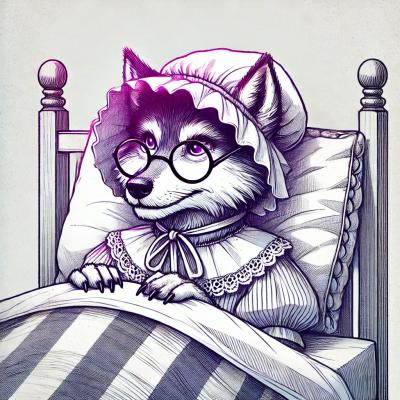
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The iterare npm package provides a set of utilities for working with iterables in JavaScript. It allows you to create, transform, and consume iterables in a functional programming style.
Creating Iterables
You can create an iterable from an array or any other iterable object using the `iterate` function.
const { iterate } = require('iterare');
const iterable = iterate([1, 2, 3, 4]);
console.log([...iterable]); // Output: [1, 2, 3, 4]
Transforming Iterables
You can transform iterables using methods like `map` and `filter` to apply functions to each element or filter elements based on a condition.
const { iterate } = require('iterare');
const iterable = iterate([1, 2, 3, 4])
.map(x => x * 2)
.filter(x => x > 4);
console.log([...iterable]); // Output: [6, 8]
Consuming Iterables
You can consume iterables using methods like `reduce` to aggregate values or perform other operations that consume the iterable.
const { iterate } = require('iterare');
const iterable = iterate([1, 2, 3, 4]);
const sum = iterable.reduce((acc, val) => acc + val, 0);
console.log(sum); // Output: 10
Lodash is a popular utility library that provides a wide range of functions for working with arrays, objects, and other data types. It includes methods for creating, transforming, and consuming collections, similar to iterare, but with a broader scope and more features.
Ramda is a functional programming library for JavaScript that emphasizes immutability and side-effect-free functions. It provides utilities for working with lists and other data structures in a functional style, similar to iterare, but with a focus on functional programming principles.
RxJS is a library for reactive programming using Observables, which can be thought of as a more powerful and flexible form of iterables. It provides a wide range of operators for transforming and consuming data streams, similar to iterare, but with a focus on reactive programming.
iterare
lat. to repeat, to iterate
ES6 Iterator library for applying multiple transformations to a collection in a single iteration.
Ever wanted to iterate over ES6 collections like Map
or Set
with Array
-built-ins like map()
, filter()
, reduce()
?
Lets say you have a large Set
of URIs and want to get a Set
back that contains file paths from all file://
URIs.
The loop solution is very clumsy and not very functional:
const uris = new Set([
'file:///foo.txt',
'http:///npmjs.com',
'file:///bar/baz.txt'
])
const paths = new Set()
for (const uri of uris) {
if (!uri.startsWith('file://')) {
continue
}
const path = uri.substr('file:///'.length)
paths.add(path)
}
Much more readable is converting the Set
to an array, using its methods and then converting back:
new Set(
Array.from(uris)
.filter(uri => uri.startsWith('file://'))
.map(uri => uri.substr('file:///'.length))
)
But there is a problem: Instead of iterating once, you iterate 4 times (one time for converting, one time for filtering, one time for mapping, one time for converting back). For a large Set with thousands of elements, this has significant overhead.
Other libraries like RxJS or plain NodeJS streams would support these kinds of "pipelines" without multiple iterations, but they work only asynchronously.
With this library you can use many methods you know and love from Array
and lodash while only iterating once - thanks to the ES6 iterator protocol:
import iterate from 'iterare'
iterate(uris)
.filter(uri => uri.startsWith('file://'))
.map(uri => uri.substr('file:///'.length))
.toSet()
iterate
accepts any kind of Iterator or Iterable (arrays, collections, generators, ...) and returns a new Iterator object that can be passed to any Iterable-accepting function (collection constructors, Array.from()
, for of
, ...).
Only when you call a method like toSet()
, reduce()
or pass it to a for of
loop will each value get pulled through the pipeline, and only once.
This library is essentially
Benchmark based on the example above:
Method | ops/sec |
---|---|
Loop | 2,562,637 ops/sec ±3.95% (80 runs sampled) |
iterare | 2,023,212 ops/sec ±1.38% (84 runs sampled) |
Array method chain | 346,117 ops/sec ±2.68% (82 runs sampled) |
Lodash (with lazy evalution) | 335,890 ops/sec ±0.55% (85 runs sampled) |
RxJS | 29,480 ops/sec ±7.01% (51 runs sampled) |
The source is written in TypeScript.
npm run build
compiles TSnpm run watch
compiles on file changesnpm test
runs testsnpm run benchmark
runs the benchmarkFAQs
Array methods for ES6 Iterators
The npm package iterare receives a total of 2,509,725 weekly downloads. As such, iterare popularity was classified as popular.
We found that iterare demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.