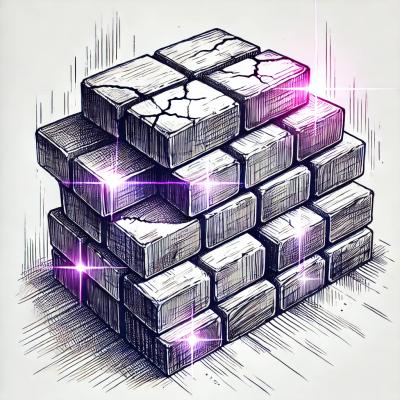
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
A collection of composable JavaScript runtime type predicates with TypeScript type guard declarations
Isntnt is a collection of composable JavaScript runtime type predicates with TypeScript type guard declarations. Supports generics including union and intersection types.
A predicate is a function that checks whether a value matches a certain type, it always returns a boolean. Well known examples of JavaScript predicates are Array.isArray
, isNan
, and Number.isNaN
. With isntnt
you add a whole slew of additional predicates to your toolbox, they are listed below.
Returns true
when you pass an object with a length property that is a valid length
.
hasLength(new ArrayBuffer()) // true
hasLength({}) // false
Always returns true
.
isAny(null) // true
isAny(12) // true
// always true
Returns true
when you pass an array.
isArray([]) // true
isArray('foo') // false
Returns true
when you pass a object that is not a function with a length property that is a valid length
.
isArrayLike({ length: 12 }) // true
isArrayLike(new Date()) // false
Returns true
when you pass a bigint.
isBigInt(116n) // true
isBigInt(12) // false
Returns true
when you pass a boolean.
isBoolean(true) // true
isBoolean(null) // false
Returns true
when you pass a Date object.
isDate(new Date()) // true
isDate(Date.now()) // false
Returns true
when you pass an object of which each property name, and each property value are a string.
isDictionary({ a: 'foo' })
isDictionary({ b: 12 })
Returns true
when you pass false
.
isFalse(false) // true
isFalse(true) // false
Returns true
when you pass a function.
isFunction(isFunction) // true
isFunction({}) // true
Returns true
when you pass an integer (a whole number).
isInt(1) // true
isInt(1.5) // false
Returns true
when you pass an integer ranging from -128 to 127.
isInt8(127) // true
isInt8(128) // false
Returns true
when you pass an integer ranging from -32,768 to 32,767.
isInt16(12) // true
isInt16(-50_000) // false
Returns true
when you pass an integer ranging from -2,147,483,648 to 2,147,483,647.
isInt32(12) // true
isInt32(Number.MAX_SAFE_INTEGER) // false
Alias to isUint32
.
Returns true
when you pas a Map object.
isMap(new Map()) // true
isMap({}) // false
Returns true
when you pas a negative number (including -0).
isNegative(-1) // true
isNegative(1) // false
Always returns false
.
isNever(null) // false
isNever(true) // false
// always returns false
Returns true
when you pass null
or undefined
.
isNone(null) // true
isNone(128) // false
Returns true
when you pass null
.
isNull(null) // true
isNull(undefined) // false
Returns true
when you pass a number that is not NaN
.
isNumber(12) // true
isNumber(NaN) // false
Returns true
when you pass an object that is not null
.
isObject({}) // true
isObject(null) // false
Returns true
when you pass an value that is not a boolean, null
, or undefined
.
isObjectLike([]) // true
isObjectLike(true) // false
Returns true
when you pass an object that was constructed by Object
.
isPlainObject({}) // true
isPlainObject(new Date()) // false
Returns true
when you pass a positive number.
isPositive(1) // true
isPositive(-1) // false
Returns true
when you pass null
, undefined
, a boolean, bigint, number, symbol, or string.
isPrimitive(1) // true
isPrimitive([]) // false
Returns true
when you pass a RegExp object.
isRegExp(/foo/) // true
isRegExp([]) // false
Returns true
when you pass a serializable primitive, a serializable array, or serializable object.
isSerializable(12) // true
isSerializable(console.log) // false
Returns true
when you pass an array where every element is serializable.
isSerializableArray(['foo', 1]) // true
isSerializableArray([new Date()]) // false
Returns true
when you pass a number that is not NaN
, Infinity
, or -Infinity
.
isSerializableNumber(1) // true
isSerializableNumber(Infinity) // false
Returns true
when you pass an object where every property is serializable.
isSerializableObject({ foo: 1 }) // true
isSerializableObject({ bar: Infinity }) // false
Returns true
when you pass null
, a serializable number, a boolean, bigint, symbol, or string.
