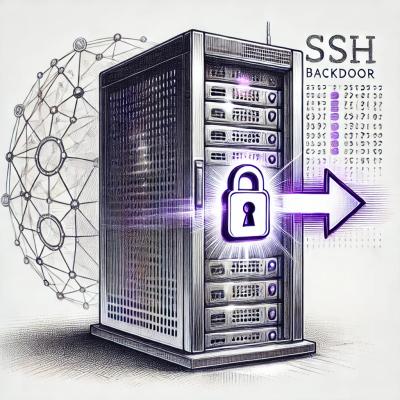
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
A collection of composable JavaScript runtime type predicates with TypeScript type guard declarations
Isntnt is a collection of composable JavaScript runtime type predicates with TypeScript type guard declarations. Supports generics including union and intersection types.
(floor: number) => (value: unknown) => value is number
const isAboveZero = above(0)
<T extends Array<Predicate<any>>>(...predicates: T) => (value: unknown) => value is Predicate<Intersect<Static<T[number]>>>
const isBetween0And21 = and(above(0), below(21))
const isUser = shape({ name: isString })
const hasEmailAddress = at('email', isString)
const isUserWithEmail = and(isUser, hasEmailAddress) // (value: unknown) => { name: string } & { email: string }
<T>(predicate: Predicate<T>) => (value: unknown) => value is Array<T>
const isAnyArray = array(isAny) // (value: unknown) => value is Array<any>
<T extends PropertyKey, U>(key: T, predicate: Predicate<U>) => (value: unknown) => value is { [P in T]: U }
const isAnyAtFoo = at('foo', isAny) // (value: unknown) => value is { foo: any }
(max: number) => (value: unknown) => value is number
const isBelow21 = below(21)
<T extends Array<Primitive>>(...literalValues: T) => (value: unknown) => value is T[number]
const isFooOrBar = either('foo', 'bar') // (value: unknown) => value is 'foo' | 'bar'
<T extends PropertyKey>(key: T) => (value: unknown) => value is { [P in T]: unknown }
const hasFoo = has('foo') // (value: unknown) => value is { 'foo': unknown }
<T extends Constructor<any, any>>(constructor: T) => (value: unknown) => value is InstanceType<T>
const isInstanceofString = instance(String) // (value: unknown) => value is String
<T extends Primitive>(literalValue: T) => (value: unknown) => value is T
const is42 = literal(42) // (value: unknown) => value is 42
<T extends number>(max: number) => (value: unknown) => value is number
const isMax255 = max(255)
<T>(predicate: Predicate<T>) => (value: unknown) => value is T | null | undefined
const isMaybeString = maybe(isString) // (value: unknown) => value is string | null | undefined
(min: number) => (value: unknown) => value is number
const isMin18 = min(18)
Aliases maybe
<T>(predicate: Predicate<T>) => (value: unknown) => value is T | null
const isNullableString = nullable(isString) // (value: unknown) => value is string | null
<T>(predicate: Predicate<T>) => (value: unknown) => value is Record<any, T>
const isEnum = object(isUint) // (value: unknown) => value is Record<any, number>
<T>(predicate: Predicate<T>) => (value: unknown) => value is T | undefined
const isOptionalString = optional(isString) // (value: unknown) => value is string | undefined
<T extends Array<Predicate<any>>>(...predicates: T) => (value: unknown) => value is Static<T[number]>
const isStringOrNumber = or(isString, isNumber) // (value: unknown) => value is string | number
<T extends PropertyKey, U>(keyPredicate: Predicate<T>, valuePredicate: Predicate<U>) => (value: unknown) => value is Record<T, U>
const isDictionary = record(isString, isInt) // (value: unknown) => value is Record<string, number>
<T extends Record<PropertyKey, Predicate<any>>>(predicates: T) => (value: unknown) => value is { [P in keyof T]: Static<T[P]> }
Note: Actual signature also considers optional members (
{ name?: T }
) in itsPredicate
type
const isCoordinate = shape({ x: isNumber, y: isNumber }) // (value: unknown) => value is { x: number, y: number }
(expression: RegExp) => (value: unknown) => value is string
const isSlug = test(/^[\w-]+$/)
<T extends Array<any>>(...predicates: { [K in keyof T]: Predicate<T[K]> }) => (value: unknown) => value is T
const isPoint = tuple(isNumber, isNumber) // (value: unknown) => value is [number, number]
(value: unknown) => value is any
Always returns true
.
