaScript developers write code with good OO design.
InversifyJS has been developed with 4 main goals:
Allow JavaScript developers to write code that adheres to the SOLID principles.
Facilitate and encourage the adherence to the best OOP and IoC practices.
Add as little runtime overhead as possible.
Provide a state of the art development experience.
Nate Kohari - Author of Ninject
"Nice work! I've taken a couple shots at creating DI frameworks for JavaScript and TypeScript, but the lack of RTTI really hinders things. The ES7 metadata gets us part of the way there (as you've discovered). Keep up the great work!"
Michel Weststrate - Author of MobX
Dependency injection like InversifyJS works nicely
You can get the latest release and the type definitions using npm:
$ npm install inversify reflect-metadata --save
The InversifyJS type definitions are included in the inversify npm package.
:warning: Important! InversifyJS requires TypeScript >= 2.0 and the
experimentalDecorators
,emitDecoratorMetadata
,types
andlib
compilation options in yourtsconfig.json
file.
{
"compilerOptions": {
"target": "es5",
"lib": ["es6"],
"types": ["reflect-metadata"],
"module": "commonjs",
"moduleResolution": "node",
"experimentalDecorators": true,
"emitDecoratorMetadata": true
}
}
InversifyJS requires a modern JavaScript engine with support for:
If your environment doesn't support one of these you will need to import a shim or polyfill.
:warning: The
reflect-metadata
polyfill should be imported only once in your entire application because the Reflect object is mean to be a global singleton. More details about this can be found here.
Check out the Environment support and polyfills page in the wiki and the Basic example to learn more.
Let’s take a look at the basic usage and APIs of InversifyJS with TypeScript:
Our goal is to write code that adheres to the dependency inversion principle. This means that we should "depend upon Abstractions and do not depend upon concretions". Let's start by declaring some interfaces (abstractions).
// file interfaces.ts
interface Warrior {
fight(): string;
sneak(): string;
}
interface Weapon {
hit(): string;
}
interface ThrowableWeapon {
throw(): string;
}
InversifyJS need to use the type as identifiers at runtime. We use symbols as identifiers but you can also use classes and or string literals.
// file types.ts
const TYPES = {
Warrior: Symbol.for("Warrior"),
Weapon: Symbol.for("Weapon"),
ThrowableWeapon: Symbol.for("ThrowableWeapon")
};
export { TYPES };
Note: It is recommended to use Symbols but InversifyJS also support the usage of Classes and string literals (please refer to the features section to learn more).
@injectable
& @inject
decoratorsLet's continue by declaring some classes (concretions). The classes are implementations of the interfaces that we just declared. All the classes must be annotated with the @injectable
decorator.
When a class has a dependency on an interface we also need to use the @inject
decorator to define an identifier for the interface that will be available at runtime. In this case we will use the Symbols Symbol.for("Weapon")
and Symbol.for("ThrowableWeapon")
as runtime identifiers.
// file entities.ts
import { injectable, inject } from "inversify";
import "reflect-metadata";
import { Weapon, ThrowableWeapon, Warrior } from "./interfaces"
import { TYPES } from "./types";
@injectable()
class Katana implements Weapon {
public hit() {
return "cut!";
}
}
@injectable()
class Shuriken implements ThrowableWeapon {
public throw() {
return "hit!";
}
}
@injectable()
class Ninja implements Warrior {
private _katana: Weapon;
private _shuriken: ThrowableWeapon;
public constructor(
@inject(TYPES.Weapon) katana: Weapon,
@inject(TYPES.ThrowableWeapon) shuriken: ThrowableWeapon
) {
this._katana = katana;
this._shuriken = shuriken;
}
public fight() { return this._katana.hit(); }
public sneak() { return this._shuriken.throw(); }
}
export { Ninja, Katana, Shuriken };
If you prefer it you can use property injection instead of constructor injection so you don't have to declare the class constructor:
@injectable()
class Ninja implements Warrior {
@inject(TYPES.Weapon) private _katana: Weapon;
@inject(TYPES.ThrowableWeapon) private _shuriken: ThrowableWeapon;
public fight() { return this._katana.hit(); }
public sneak() { return this._shuriken.throw(); }
}
We recommend to do this in a file named inversify.config.ts
. This is the only place in which there is some coupling.
In the rest of your application your classes should be free of references to other classes.
// file inversify.config.ts
import { Container } from "inversify";
import { TYPES } from "./types";
import { Warrior, Weapon, ThrowableWeapon } from "./interfaces";
import { Ninja, Katana, Shuriken } from "./entities";
const myContainer = new Container();
myContainer.bind<Warrior>(TYPES.Warrior).to(Ninja);
myContainer.bind<Weapon>(TYPES.Weapon).to(Katana);
myContainer.bind<ThrowableWeapon>(TYPES.ThrowableWeapon).to(Shuriken);
export { myContainer };
You can use the method get<T>
from the Container
class to resolve a dependency.
Remember that you should do this only in your composition root
to avoid the service locator anti-pattern.
import { myContainer } from "./inversify.config";
import { TYPES } from "./types";
import { Warrior } from "./interfaces";
const ninja = myContainer.get<Warrior>(TYPES.Warrior);
expect(ninja.fight()).eql("cut!"); // true
expect(ninja.sneak()).eql("hit!"); // true
As we can see the Katana
and Shuriken
were successfully resolved and injected into Ninja
.
InversifyJS supports ES5 and ES6 and can work without TypeScript. Head to the JavaScript example to learn more!
Let's take a look to the InversifyJS features!
Please refer to the wiki for additional details.
In order to provide a state of the art development experience we are also working on:
Please refer to the ecosystem wiki page to learn more.
If you are experience any kind of issues we will be happy to help. You can report an issue using the issues page or the chat. You can also ask questions at Stack overflow using the inversifyjs
tag.
If you want to share your thoughts with the development team or join us you will be able to do so using the official the mailing list. You can check out the wiki to learn more about InversifyJS internals.
Thanks a lot to all the contributors, all the developers out there using InversifyJS and all those that help us to spread the word by sharing content about InversifyJS online. Without your feedback and support this project would not be possible.
License under the MIT License (MIT)
Copyright © 2015-2017 Remo H. Jansen
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
A powerful and lightweight inversion of control container for JavaScript and Node.js apps powered by TypeScript.
The npm package inversify receives a total of 630,969 weekly downloads. As such, inversify popularity was classified as popular.
We found that inversify demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.