isSerializablePrimitive(null) // true
isSerializablePrimitive(NaN) // false
Returns true
when you pas a Set object.
isSet(new Set()) // true
isSet([]) // false
Returns true
when you pas a value other than null
or undefined
.
isSome('foo') // true
isSome(null) // false
Returns true
when you pas a string.
isString('bar') // true
isString(48) // false
Returns true
when you pas a symbol.
isSymbol(Symbol()) // true
isSymbol('baz') // false
Returns true
when you pas true
.
isTrue(true) // true
isTrue(false) // false
Returns true
when you pass a positive integer.
isUint(112) // true
isUint(-16) // false
Returns true
when you pass an unsigned integer ranging from 0 to 255.
isUint8(255) // true
isUint8(1.2) // false
Returns true
when you pass an unsigned integer ranging from 0 to 65,535.
isUint16(2048) // true
isUint16(-12) // false
Returns true
when you pass an unsigned integer ranging from 0 to 4,294,967,295.
isUint32(256) // true
isUint(Number.MAX_SAFE_INTEGER) // false
Returns true
when you pass undefined
.
isUndefined(undefined) // true
isUndefined(null) // false
Returns true
when you pass a WeakMap
object.
isWeakMap(new WeakMap()) // true
isWeakMap(new Map()) // false
Predicate factories is where the real fun begins, they allow you to create and combine predicates of your own to create new predicates. By composing predicates you can describe objects, union types, number ranges, and so on.
Create a predicate based on a floor value, it checks if a number exceeds the floor value it’s given. This is an analogue to the >
operator.
const isAboveZero = above(0)
isAboveZero(-1) // false
isAboveZero(12) // true
The isAboveZero
predicate above returns true
when you pass a number that is greater than 0
.
Create a predicate based on several other predicates, it returns true
if every predicate that is passed also returns true
. This is an analogue to the &&
operator.
const adultAge = 18
const isMinorAge = and(isUint, below(adultAge))
isMinorAge(12) // true
isMinorAge(33) // false
The isMinorAge
predicate above returns true
when you pass an unsigned integer that is below 18.
const isUser = shape({ name: isString })
const hasEmailAddress = shape({ email: isString })
const isUserWithEmail = and(isUser, hasEmailAddress)
The isUserWithEmail
predicate above returns true
when you pass an object with a name
and an email
property, both of which are a string.
Create a predicate that checks every array element, it returns true
when its predicate returns true
for every element in an array.
const isNumberArray = array(isNumber)
The isNumberArray
predicate above returns true
when you pass an array of which every element is a number.
Create a predicate based on a property key and another predicate, it returns true
when its predicate returns true
for the value of an object at its given key.
const isStringAtName = at('name', isString)
isStringAtName({ name: 'Jane' }) // true
isStringAtName({ name: null }) // false
The isStringAtName
predicate above returns true
when you pass an object of which the name
property is a string.
Create a predicate based on a ceiling value, it checks if a number is below the ceiling value it’s given. This is an analogue to the <
operator.
const isBelow100 = below(100)
isBelow100(124) // false
isBelow100(32) // true
The isBelow100
predicate above returns true
when you pass a number that is less than 100
.
Create a predicate based on several literal values, it returns true
if a value matches either of the primitive values it’s given.
const isAOrB = either('a', 'b')
isAOrB('a') // true
isAOrB('c') // false
The isAOrB
predicate above returns true
when you pass either a
, or b
.
Create a predicate based on a property key, it returns true
if an object has a property of the key it’s given.
const hasName = has('name')
hasName({ name: null }) // true
hasName({ age: 36 }) // false
The hasName
predicate above returns true
when you pass an object that has a name
property.
Create a predicate based on a constructor, it returns true
if an object is an instance of the constructor key it’s given.
const isInstanceofString = instance(String)
isInstanceofString(new String('foo')) // true
isInstanceofString('foo') // false
The isInstanceofString
predicate above returns true
when you pass a String object.
Create a predicate based a primitive value, it returns true
if a value equals the primitive value it’s given.
const is42 = literal(42)
is42(42) // true
is42(43) // false
The is42
predicate above returns true
when you pass 42
.
Create a predicate based on a max. value, it checks if a number is below or equal to the max. value it’s given. This is an analogue to the <=
operator.
const isMax100 = max(100)
isMax100(100) // true
isMax100(101) // false
The isMax100
predicate above returns true
when you pass a number that is below or equal to 100
.