isAny(value)
(value: unknown) => value is Array<unknown>
isArray(value)
(value: unknown) => value is Record<number, unknown>
isArrayLike(value)
(value: unknown) => value is bigint
isBigInt(value)
(value: unknown) => value is boolean
isBoolean(value)
(value: unknown) => value is Date
isDate(value)
(value: unknown) => value is Record<any, string>
isDictionary(value)
(value: unknown) => value is false
isFalse(value)
(value: unknown) => value is Function
isFunction(value)
(value: unknown) => value is number
isInt(value)
(value: unknown) => value is number
isInt8(value)
(value: unknown) => value is number
isInt16(value)
(value: unknown) => value is number
isInt32(value)
(value: unknown) => value is number
isLength(value)
(value: unknown) => value is Map<any, unknown>
isMap(value)
(value: unknown) => value is number
isNegative(value)
(value: unknown) => value is never
Always returns false
;
isNever(value)
(value: unknown) => value is null | undefined
isNone(value)
(value: unknown) => value is null
isNull(value)
(value: unknown) => value is number
isNumber(value)
(value: unknown) => value is object
isObject(value)
(value: unknown) => value is ObjectLike
isObjectLike(value)
(value: unknown) => value is {}
isPlainObject(value)
(value: unknown) => value is number
isPositive(value)
(value: unknown) => value is Primitive
isPrimitive(value)
(value: unknown) => value is RegExp
isRegExp(value)
(value: unknown) => value is Serializable
isSerializable(value)
(value: unknown) => value is Array<Serializable>
isSerializableArray(value)
(value: unknown) => value is number
isSerializableNumber(value)
(value: unknown) => value is Record<string, Serializable>
isSerializableObject(value)
(value: unknown) => value is SerializablePrimitive
isSerializablePrimitive(value)
(value: unknown) => value is Set<unknown>
isSet(value)
(value: unknown) => value is Some
isSome(value)
(value: unknown) => value is string
isString(value)
(value: unknown) => value is symbol
isSymbol(value)
(value: unknown) => value is true
isTrue(value)
(value: unknown) => value is number
isUint(value)
(value: unknown) => value is number
isUint8(value)
(value: unknown) => value is number
isUint16(value)
(value: unknown) => value is number
isUint32(value)
(value: unknown) => value is undefined
isUndefined(value)
(value: unknown) => value is WeakMap<any, unknown>
isWeakMap(value)
(value: unknown) => value is { length: number }
isWithLength(value)
Intersect<A | B> // A & B
Maybe<T> // T | null | undefined
type MaybeString = Maybe<string> // string | null | undefined
None // null | undefined
Nullable<T> // T | null
type NullableString = Nullable<string> // string | null
Optional<T> // T | undefined
type OptionalString = Optional<string> // string | undefined
Predicate<T> // (value: unknown, ...rest: Array<unknown>) => value is T
Primitive // null | undefined | boolean | number | string | symbol | bigint
Serializable // SerializableArray | SerializableObject | SerializablePrimitive
SerializableArray // Array<Serializable>
SerializableObject // Partial<{ [key: string]: Serializable }>
SerializablePrimitive // null | boolean | number | string
Some // Function | boolean | bigint | number | string | symbol | object
Some<T> // Exclude<T, undefined | null>
// Make sure `T` is not `null` or `undefined`
type Option<T extends Some, E extends Error> = T | E
// Remove `null` or `undefined` from a type
type MaybeString = Optional<string> // string | null | undefined
type SomeString = Some<MaybeString> // string
Static<Predicate<T>> // T
type True = Static<typeof isTrue> // true
FAQs
A collection of composable JavaScript runtime type predicates with TypeScript type guard declarations
The npm package isntnt receives a total of 546 weekly downloads. As such, isntnt popularity was classified as not popular.
We found that isntnt demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.