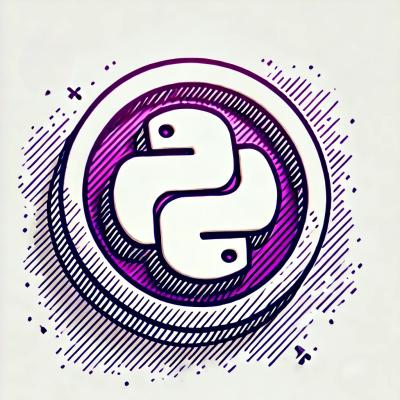
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
iterable-operator
Advanced tools
Utility for JavaScript Iterable and AsyncIterable.
npm install --save iterable-operator
# or
yarn add iterable-operator
import { map, toArray } from 'iterable-operator'
const iter = [1, 2, 3]
const doubleIter = map(iter, x => x * 2)
const result = toArray(doubleIter)
import { IterableOperator } from 'iterable-operator/lib/es2018/style/chaining'
const result = new IterableOperator([1, 2, 3])
.map(x => x * 2)
.toArray()
It is prepared for bind-operator.
import { map, toArray } from 'iterable-operator/lib/es2018/style/binding'
const result = [1, 2, 3]
::map(x => x * 2)
::toArray()
It is prepared for pipeline-operator.
import { map, toArray } from 'iterable-operator/lib/es2018/style/pipeline'
const result = [1, 2, 3]
|> map(x => x * 2)
|> toArray()
There are three types of operators:
function countdown(begin: number, end: number): Iterable<number>
countdown(2, -2) // [2, 1, 0, -1, -2]
countdown(1, 1) // [1]
countdown(0, 1) // []
function countup(begin: number, end: number): Iterable<number>
countup(-2, 2) // [-2, -1, 0, 1, 2]
countup(1, 1) // [1]
countup(1, 0) // []
function range(start: number, end: number, step: number = 1): Iterable<number>
// step > 0
range(1, 1) // []
range(-2, 2) // [-2, -1, 0, 1]
range(2, -2) // [2, 1, 0, -1]
range(1, -1, 0.5) // [1, 0.5, 0, -0.5]
range(2, -2, 0) // throw InvalidArgumentError
range(2, -2, -0.5) // throw InvalidArgumentError
function chunk<T>(iterable: Iterable<T>, size: number): Iterable<T[]>
function chunkAsync<T>(iterable: AsyncIterable<T>, size: number): AsyncIterable<T[]>
// size > 0
chunk([1, 2, 3], 2) // [[1, 2], [3]]
chunk([1, 2, 3], 3) // [[1, 2, 3]]
chunk([1, 2, 3], 5) // [[1, 2, 3]]
chunk([1, 2, 3], 0) // throw InvalidArgumentError
chunk([1, 2, 3], -1) // throw InvalidArgumentError
function chunkBy<T>(iterable: Iterable<T>, fn: (element: T, index: number) => boolean): Iterable<T[]>
function chunkByAsync<T>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => boolean | PromiseLike<boolean>): AsyncIterable<T[]>
chunkBy([1, 2, 3], x => x === 2) // [[1, 2], [3]]
chunkBy([1, 2, 3], x => x === 3) // [[1, 2, 3]]
chunkBy([1, 2, 3], x => x === 5) // [[1, 2, 3]]
function concat<TResult>(...iterables: Iterable<unknown>[]): Iterable<TResult>
function concatAsync<TResult>(...iterables: Array<Iterable<unknown> | AsyncIterable<unknown>>): AsyncIterable<TResult>
concat([1, 2, 3]) // throw InvalidArgumentsLengthError
concat([1, 2, 3], ['a', 'b', 'c']) // [1, 2, 3, 'a', 'b', 'c']
function drop<T>(iterable: Iterable<T>, count: number): Iterable<T>
function dropAsync<T>(iterable: AsyncIterable<T>, count: number): AsyncIterable<T>
// count >= 0
drop([1, 2, 3], 0) // [1, 2, 3]
drop([1, 2, 3], 2) // [3]
drop([1, 2, 3], 3) // []
drop([1, 2, 3], 5) // []
drop([1, 2, 3], -1) // throw InvalidArgumentError
function dropRight<T>(iterable: Iterable<T>, count: number): Iterable<T>
function dropRightAsync<T>(iterable: AsyncIterable<T>, count: number): AsyncIterable<T>
// count >= 0
dropRight([1, 2, 3], 0) // [1, 2, 3]
dropRight([1, 2, 3], 2) // [1]
dropRight([1, 2, 3], 3) // []
dropRight([1, 2, 3], 5) // []