Create a predicate based on another predicate, it checks if a value matches it’s given predicate, or equals null
or undefined
.
const isMaybeString = maybe(isString)
isMaybeString(null) // true
isMaybeString(12) // false
The isMaybeString
predicate above returns true
when you pass a string, null
, or undefined
.
Create a predicate based on a min. value, it checks if a number is above or equal to the min. value it’s given. This is an analogue to the >=
operator.
const isMin100 = min(100)
isMin100(100) // true
isMin100(99) // false
The isMin0
predicate above returns true
when you pass a number that is above or equal to 100
.
Aliases maybe
.
Create a predicate based on another predicate, it checks if a value matches it’s given predicate, or equals null
.
const isNullableString = nullable(isString)
isNullableString(null) // true
isNullableString(12) // false
The isNullableString
predicate above returns true
when you pass a string or null
.
Create a predicate that checks every object property, it returns true
when its predicate returns true
for every object value.
const isEnumerable = object(isUint)
The isEnumerable
predicate above returns true
when you pass an object of which every element is an unsigned integer.
<T>(predicate: Predicate<T>) => (value: unknown) => value is T | undefined
const isOptionalString = optional(isString)
isOptionalString(undefined) // true
isOptionalString(12) // false
The isOptionalString
predicate above returns true
when you pass a string or undefined
.
Create a predicate based on several other predicates, it returns true
if some predicate that is passed also returns true
. This is an analogue to the ||
operator.
const isContent = or(isString, isSerializableNumber)
isContent(12) // true
isContent(null) // false
The isContent
predicate above returns true
when you pass a string or a primitive number.
const isStringOrNumber = or(isString, isNumber)
isStringOrNumber(8) // true
isStringOrNumber([]) // false
Create a predicate based on a key predicate and a value predicate, it returns true
if each property key of an object returns true
for its key predicate, and each value returns true
for its value predicate.
const isValidKey = either('a', 'b')
const isKeyValueMap = record(isValidKey, isInt)
isKeyValueMap({ a: 2 }) // true
isKeyValueMap({ a: null }) // false
isKeyValueMap({ c: 2 }) // false
The isKeyValueMap
predicate above returns true
when you pass an object where each key is either a
or b
, and each property value is an integer.
Create a predicate based on a predicate object, it returns true
if each property value of an object returns true
for its corresponding predicate.
const isPoint = shape({ x: isNumber, y: isNumber })
isPoint({ x: 12, y: 36 }) // true
isPoint({ x: 12 }) // false
The isPoint
predicate above returns true
when you an pass an object where both x
and y
properties are a number.
const isSlug = test(/^[A-Z0-9-]+$/i)
isSlug('foo-bar') // true
isSlug('!#$') // false
The isSlug
predicate above returns true
when you an pass a string that matches /^[A-Z0-9-]+$/i
.
Create a predicate based on index-based predicates, it returns true
if the element at each index of an array with a fixed length returns true
for its corresponding predicate.
const isLatitude = and(min(-90), max(90))
const isLongitude = and(min(-180), max(180))
const isLatLong = tuple(isLatitude, isLongitude)
isLatLong(45, -120) // true
isLatLong(120, 200) // false
The isLatLong
predicate above returns true
when you an pass an array with a length of 2 where index 0 is a latitude, and index 1 is a longitude value.
type User = Intersect<{ name: string } | { email: string }> // { name: string } & { email: string }
Unions a type with null
and undefined
.
type MaybeString = Maybe<string> // string | null | undefined
Union of null
and undefined
.
type X = None // null | undefined
Unions a type with null
.
type NullableString = Nullable<string> // string | null
Unions a type with undefined
.
type OptionalString = Optional<string> // string | undefined
Describes a predicate function signature
type IsString = Predicate<string> // <U>(value: U): value is Extract<U, string>
Union of all primitive types.
type X = Primitive // null | undefined | boolean | number | string | symbol | bigint
type X = Serializable // SerializableArray | SerializableObject | SerializableObject
type X = SerializableArray // Array<Serializable> | ReadonlyArray<Serializable>
type X = SerializableObject // { [key: string]: Serializable | undefined }
type X = SerializablePrimitive // null | boolean | number | string
Excludes null
and undefined
from a type, type parameter is optional.
type X = Some // Function | boolean | bigint | number | string | symbol | object
type Y = Some<string | null> // string
Extracts type parameter from Predicate type. This is useful to extract TypeScript types from (complex) predicates.
type X = Static<Predicate<string>>> // string
FAQs
A collection of composable JavaScript runtime type predicates with TypeScript type guard declarations
We found that isntnt demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.