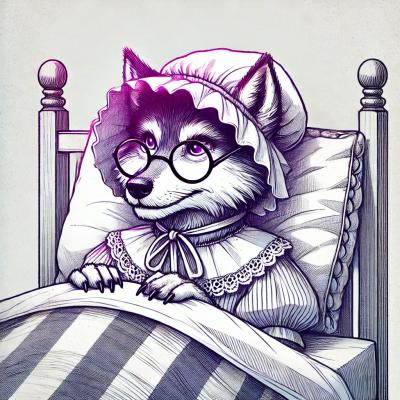
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
istanbul-lib-report
Advanced tools
The istanbul-lib-report package is a library for generating code coverage reports in various formats. It is part of the Istanbul code coverage tooling ecosystem and provides a way to create detailed and summary coverage reports based on the coverage data collected during test execution. This package is typically used in conjunction with other Istanbul libraries to collect and process coverage information.
Creating a coverage report
This code demonstrates how to generate an HTML coverage report using istanbul-lib-report. It involves creating a report context with the output directory and coverage map, then executing the report generation with the desired format ('html' in this case).
const libReport = require('istanbul-lib-report');
const reports = require('istanbul-reports');
let context = libReport.createContext({
dir: './coverage', // Output directory
watermarks: libReport.getDefaultWatermarks(),
coverageMap: coverageMap // Assume coverageMap is previously defined
});
let report = reports.create('html', {
projectRoot: './'
});
report.execute(context);
Configuring watermarks for coverage thresholds
This example shows how to configure watermarks for coverage thresholds in a report. Watermarks define the color coding of coverage results (e.g., red for low coverage, yellow for medium, green for high). The thresholds are set for statements, functions, branches, and lines.
const libReport = require('istanbul-lib-report');
let context = libReport.createContext({
dir: './coverage',
watermarks: {
statements: [50, 80],
functions: [50, 80],
branches: [50, 80],
lines: [50, 80]
},
coverageMap: coverageMap
});
nyc is a command line interface and a higher-level wrapper around Istanbul, offering a simpler way to collect and report code coverage. It integrates with Istanbul libraries but provides additional features like process spawning and support for ES2015. Compared to istanbul-lib-report, nyc is more of a complete toolset rather than a library focused on reporting.
Coveralls is a web service to help you track your code coverage over time, and ensure that all your new code is fully covered. While not a direct alternative to istanbul-lib-report, it can be used in conjunction with Istanbul to provide detailed coverage reports and history in a CI/CD environment. It focuses more on the integration and visualization of coverage data rather than the generation of reports.
Codecov is another web service similar to Coveralls, offering features to track code coverage, see how it changes over time, and ensure that pull requests do not reduce coverage. It integrates with CI tools and version control systems to provide detailed reports and analytics. Like Coveralls, Codecov is complementary to istanbul-lib-report, focusing on the aggregation and visualization of coverage data.
Core reporting utilities for istanbul.
const libReport = require('istanbul-lib-report');
const reports = require('istanbul-reports');
// coverageMap, for instance, obtained from istanbul-lib-coverage
const coverageMap;
const configWatermarks = {
statements: [50, 80],
functions: [50, 80],
branches: [50, 80],
lines: [50, 80]
};
// create a context for report generation
const context = libReport.createContext({
dir: 'report/output/dir',
// The summarizer to default to (may be overridden by some reports)
// values can be nested/flat/pkg. Defaults to 'pkg'
defaultSummarizer: 'nested',
watermarks: configWatermarks,
coverageMap,
})
// create an instance of the relevant report class, passing the
// report name e.g. json/html/html-spa/text
const report = reports.create('json', {
skipEmpty: configSkipEmpty,
skipFull: configSkipFull
})
// call execute to synchronously create and write the report to disk
report.execute(context)
FAQs
Base reporting library for istanbul
The npm package istanbul-lib-report receives a total of 4,687,641 weekly downloads. As such, istanbul-lib-report popularity was classified as popular.
We found that istanbul-lib-report demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.