ipfs-unixfs JavaScript Implementation

JavaScript implementation of IPFS' UnixFS (a Unix FileSystem files representation on top of a MerkleDAG)
The UnixFS spec can be found inside the ipfs/specs repository
Lead Maintainer
Alex Potsides
Table of Contents
Install
npm
> npm i ipfs-unixfs
Use in Node.js
var { UnixFS } = require('ipfs-unixfs')
Use in a browser with browserify, webpack or any other bundler
The code published to npm that gets loaded on require is in fact a ES5 transpiled version with the right shims added. This means that you can require it and use with your favourite bundler without having to adjust asset management process.
var { UnixFS } = require('ipfs-unixfs')
Use in a browser Using a script tag
Loading this module through a script tag will make the UnixFS
obj available in the global namespace.
<script src="https://npmcdn.com/ipfs-unixfs/dist/index.min.js"></script>
<script src="https://npmcdn.com/ipfs-unixfs/dist/index.js"></script>
Usage
Examples
Create a file composed by several blocks
const data = new UnixFS({ type: 'file' })
data.addBlockSize(256)
data.addBlockSize(256)
Create a directory that contains several files
Creating a directory that contains several files is achieve by creating a unixfs element that identifies a MerkleDAG node as a directory. The links of that MerkleDAG node are the files that are contained in this directory.
const data = new UnixFS({ type: 'directory' })
API
UnixFS Data Structure
syntax = "proto2";
message Data {
enum DataType {
Raw = 0;
Directory = 1;
File = 2;
Metadata = 3;
Symlink = 4;
HAMTShard = 5;
}
required DataType Type = 1;
optional bytes Data = 2;
optional uint64 filesize = 3;
repeated uint64 blocksizes = 4;
optional uint64 hashType = 5;
optional uint64 fanout = 6;
optional uint32 mode = 7;
optional UnixTime mtime = 8;
}
message UnixTime {
required int64 Seconds = 1;
optional fixed32 FractionalNanoseconds = 2;
}
message Metadata {
optional string MimeType = 1;
}
create an unixfs Data element
const data = new UnixFS([options])
options
is an optional object argument that might include the following keys:
- type (string, default
file
): The type of UnixFS entry. Can be:
raw
directory
file
metadata
symlink
hamt-sharded-directory
- data (Uint8Array): The optional data field for this node
- blockSizes (Array, default:
[]
): If this is a file
node that is made up of multiple blocks, blockSizes
is a list numbers that represent the size of the file chunks stored in each child node. It is used to calculate the total file size. - mode (Number, default
0644
for files, 0755
for directories/hamt-sharded-directories) file mode - mtime (
Date
, { secs, nsecs }
, { Seconds, FractionalNanoseconds }
, [ secs, nsecs ]
): The modification time of this node
add and remove a block size to the block size list
data.addBlockSize(<size in bytes>)
data.removeBlockSize(<index>)
get total fileSize
data.fileSize()
marshal and unmarshal
const marshaled = data.marshal()
const unmarshaled = Unixfs.unmarshal(marshaled)
is this UnixFS entry a directory?
const dir = new Data({ type: 'directory' })
dir.isDirectory()
const file = new Data({ type: 'file' })
file.isDirectory()
has an mtime been set?
If no modification time has been set, no mtime
property will be present on the Data
instance:
const file = new Data({ type: 'file' })
file.mtime
Object.prototype.hasOwnProperty.call(file, 'mtime')
const dir = new Data({ type: 'dir', mtime: new Date() })
dir.mtime
Contribute
Feel free to join in. All welcome. Open an issue!
This repository falls under the IPFS Code of Conduct.
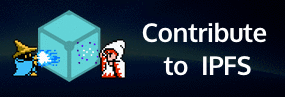
License
MIT