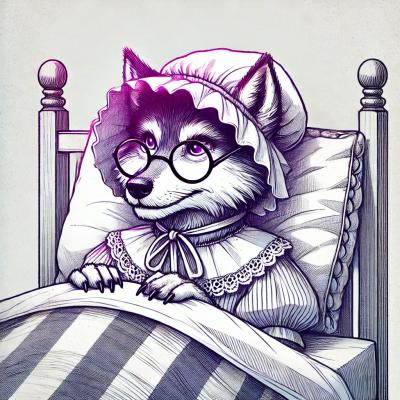
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
JS type check (TypeScript supported) functions like `isPlainObject() isArray()` etc. A simple & small integration.
The is-what npm package provides utility functions to check the type of a given value. It helps in determining whether a value is an object, array, function, string, number, etc. This can be particularly useful in type-checking scenarios and ensuring that values conform to expected types.
isObject
The isObject function checks if a given value is a plain object. It returns true for plain objects and false for arrays and other types.
const { isObject } = require('is-what');
console.log(isObject({})); // true
console.log(isObject([])); // false
isArray
The isArray function checks if a given value is an array. It returns true for arrays and false for other types.
const { isArray } = require('is-what');
console.log(isArray([])); // true
console.log(isArray({})); // false
isFunction
The isFunction function checks if a given value is a function. It returns true for functions and false for other types.
const { isFunction } = require('is-what');
console.log(isFunction(function() {})); // true
console.log(isFunction({})); // false
isString
The isString function checks if a given value is a string. It returns true for strings and false for other types.
const { isString } = require('is-what');
console.log(isString('hello')); // true
console.log(isString(123)); // false
isNumber
The isNumber function checks if a given value is a number. It returns true for numbers and false for other types.
const { isNumber } = require('is-what');
console.log(isNumber(123)); // true
console.log(isNumber('123')); // false
Lodash is a popular utility library that provides a wide range of functions for manipulating arrays, objects, and other types. It includes type-checking functions similar to is-what, such as _.isObject, _.isArray, _.isFunction, _.isString, and _.isNumber. Lodash offers more comprehensive functionality beyond type-checking.
Underscore is another utility library that provides functional programming helpers for working with arrays, objects, and other types. It includes type-checking functions like _.isObject, _.isArray, _.isFunction, _.isString, and _.isNumber. Underscore is similar to Lodash but with a smaller footprint and fewer features.
Type-detect is a simple library for detecting the type of a given value. It provides functions like typeDetect(value) which returns a string representing the type of the value. While it offers similar functionality to is-what, it focuses on returning type names rather than boolean checks.
Very simple & small JS type check functions. It's fully TypeScript supported!
npm i is-what
Or for deno available at: "deno.land/x/is_what"
Also check out is-where 🙈
I built is-what because the existing solutions were all too complex or too poorly built.
I was looking for:
{}
or a special object (like a class instance) ‼️And that's exactly what is-what
is! (what a great wordplay 😃)
is-what is really easy to use, and most functions work just like you'd expect.
// import functions you want to use like so:
import { isString, isDate, isPlainObject } from 'is-what'
isNumber
and isDate
have special treatment.// basics
isBoolean(true) // true
isBoolean(false) // true
isUndefined(undefined) // true
isNull(null) // true
// strings
isString('') // true
isEmptyString('') // true
isFullString('') // false
// numbers
isNumber(0) // true
isNumber('0') // false
isNumber(NaN) // false *
isPositiveNumber(1) // true
isNegativeNumber(-1) // true
// * see below for special NaN use cases!
// arrays
isArray([]) // true
isEmptyArray([]) // true
isFullArray([1]) // true
// objects
isPlainObject({}) // true *
isEmptyObject({}) // true
isFullObject({ a: 1 }) // true
// * see below for special object (& class instance) use cases!
// functions
isFunction(function () {}) // true
isFunction(() => {}) // true
// dates
isDate(new Date()) // true
isDate(new Date('invalid date')) // false
// maps & sets
isMap(new Map()) // true
isSet(new Set()) // true
isWeakMap(new WeakMap()) // true
isWeakSet(new WeakSet()) // true
// others
isRegExp(/\s/gi) // true
isSymbol(Symbol()) // true
isBlob(new Blob()) // true
isFile(new File([''], '', { type: 'text/html' })) // true
isError(new Error('')) // true
isPromise(new Promise((resolve) => {})) // true
// primitives
isPrimitive('') // true
// true for any of: boolean, null, undefined, number, string, symbol
isNaN
is a built-in JS Function but it really makes no sense:
// 1)
typeof NaN === 'number' // true
// 🤔 ("not a number" is a "number"...)