dropRight([1, 2, 3], -1) // throw InvalidArgumentError
function dropUntil<T>(iterable: Iterable<T>, fn: (element: T, index: number) => boolean): Iterable<T>
function dropUntilAsync<T>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => boolean | PromiseLike<boolean>): AsyncIterable<T>
dropUntil([1, 2, 3], x => x === 2) // [2, 3]
function filter<T, U extends T = T>(iterable: Iterable<T>, fn: (element: T, index: number) => boolean): Iterable<U>
function filterAsync<T, U extends T = T>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => boolean | PromiseLike<boolean>): AsyncIterable<U>
filter([1, 2, 3], x => x % 2 === 1) // [1, 3]
function flatten<T>(iterable: Iterable<unknown>): Iterable<T>
function flattenAsync<T>(iterable: AsyncIterable<unknown>): AsyncIterable<T>
flatten([]) // []
flatten('123') // ['1', '2', '3']
flatten(['one', ['two'], 0, [1, [2]]]) // ['o', 'n', 'e', 'two', 0, 1, [2]]
function flattenDeep<T>(iterable: Iterable<unknown>, depth: number = Infinity): Iterable<T>
function flattenDeepAsync<T>(iterable: AsyncIterable<unknown>, depth: number = Infinity): AsyncIterable<T>
// depth >= 0
flattenDeep([]) // []
flattenDeep('123') // ['1', '2', '3']
flattenDeep([], -1) // throw InvalidArgumentError
flattenDeep([0, [1]], 0) // [0, [1]]
flattenDeep(['one', ['two', ['three']], 0, [1, [2, [3]]]], 2) // ['o', 'n', 'e', 't', 'w', 'o', 'three', 0, 1, 2, [3]]
function flattenBy(iterable: Iterable<unknown>, fn: (element: unknown, level: number) => boolean): Iterable<any>
function flattenByAsync(iterable: Iterable<unknown> | AsyncIterable<unknown>, fn: (element: unknown, level: number) => boolean | PromiseLike<unknown>): AsyncIterable<any>
flattenBy(['one', ['two'], 0, [1]], x => typeof x !== 'string') // ['one', 'two', 0, 1]
flattenBy([], () => true) // []
flattenBy('123', () => true) // ['1', '2', '3']
function map<T, U>(iterable: Iterable<T>, fn: (element: T, index: number) => U): Iterable<U>
function mapAsync<T, U>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => U | PromiseLike<U>): AsyncIterable<U>
map([1, 2, 3], x => x * 2) // [2, 4, 6]
function repeat<T>(iterable: Iterable<T>, times: number): Iterable<T>
function repeatAsync<T>(iterable: AsyncIterable<T>, times: number): AsyncIterable<T>
// times >= 0
repeat([1, 2, 3], 2) // [1, 2, 3, 1, 2, 3]
repeat([1, 2, 3], 0) // []
repeat([1, 2, 3], -1) // throw InvalidArgumentError
function slice<T>(iterable: Iterable<T>, start: number, end: number = Infinity): Iterable<T>
function sliceAsync<T>(iterable: AsyncIterable<T>, start: number, end: number = Infinity): AsyncIterable<T>
// start >= 0, end >= start
slice([1, 2, 3], -1, 1) // throw InvalidArgumentError
slice([1, 2, 3], 3, 5) // []
slice([1, 2, 3], 1, 2) // [2]
slice([1, 2, 3], 1, 1) // []
slice([1, 2, 3], 2, 1) // throw InvalidArgumentError
function split<T>(iterable: Iterable<T>, separator: T): Iterable<T[]>
function splitAsync<T>(iterable: AsyncIterable<T>, separator: T): AsyncIterable<T[]>
split([1, 2, 3, 4, 5], 3) // [[1, 2], [4, 5]]
split([1, 2, 3, 4, 5], 1) // [[], [2, 3, 4, 5]]
split([1, 2, 3, 4, 5], 5) // [[1, 2, 3, 4], []]
split([1, 2, 3, 4, 5], 0) // [[1, 2, 3, 4, 5]]
function splitBy<T>(iterable: Iterable<T>, fn: (element: T, index: number) => boolean): Iterable<T[]>
