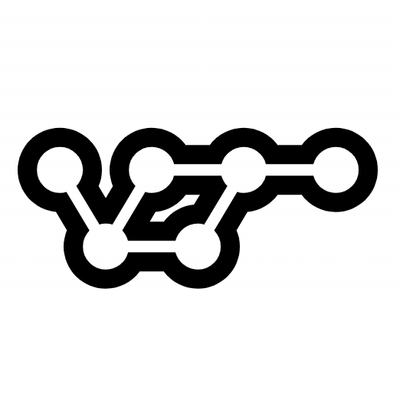
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
ionic4-auto-complete
Advanced tools
[](https://www.npmjs.com/package/ionic4-auto-complete/) [](https://www.npmjs.com/package/ionic4-auto-complete) [:
import { AutoCompleteModule } from 'ionic4-auto-complete';
...
@NgModule({
...
imports: [
AutoCompleteModule,
...
],
...
})
export class AppModule {}
Import scss stylesheet from node_modules
(i.e. app.scss
, global.scss
):
@import "../../node_modules/ionic4-auto-complete/auto-complete";
The component is not responsible for getting the data from the server. There are two options for providing data to the component.
import {Component} from '@angular/core';
@Component({
selector: 'auto-complete-component',
templateUrl: 'auto-complete-component.component.html',
styleUrls: [
'auto-complete-component.component.scss'
],
})
export class AutoCompleteComponent {
public labelAttribute:string;
public objects:any[];
constructor() {
const objects = [
...
]
}
protected filter(keyword) {
keyword = keyword.toLowerCase();
return this.objects.filter(
(object) => {
const value = object[this.labelAttribute].toLowerCase();
return value.includes(keyword);
}
);
}
}
When implementing an AutoCompleteService interface, you must implement two properties:
import {Injectable} from '@angular/core';
import {HttpClient} from '@angular/common/http';
import {map} from 'rxjs/operators';
import {Observable, of} from 'rxjs';
import {AutoCompleteService} from 'ionic4-auto-complete';
@Injectable()
export class SimpleService implements AutoCompleteService {
labelAttribute = 'name';
private countries:any[] = [];
constructor(private http:HttpClient) {
}
getResults(keyword:string):Observable<any[]> {
let observable:Observable<any>;
if (this.countries.length === 0) {
observable = this.http.get('https://restcountries.eu/rest/v2/all');
} else {
observable = of(this.countries);
}
return observable.pipe(
map(
(result) => {
return result.filter(
(item) => {
return item.name.toLowerCase().startsWith(
keyword.toLowerCase()
);
}
);
}
)
);
}
}
To indicate that you don't want the label as value but another field of the country object returned by the REST service, you can specify the attribute formValueAttribute on your dataProvider. For example, we want to use the country numeric code as value and still use the country name as label.
Create a service which includes the formValueAttribute
property.
import {Injectable} from '@angular/core';
import {map} from 'rxjs/operators';
import {HttpClient} from '@angular/common/http';
import {AutoCompleteService} from 'ionic4-auto-complete';
@Injectable()
export class CompleteTestService implements AutoCompleteService {
labelAttribute = 'name';
formValueAttribute = 'numericCode';
constructor(private http:HttpClient) {
}
getResults(keyword:string) {
if (!keyword) { return false; }
return this.http.get('https://restcountries.eu/rest/v2/name/' + keyword).pipe(map(
(result: any[]) => {
return result.filter(
(item) => {
return item.name.toLowerCase().startsWith(
keyword.toLowerCase()
);
}
);
}
));
}
}
country
is the selected country's numericCode while the displayed name is the labelAttribute
.Create a component:
import {Component} from '@angular/core';
import {NavController} from 'ionic-angular';
import {CompleteTestService} from '../../providers/CompleteTestService';
import {FormGroup, Validators, FormControl } from '@angular/forms'
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
myForm: FormGroup
constructor(public navCtrl: NavController, public completeTestService: CompleteTestService) {
}
ngOnInit(): void {
this.myForm = new FormGroup({
country: new FormControl('', [
Validators.required
])
})
}
submit(): void {
let country = this.myForm.value.country
}
}
Add ion-auto-complete
within the HTML of your parent module.
Pass the data:
<ion-auto-complete [dataProvider]="completeTestService"></ion-auto-complete>`
Requires labelAttribute
as both label and form value (default behavior).
labelAttribute
property is used to take the good field of each object to display in the suggestion list. For backward compatibility, if nothing is specified, this attribute is also used to grab the value used in the form.Add form to the component's HTML and add the formControlName
attribute:
<form [formGroup]="myForm"
(ngSubmit)="submit()"
novalidate>
<div class="ion-form-group">
<ion-auto-complete [dataProvider]="completeTestService"
formControlName="country"></ion-auto-complete>
</div>
<button ion-button
type="submit"
block>
Add Country
</button>
</form>
Simply set formValueAttribute
to empty string:
import {AutoCompleteService} from 'ionic4-auto-complete';
import {HttpClient} from '@angular/common/http';
import {Injectable} from "@angular/core";
import 'rxjs/add/operator/map'
@Injectable()
export class CompleteTestService implements AutoCompleteService {
...
formValueAttribute = ''
constructor(private http:HttpClient) {
...
