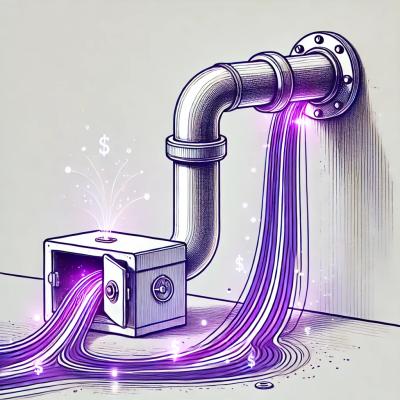
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
istanbul-cobertura-badger
Advanced tools
Creates a coverage badge by reading the Cobertura XML coverage report from node-istanbul reports using https://github.com/badges/shields.
I started to work with Node a few months ago, and I recently stumbled upon many different badges on GitHub pages, including code coverage. I posted a question on Stack Overflow about it:
http://stackoverflow.com/questions/26028024/how-to-make-a-gulp-code-coverage-badge
The idea is to serve in the Node.js README for internal use in a GitHub enterprise machine along with Jenkins.
npm install --save-dev istanbul-cobertura-badger
The following parameters are used:
You can generate the cobertura XML report by adding a new report to the istanbul command as follows:
--report cobertura
Take a look at this project's package.json
for details.
The option to change in the code is as follows:
// Setting the default coverage file generated by istanbul cobertura report.
opts.istanbulReportFile = opts.istanbulReportFile || "./coverage/cobertura-coverage.xml";
The destination directory will, by default, be the one from istanbul $APP/coverage
.
// The default location for the destination being the coverage directory from istanbul.
opts.destinationDir = opts.destinationDir || "./coverage/";
Note that the default directory will be used as coverage/cobertura-coverage.xml
.
The overall threshold is computed by using the line rate
, branch rate
and function rate
. That's the final value that defines
coverage result
. You can adjust the thresholds to properly generate the badges with appropriate colors.
// The thresholds to be used to give colors to the badge.
opts.thresholds = {
excellent: 90,
good: 65
};
Upon calling the callback function, the file cobertura.svg
will be available in the destination path.
var coberturaBadger = require('istanbul-cobertura-badger');
// Use the fixture that's without problems
var opts = {
//badgeFileName: "cobertura", // No extension, Defaults to "coverage"
destinationDir: __dirname, // REQUIRED PARAMETER!
istanbulReportFile: path.resolve(__dirname, "coverage", "cobertura-coverage.xml"),
thresholds: {
// overall percent >= excellent, green badge
excellent: 90,
// overall percent < excellent and >= good, yellow badge
good: 65
// overall percent < good, red badge
}
};
// Load the badge for the report$
badger(opts, function parsingResults(err, badgeStatus) {
if (err) {
console.log("An error occurred: " + err.message);
}
console.log("Badge successfully generated at " + badgeStatus.badgeFile.file);
console.log(badgeStatus);
});
An example of a successful badge creation is as follows:
{ overallPercent: 66,
functionRate: 0.7368421052631579,
lineRate: 0.8034,
branchRate: 0.47369999999999995,
url: 'http://img.shields.io/badge/coverage-66%-yellow.svg',
badgeFile: {
method: 'GET',
code: 200,
file: '/home/mdesales/dev/github/intuit/istanbul-cobertura-badger/test/coverage.svg'
}
}
gulp.task('test', function() {
gulp.src('src/**/*.js')
.pipe(istanbul()) // coverying files
.on('finish', function () {
gulp.src(['test/*.js'])
.pipe(mocha({reporter: 'spec'})) // different reporters at http://mochajs.org/#reporters
.pipe(istanbul.writeReports({
reporters: ['cobertura', 'text-summary', 'html'], // https://www.npmjs.org/package/gulp-istanbul#reporters
reportOpts: { dir: './docs/tests' }
}))
.on('end', function() {
var opts = {
destinationDir: path.resolve(__dirname, "docs", "tests"),
istanbulReportFile: path.resolve(__dirname, "docs", "tests", "cobertura-coverage.xml"),
}
coverageBadger(opts, function(err, results) {
if (err) {
console.log("An error occurred while generating the coverage badge" + err.message);
process.exit(-1);
}
console.log("Badge generated successfully: " + results)
process.exit(0);
});
});
});
});
You can now use the CLI to create the badge for ANY XML Cobertura report created from Istanbul or Java applications.
The CLI prints the following help:
$ istanbul-cobertura-badger
Usage: cli [options]
Generates a badge for a given Cobertura XML report
Options:
-h, --help output usage information
-V, --version output the version number
-f, --defaults Use the default values for all the input.
-e, --excellentThreshold <n> The threshold for green badges, where coverage >= -e
-g, --goodThreshold <n> The threshold for yellow badges, where -g <= coverage < -e
-b, --badgeFileName <badge> The badge file name that will be saved.
-r, --reportFile <report> The istanbul cobertura XML file path.
-d, --destinationDir <destination> The directory where 'coverage.svg' will be generated at.
-v, --verbose Prints the metadata for the command
Examples:
$ istanbul-cobertura-badger -e 90 -g 65 -r coverage/cobertura.xml -d coverage/
* Green: coverage >= 90
* Yellow: 65 <= coverage < 90
* Red: coverage < 65
* Created at the coverage directory from the given report.
$ istanbul-cobertura-badger -e 80 -d /tmp/build
* Green: coverage >= 80
* Yellow: 65 <= coverage < 80
* Red: coverage < 65
Run the CLI using the default values defined above. Here's the example of running against this project.
$ istanbul-cobertura-badger -f -v
{ overallPercent: 91,
functionRate: 1,
lineRate: 0.9309999999999999,
branchRate: 0.8167,
url: 'http://img.shields.io/badge/coverage-91%-brightgreen.svg',
badgeFile:
{ downloaded: true,
filePath: '/home/mdesales/dev/github/intuit/istanbul-cobertura-badger/coverage/coverage.svg',
size: 730 },
color: 'brightgreen' }
Badge created at /home/mdesales/dev/github/intuit/istanbul-cobertura-badger/coverage/coverage.svg
Just use the simple command with some options.
$ istanbul-cobertura-badger -e 85 -g 70 -r test/fixture/istanbul-report.xml -d /tmp/
Badge created at /tmp/coverage.svg
The overall information collected is also presented when using the verbose option.
$ istanbul-cobertura-badger -e 85 -g 70 -r test/fixture/istanbul-report.xml -d /tmp/ -v
{ overallPercent: 66,
functionRate: 0.7368421052631579,
lineRate: 0.8034,
branchRate: 0.47369999999999995,
url: 'http://img.shields.io/badge/coverage-66%-red.svg',
badgeFile: { downloaded: true, filePath: '/tmp/coverage.svg', size: 733 },
color: 'red' }
Badge created at /tmp/coverage.svg
You can print the current version of the project by using the -V option. It uses the package.json#version as the value.
$ istanbul-cobertura-badger -V
1.0.0
We use the GitFlow branching model http://nvie.com/posts/a-successful-git-branching-model/.
git checkout -b feature/issue-444-Add-Rest-APIs origin/master --track
)git commit -am 'Fix #444: Add support to REST-APIs'
)git push feature/issue-444-Add-Rest-APIS
)FAQs
Istanbul test coverage report badger task.
The npm package istanbul-cobertura-badger receives a total of 917 weekly downloads. As such, istanbul-cobertura-badger popularity was classified as not popular.
We found that istanbul-cobertura-badger demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.