function splitByAsync<T>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => boolean | PromiseLike<boolean>): AsyncIterable<T[]> {
splitBy([1, 2, 3, 4, 5], x => x === 3) // [[1, 2], [4, 5]]
splitBy([1, 2, 3, 4, 5], x => x === 1) // [[], [2, 3, 4, 5]]
splitBy([1, 2, 3, 4, 5], x => x === 5) // [[1, 2, 3, 4], []]
splitBy([1, 2, 3, 4, 5], x => x === 0) // [[1, 2, 3, 4, 5]]
function take<T>(iterable: Iterable<T>, count: number): Iterable<T>
function takeAsync<T>(iterable: AsyncIterable<T>, count: number): AsyncIterable<T>
take([1, 2, 3], 5) // [1, 2, 3]
take([1, 2, 3], 2) // [1, 2]
take([1, 2, 3], 0) // []
take([1, 2, 3], -1) // throw InvalidArgumentError
function takeRight<T>(iterable: Iterable<T>, count: number): Iterable<T>
function takeRightAsync<T>(iterable: AsyncIterable<T>, count: number): AsyncIterable<T>
takeRight([1, 2, 3], 2) // [2, 3]
takeRight([1, 2, 3], 5) // [1, 2, 3]
takeRight([1, 2, 3], 0) // []
takeRight([1, 2, 3], -1) // throw InvalidArgumentError
function takeUntil<T>(iterable: Iterable<T>, fn: (element: T, index: number) => boolean): Iterable<T>
function takeUntilAsync<T>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => boolean | PromiseLike<T>): AsyncIterable<T>
takeUntil([1, 2, 3], x => x === 2) // [1]
function tap<T>(iterable: Iterable<T>, fn: (element: T, index: number) => unknown): Iterable<T> {
function tapAsync<T>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => unknown | PromiseLike<unknown>): AsyncIterable<T>
tap([1, 2, 3], x => console.log(x)) // [1, 2, 3]
function toAsyncIterable<T>(iterable: Iterable<T | PromiseLike<T>>): AsyncIterable<T>
toAsyncIterable([1, 2, 3]) // AsyncIterable [1, 2, 3]
function transform<T, U>(iterable: Iterable<T>, transformer: (iterable: Iterable<T>) => Iterable<U>): Iterable<U>
function transformAsync<T, U>(iterable: Iterable<T>, transformer: (iterable: Iterable<T>) => AsyncIterable<U>): AsyncIterable<U>
function transformAsync<T, U>(iterable: AsyncIterable<T>, transformer: (iterable: AsyncIterable<T>) => AsyncIterable<U>): AsyncIterable<U>
transform([1, 2, 3], function* double(iter) {
for (const element of iter) {
yield element * 2
}
}) // [2, 4, 6]
function uniq<T>(iterable: Iterable<T>): Iterable<T>
function uniqAsync<T>(iterable: AsyncIterable<T>): AsyncIterable<T>
uniq([1, 1, 2, 2, 3, 3]) // [1, 2, 3]
function uniqBy<T, U>(iterable: Iterable<T>, fn: (element: T, index: number) => U): Iterable<T> {
function uniqByAsync<T, U>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => U | PromiseLike<U>): AsyncIterable<T>
uniqBy([1, 2, 3], x => x % 2) // [1, 2]
function zip<TResult>(...iterables: Iterable<unknown>[]): Iterable<TResult[]>
function zipAsync<TResult>(...iterables: Array<Iterable<unknown> | AsyncIterable<unknown>>): AsyncIterable<TResult[]>
zip([1, 2, 3], ['a', 'b', 'c']) // [[1, 'a'], [2, 'b'], [3, 'c']]
zip([1, 2, 3], ['a', 'b']) // [[1, 'a'], [2, 'b']]
function consume<T, U>(iterable: Iterable<T>, consumer: (iterable: Iterable<T>) => U): U
function consume<T, U>(iterable: AsyncIterable<T>, consumer: (iterable: AsyncIterable<T>) => U): U
consume([1, 2, 3], xs => new Set(xs)) // Set [1, 2, 3]
