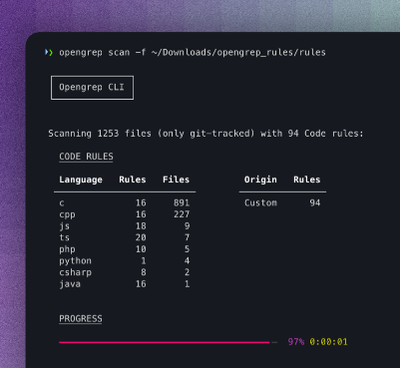
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
This module is designed to provide simple event driven ipc system over native nodejs socket module.
Run the following command to install the module.
npm install ipcevt
Note that this library is written using es6 modules. Please remember to use following nodejs option to use the module
node --experimental-modules [your boot script path]
The ipcevt server is designed as a simple broadcasting server that only receive connection and broadcast events among the clients.
import crypto from "crypto";
import {IPCEvtServer} from "ipcevt";
(new IPCEvtServer())
.on( 'connected', (socket)=>{
// emit when socket is connected to the server!
// Note that the socket object will be persistent in other events too!
socket.id = crypto.randomBytes(20).toString('hex');
console.log( `${socket.id} is connected!` );
})
.on( 'disconnected', (socket)=>{
// emit when socket is disconnected from the server!
console.log( `${socket.id} is disconnected!` );
})
.on( 'netevt', (socket, event, evt_args)=>{
// evt_args are raw buffers, you can manipulate if you want!
console.log( `Receiving ${event} from ${socket.id} (contains ${evt_args.length} arguments)` );
})
.listen( 12334, 'localhost' );
The ipcevt client is designed to behave like normal event emitters.
import {IPCEvtClient} from "ipcevt";
const Client = new IPCEvtClient();
// Register the central serializer to serialize each event argument passed into emit function
Client._serializer = (input)=>{
return Buffer.from(JSON.stringify(input), 'utf8');
};
// Register the central deserializer to automatically deserialize evey event arguments
Client._deserializer = (input)=>{
return JSON.parse(input.toString('utf8'));
};
Client
.on( 'connected', function() {
console.log( "Client has connected to the server!" );
// Note that the input must be a Buffer or an error will be thrown
// You can register the _serializer is to preprocess each argument!
Client.emit( 'greeting', id, 'Hi! There!!!', {a:1, b:2, c:"123", d:Date.now()} );
})
.on( 'disconnected', function() {
console.log( "Client has disconnected from the server!" );
})
.on( 'greeting', function(...args){
// The arguments will be Buffer if the _deserializer is not registered!
console.log(`Receiving [test-event]: `, ...args);
})
.connect( 12334, 'localhost' );
FAQs
A tiny socket based event system
We found that ipcevt demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.