}
getResults(keyword:string) {
...
}
}
autoFocus($event)
is fired when the input is focused.autoBlur($event)
is fired when the input is blured.ionAutoInput($event)
is fired when user inputs.itemChanged($event)
is fired when the selection changes (clicked).itemsHidden($event)
is fired when items are hidden.itemRemoved($event)
is fired when item is removed (clicked).itemSelected($event)
is fired when item is selected from suggestions (clicked).itemsShown($event)
is fired when items are shown.Ionic4-auto-complete supports the regular Ionic's Searchbar properties, which are set to their default values as specified in the docs.
You can override these default values by adding the [options]
attribute to the <ion-auto-complete>
tag, for instance:
<ion-auto-complete [dataProvider]="someProvider" [options]="{ placeholder : 'Lorem Ipsum' }"></ion-auto-complete>
Options include, but not limited to:
color
- (default is null
)debounce
- (default is 250
)type
- ("text", "password", "email", "number", "search", "tel", "url". Default "search".)placeholder
- (default "Search")For best visual results use viewport size / fixed size
( in pixels).
ion-auto-complete {
width: 50vw;
}
You can display any attribute associated with your data items by accessing it from the data
input class member in the template.
For example:
Let's assume that in addition to the country name, we also wish to display the country flag.
For that, we use the ng-template
directive, which let's us pass the template as an input to the component.
Within your component's HTML add the a template:
<ng-template #withFlags let-attrs="attrs">
<img src="assets/image/flags/{{attrs.data.name}}.png"
class="flag"/>
<span [innerHTML]="attrs.data.name | boldprefix:attrs.keyword"></span>
</ng-template>
<ion-auto-complete [dataProvider]="service"
[template]="withFlags"></ion-auto-complete>
IMPORTANT: The attribute let-attrs
is required.
In addition to the searchbar options, ion-auto-complete
also supports the following option attributes:
[template]
(TemplateRef) - custom template reference for your auto complete items (see below).[emptytemplate]
(TemplateRef) - custom template reference for your auto complete no items display.[selectionTemplate]
(TemplateRef) - custom template reference for your own selection display when using multi.[clearInvalidInput]
(boolean) - automatically clear the input field if a valid option is not selected from suggestions (Default true
).[showResultsFirst]
(boolean) - for small lists it might be nicer to show all options on first tap (you might need to modify your service to handle an empty keyword
).[maxResults]
(number) - limits the max number of suggestions shown (Default 8
).[alwaysShowList]
(boolean) - always show the list - defaults to false).[hideListOnSelection]
(boolean) - if allowing multiple selections, it might be nice not to dismiss the list after each selection - defaults to true).Within your component:
@ViewChild('searchbar')
searchbar: AutoCompleteComponent;
Add #searchbar
within your component's HTML:
<ion-auto-complete [dataProvider]="provider" #searchbar></ion-auto-complete>
getValue()
returns the string value of the selected item.
this.searchbar.getValue()
getSelection()
returns the selected object.
this.searchbar.getSelection()
setFocus()
sets focus on the searchbar.
this.searchbar.setFocus()
To contribute, clone the repo. Then, run npm install
to get the packages needed for the library to work. Running gulp
will run a series of tasks that builds the files in /src
into /dist
. Replace the /dist
into whatever Ionic application's node_modules
where you're testing your changes to continuously improve the library.
If you find any issues feel free to open a request in the Issues tab. If I have the time I will try to solve any issues but cannot make any guarantees. Feel free to contribute yourself.
Run npm install
to get packages required for the demo and then run ionic serve
to run locally.
npm run docs:build
package.json
files in both the root and dist/
directory following Semantic Versioning (2.0.0).npm run build
from root.dist/
contents into demo/node_modules/ionic4-auto-complete/
cp -fr dist/* demo/node_modules/ionic4-auto-complete/
ionic serve
from demo/
ionic build --prod
from demo/
npm publish
from dist/
directory.CHANGELOG.md
in root.The auto-complete component allows you to use templates for customize the display of each suggestion. But in many cases, the default template is good. However, you need to concatenate several fields (like firstname and lastname) to produce a full label. In that case, you can declare a method named getItemLabel
instead of using labelAttribute
.
For example, we want to display the country name and the population:
import {AutoCompleteService} from 'ionic4-auto-complete';
import {HttpClient} from '@angular/common/http';
import {Injectable} from "@angular/core";
import 'rxjs/add/operator/map'
@Injectable()
export class CompleteTestService implements AutoCompleteService {
formValueAttribute = ""
constructor(private http:HttpClient) {
}
getResults(keyword:string) {
return this.http.get("https://restcountries.eu/rest/v1/name/"+keyword)
.map(
result =>
{
return result.json()
.filter(item => item.name.toLowerCase().startsWith(keyword.toLowerCase()) )
});
}
getItemLabel(country: any) {
return country.name + ' (' + country.population + ')'
}
}
FAQs
README.md
The npm package ionic4-auto-complete receives a total of 207 weekly downloads. As such, ionic4-auto-complete popularity was classified as not popular.
We found that ionic4-auto-complete demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.