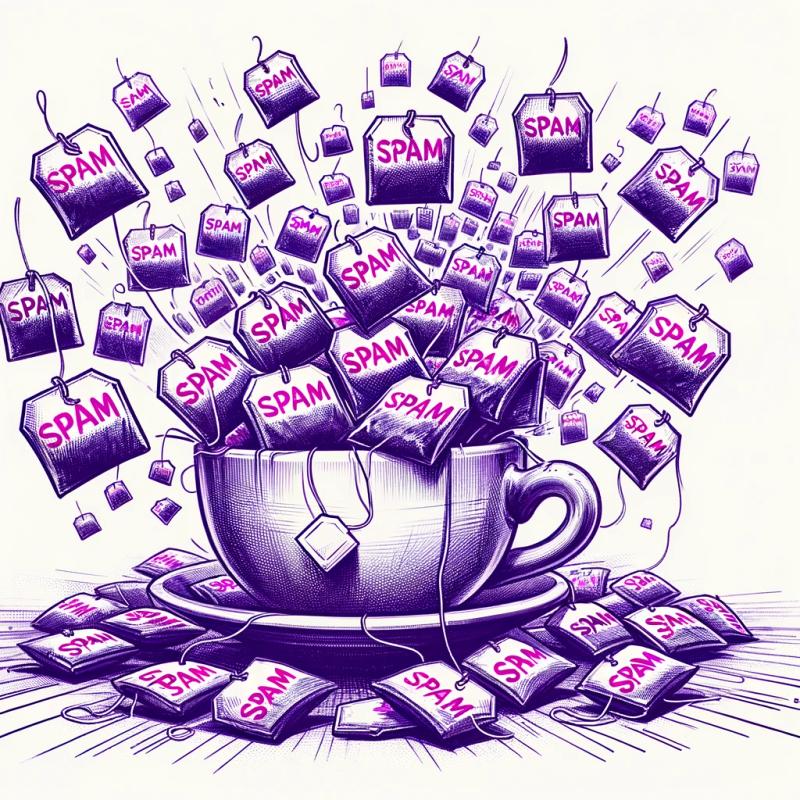
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
jacuzzi
Advanced tools
Readme
A generic resource pool and balancer.
Inspired by @coopernurse's node-pool but with an additional balancer layer and Promises (API supports both callbacks and Promises).
Caution: This project is in early stages. It'll be battle tested in next couple of weeks. If you run into misbehavior, try setting DEBUG=jacuzzi:*
. Feedback of any kind is welcome.
A Generic Resource Pool.
var Pool = require('jacuzzi').Pool
Instantiates a new Pool. If given, the name
is used for debugging purposes. The args
provided after the opts
argument are forwarded to the opts.create
function.
Options:
Promise
or use the callback
argument)Promise
or use the callback
argument for asynchronous destruction)true
if the resource is OK, or otherwise false
; for asynchronous checks it could also return a Promise
or use the callback
argument)This method is used to acquire / request a resource from the pool. It returns a Promise
. As soon as there is a resource available, the Promise
gets resolved and (if provided) the callback
called.
This method is used to release / return a resource
back to the pool.
Gracefully shut down the pool.
Keep in mind that jacuzzi
is generic, i.e., resources are neither limited to sockets nor to connections!
var net = require('net')
var Pool = require('jacuzzi').Pool
var pool = new Pool({
create: function(port, callback) {
var socket = net.connect(4000, function() {
callback(socket)
})
socket.setEncoding('utf8')
socket.setTimeout(300000)
},
destroy: function(socket, callback) {
if (!socket.localPort) callback()
else socket.end(callback)
},
check: function(socket) {
return !!socket.localPort
}
})
pool.acquire(function(err, socket) {
pool.release(socket)
})
A resource pool balancer.
var Balancer = require('jacuzzi').Balancer
Instantiates a new Balancer.
Options:
true
if the pool is OK, or otherwise false
; for asynchronous checks it could also return a Promise
or use the callback
argument)This method is used to add a pool to the balancer. The priority
argument affects the scheduling. The lowest number for priority
indicates the highest priority. Pools with lower priority are only selected, if the higher ones are down. Multiple pools with the same priority a scheduled with first come first serve.
Example:
balancer.add(a, 1)
balancer.add(b, 2)
balancer.add(c, 3)
balancer.acquire() // ~> a
balancer.acquire() // ~> a
balancer.acquire() // ~> a
...
// a goes down
balancer.acquire() // ~> b
balancer.acquire() // ~> c
balancer.acquire() // ~> b
balancer.acquire() // ~> c
...
This method is used to acquire / request a resource from the balancer. It returns a Promise
. As soon as there is a resource available, the Promise
gets resolved and (if provided) the callback
called.
This method is used to release / return a resource
back to its pool.
Drain all pools.
Keep in mind that jacuzzi
is generic, i.e., resources are neither limited to sockets nor to connections!
var net = require('net'), a, b, c
var opts = {
create: function(port, callback) {
var socket = net.connect(port, function() {
callback(socket)
})
socket.setEncoding('utf8')
socket.setTimeout(300000)
},
destroy: function(socket, callback) {
if (!socket.localPort) callback()
else socket.end(callback)
},
check: function(socket) {
return !!socket.localPort
}
}
var Balancer = require('jacuzzi').Pool
var balancer = new Balancer({
check: function(pool) {
// e.g. ping
// pool.opts.args = [4001] (or 4002, 4003 respectively)
}
})
balancer.add(a = new Pool('Pool A', opts, 4001), 1)
balancer.add(b = new Pool('Pool B', opts, 4002), 2)
balancer.add(c = new Pool('Pool C', opts, 4003), 2)
balancer.acquire(function(err, socket) {
balancer.release(socket)
})
Debug message can be turned on with the env DEBUG=jacuzzi:*
.
Copyright (c) 2014-2015 Markus Ast
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
A generic resource pool and balancer.
The npm package jacuzzi receives a total of 0 weekly downloads. As such, jacuzzi popularity was classified as not popular.
We found that jacuzzi demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.