function each<T>(iterable: Iterable<T>, fn: (element: T, index: number) => unknown): void
function eachAsync<T>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => unknown | PromiseLike<unknown>): Promise<void>
each([1, 2, 3], x => console.log(x)) // void
function every<T>(iterable: Iterable<T>, fn: (element: T, index: number) => boolean): boolean
function everyAsync<T>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => boolean | PromiseLike<boolean>): Promise<boolean>
every([1, 2, 3], x => x < 5) // true
every([1, 2, 3], x => x <= 2) // false
function find<T>(iterable: Iterable<T>, fn: (element: T, index: number) => boolean): T
function findAsync<T>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => boolean | PromiseLike<boolean>): Promise<T>
find([1, 2, 3], x => x === 2) // 2
find([1, 2, 3], x => x === 4) // throw RuntimeError
function first<T>(iterable: Iterable<T>): T
function firstAsync<T>(iterable: AsyncIterable<T>): Promise<T>
first([1, 2, 3]) // 1
first([]) // throw RuntimeError
function includes<T>(iterable: Iterable<T>, value: T): boolean
function includesAsync<T>(iterable: AsyncIterable<T>, value: T): Promise<boolean>
includes([1, 2, 3], 2) // true
includes([1, 2, 3], 4) // false
function last<T>(iterable: Iterable<T>): T
function lastAsync<T>(iterable: AsyncIterable<T>): Promise<T>
last([1, 2, 3]) // 3
last([]) // throw RuntimeError
function match<T>(iterable: Iterable<T>, sequence: ArrayLike<T>): boolean
function matchAsync<T>(iterable: AsyncIterable<T>, sequence: ArrayLike<T>): Promise<boolean>
match([1, 2, 3], [2, 3]) // true
match([1, 2, 3], [3, 2]) // false
match([1, 2, 3], []) // true
function reduce<T, U>(
iterable: Iterable<T>
, fn:
((accumulator: T, currentValue: T, index: number) => T)
& ((accumulator: U, currentValue: T, index: number) => U)
, initialValue?: U
)
function reduceAsync<T, U>(
iterable: Iterable<T> | AsyncIterable<T>
, fn:
((accumulator: T, currentValue: T, index: number) => T | PromiseLike<T>)
& ((accumulator: U, currentValue: T, index: number) => U | PromiseLike<U>)
, initialValue?: U
)
reduce([], (acc, cur) => acc + cur) // throw RuntimeError
reduce([1], (acc, cur) => acc + cur) // 1
reduce([1, 2, 3], (acc, cur) => acc + cur) // 6
reduce([1, 2, 3], (acc, cur, index) => {
acc.push([cur, index])
return acc
}) // [[1, 0], [2, 1], [3, 2]]
function some<T>(iterable: Iterable<T>, fn: (element: T, index: number) => boolean): boolean
function someAsync<T>(iterable: Iterable<T> | AsyncIterable<T>, fn: (element: T, index: number) => boolean | PromiseLike<boolean>): Promise<boolean>
some([1, 2, 3], x => x === 2) // true
some([1, 2, 3], x => x === 4) // false
function toArray<T>(iterable: Iterable<T>): T[]
function toArrayAsync<T>(iterable: AsyncIterable<T>): Promise<T[]>
toArray([1, 2, 3]) // Array [1, 2, 3]
function toSet<T>(iterable: Iterable<T>): Set<T>
function toSetAsync<T>(iterable: AsyncIterable<T>): Promise<Set<T>>
toSet([1, 1, 2, 2, 3, 3]) // Set [1, 2, 3]
FAQs
Utilities for JavaScript Iterable and AsyncIterable
The npm package iterable-operator receives a total of 6,411 weekly downloads. As such, iterable-operator popularity was classified as popular.
We found that iterable-operator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.