// 2)
isNaN('1') // false
// 🤔 the string '1' is not-"not a number"... so it's a number??
// 3)
isNaN('one') // true
// 🤔 'one' is NaN but `NaN === 'one'` is false...
With is-what the way we treat NaN makes a little bit more sense:
import { isNumber, isNaNValue } from 'is-what'
// 1)
isNumber(NaN) // false!
// let's not treat NaN as a number
// 2)
isNaNValue('1') // false
// if it's not NaN, it's not NaN!!
// 3)
isNaNValue('one') // false
// if it's not NaN, it's not NaN!!
isNaNValue(NaN) // true
Checking for a JavaScript object can be really difficult. In JavaScript you can create classes that will behave just like JavaScript objects but might have completely different prototypes. With is-what I went for this classification:
isPlainObject
will only return true
on plain JavaScript objects and not on classes or othersisAnyObject
will be more loose and return true
on regular objects, classes, etc.// define a plain object
const plainObject = { hello: 'I am a good old object.' }
// define a special object
class SpecialObject {
constructor(somethingSpecial) {
this.speciality = somethingSpecial
}
}
const specialObject = new SpecialObject('I am a special object! I am a class instance!!!')
// check the plain object
isPlainObject(plainObject) // returns true
isAnyObject(plainObject) // returns true
getType(plainObject) // returns 'Object'
// check the special object
isPlainObject(specialObject) // returns false !!!!!!!!!
isAnyObject(specialObject) // returns true
getType(specialObject) // returns 'Object'
Please note that
isPlainObject
will only returntrue
for normal plain JavaScript objects.
You can check for specific types with getType
and isType
:
import { getType, isType } from 'is-what'
getType('') // returns 'String'
// pass a Type as second param:
isType('', String) // returns true
If you just want to make sure your object inherits from a particular class or
toStringTag
value, you can use isInstanceOf()
like this:
import { isInstanceOf } from 'is-what'
isInstanceOf(new XMLHttpRequest(), 'EventTarget')
// returns true
isInstanceOf(globalThis, ReadableStream)
// returns false
is-what makes TypeScript know the type during if statements. This means that a check returns the type of the payload for TypeScript users.
function isNumber(payload: any): payload is number {
// return boolean
}
// As you can see above, all functions return a boolean for JavaScript, but pass the payload type to TypeScript.
// usage example:
function fn(payload: string | number): number {
if (isNumber(payload)) {
// ↑ TypeScript already knows payload is a number here!
return payload
}
return 0
}
isPlainObject
and isAnyObject
with TypeScript will declare the payload to be an object type with any props:
function isPlainObject(payload: any): payload is { [key: string]: any }
function isAnyObject(payload: any): payload is { [key: string]: any }
// The reason to return `{[key: string]: any}` is to be able to do
if (isPlainObject(payload) && payload.id) return payload.id
// if isPlainObject() would return `payload is object` then it would give an error at `payload.id`
If you want more control over what kind of interface/type is casted when checking for objects.
To cast to a specific type while checking for isAnyObject
, can use isObjectLike<T>
:
import { isObjectLike } from 'is-what'
const payload = { name: 'Mesqueeb' } // current type: `{ name: string }`
// Without casting:
if (isAnyObject(payload)) {
// in here `payload` is casted to: `Record<string | number | symbol, any>`
// WE LOOSE THE TYPE!
}
// With casting:
// you can pass a specific type for TS that will be casted when the function returns
if (isObjectLike<{ name: string }>(payload)) {
// in here `payload` is casted to: `{ name: string }`
}
Please note: this library will not actually check the shape of the object, you need to do that yourself.
isObjectLike<T>
works like this under the hood:
function isObjectLike<T extends object>(payload: any): payload is T {
return isAnyObject(payload)
}
It's litterally just these functions:
function getType(payload) {
return Object.prototype.toString.call(payload).slice(8, -1)
}
function isUndefined(payload) {
return getType(payload) === 'Undefined'
}
function isString(payload) {
return getType(payload) === 'String'
}
function isAnyObject(payload) {
return getType(payload) === 'Object'
}
// etc...
See the full source code here.
FAQs
JS type check (TypeScript supported) functions like `isPlainObject() isArray()` etc. A simple & small integration.
The npm package is-what receives a total of 3,118,617 weekly downloads. As such, is-what popularity was classified as popular.
We found that is-what demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.